How do I use isolated?
Asked on 08/02/2024
1 search
To use isolated
in Swift, you need to understand how Swift concurrency handles isolation and guarantees. Here are some key points from the session "Migrate your app to Swift 6" at WWDC 2024:
-
Non-Isolated Methods:
- If a method is part of a type that is isolated to an actor (e.g., the main actor), but the method itself does not need to be isolated, you can declare it as
nonisolated
. This means the method can be called from any context without requiring the actor's isolation. - Example:
nonisolated func someMethod() { // This method is not isolated to the actor }
- Migrate your app to Swift 6 (17:12)
- If a method is part of a type that is isolated to an actor (e.g., the main actor), but the method itself does not need to be isolated, you can declare it as
-
Assume Isolated:
- When you know a function will always be called on a specific actor, you can use
assume isolated
to inform the compiler. This avoids starting a new task and simply tells Swift that the code is already running on the specified actor. - Example:
@MainActor assume isolated func someMainActorMethod() { // This method assumes it's being called on the main actor }
- Migrate your app to Swift 6 (24:15)
- When you know a function will always be called on a specific actor, you can use
-
Non-Isolated Unsafe:
- In cases where you need to bypass Swift's concurrency safety checks, you can use
nonisolated unsafe
. This should be a last resort and used with caution, as it places the burden of ensuring safety on you. - Example:
nonisolated unsafe var someUnsafeVariable: SomeType
- Migrate your app to Swift 6 (14:56)
- In cases where you need to bypass Swift's concurrency safety checks, you can use
-
Concurrency and Delegate Callbacks:
- When dealing with delegate callbacks, you can annotate the delegate protocol or callback to specify that it will always be called on a particular actor, such as the main actor.
- Example:
protocol SomeDelegate: AnyObject { @MainActor func someCallback() }
- Migrate your app to Swift 6 (21:33)
These techniques help you manage concurrency and data isolation in Swift, ensuring that your code is safe and free from data races. For more detailed information, you can refer to the session "Migrate your app to Swift 6" from WWDC 2024.
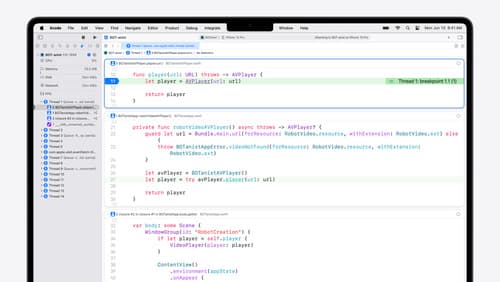
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
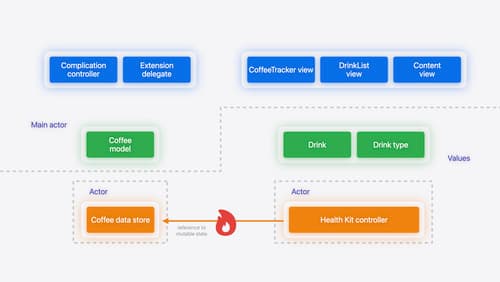
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.