can you teach me swift concurrency
Generated on 8/2/2024
1 search
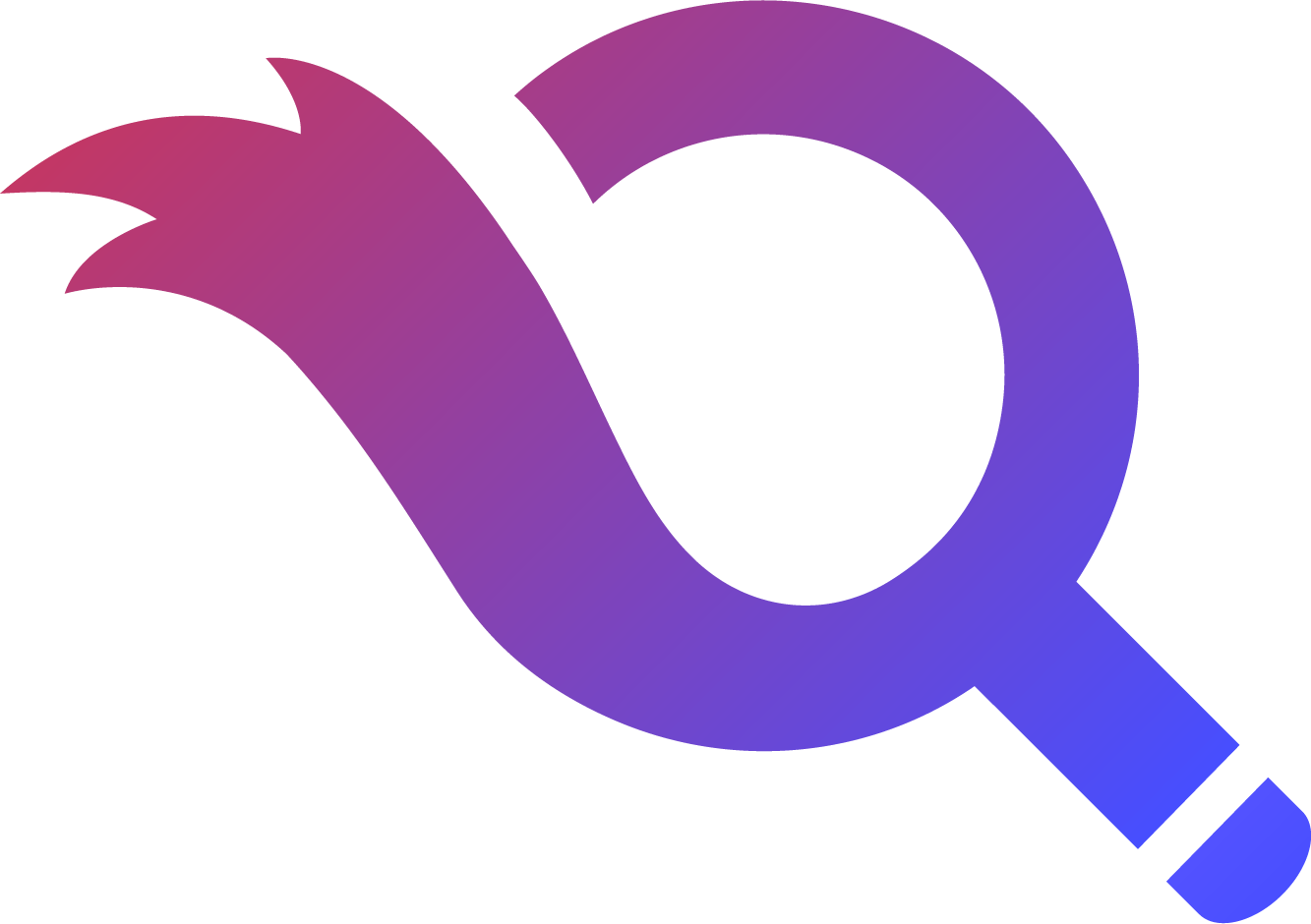
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To learn about Swift concurrency, you can refer to several sessions from WWDC 2024 that cover various aspects of concurrency in Swift. Here are some key points and sessions that will help you get started:
-
Fundamentals of Swift Concurrency:
- The fundamental unit of concurrency in Swift is a task, which represents an independent concurrent execution context. Tasks are lightweight and can be created in large numbers. You can wait for a task to complete to get its result or cancel it if its work becomes unnecessary.
- Tasks can execute concurrently, making them suitable for handling operations like HTTP requests.
You can learn more about this in the session A Swift Tour: Explore Swift’s features and design.
-
Actors in Swift:
- Actors are similar to classes but are designed to encapsulate shared mutable state safely. They automatically protect their state by serializing accesses, ensuring that only a single task executes at a time on an actor.
- Calls to actor methods from outside the context of the actor are asynchronous.
More details can be found in the session A Swift Tour: Explore Swift’s features and design.
-
Concurrency in Practice:
- When adopting Swift concurrency, you might encounter warnings or errors from the Swift compiler about concurrency issues. For example, ensuring that delegate callbacks are executed on the main actor can be crucial for UI updates.
- Swift 6 introduces complete data-race protection, making it easier to write safe concurrent code.
Practical examples and migration strategies are discussed in the session Migrate your app to Swift 6.
-
Testing Concurrent Code:
- When writing concurrent test code, you can use the same concurrency features in Swift as in your production code. The
await
keyword works the same way in tests, allowing other test code to keep the CPU busy while work is pending.
Techniques for testing concurrent code are covered in the session Go further with Swift Testing.
- When writing concurrent test code, you can use the same concurrency features in Swift as in your production code. The
Relevant Sessions:
- Migrate your app to Swift 6
- A Swift Tour: Explore Swift’s features and design
- Go further with Swift Testing
These sessions provide a comprehensive overview of Swift concurrency, from basic concepts to practical implementation and testing.
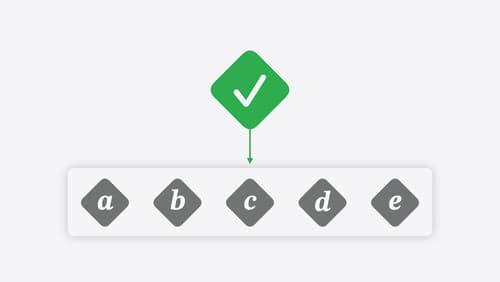
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
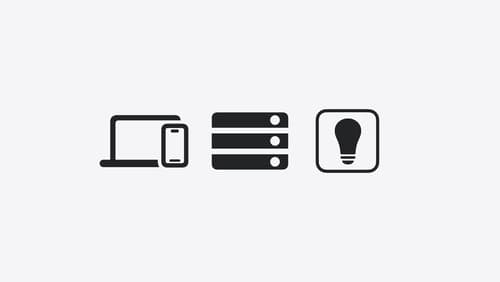
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
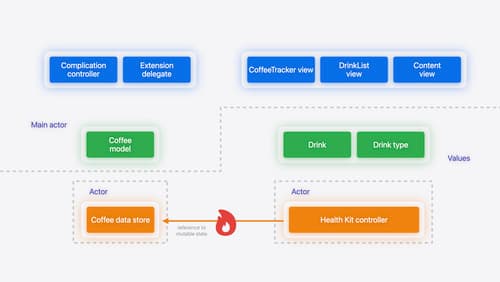
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.