how can you build a multi level nested list with swiftUI? like a table view but it can have n numbers of levels
Generated on 8/2/2024
1 search
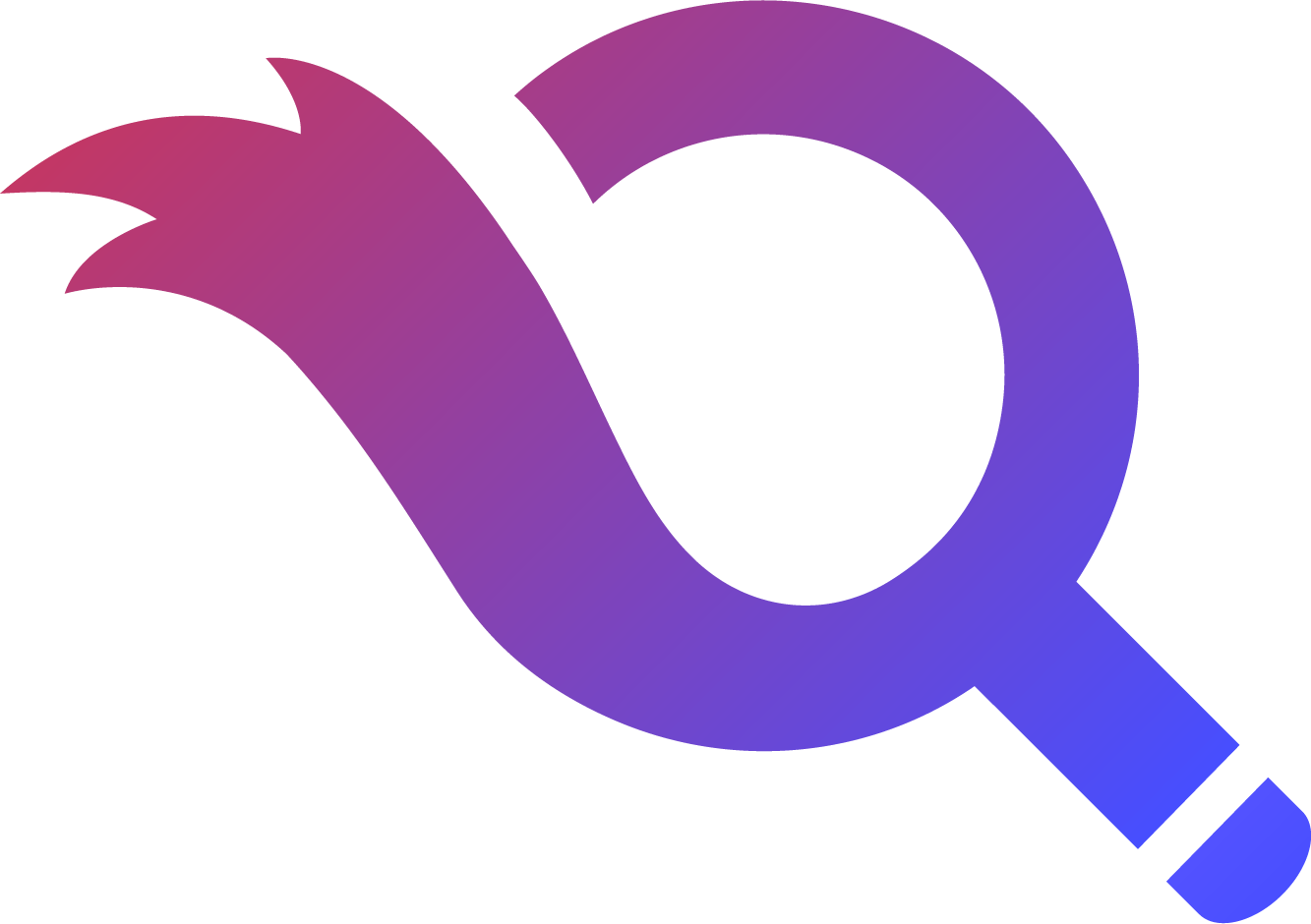
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To build a multi-level nested list in SwiftUI, similar to a table view with multiple levels, you can use a combination of List
, ForEach
, and Section
views. SwiftUI's declarative syntax and view composition capabilities make it straightforward to create such complex structures.
Here's a basic example to get you started:
import SwiftUI
struct ContentView: View {
var body: some View {
List {
ForEach(data, id: \.self) { item in
Section(header: Text(item.title)) {
ForEach(item.subItems, id: \.self) { subItem in
Text(subItem)
}
}
}
}
}
}
struct Item: Identifiable {
var id = UUID()
var title: String
var subItems: [String]
}
let data = [
Item(title: "Level 1 - Item 1", subItems: ["Level 2 - Item 1", "Level 2 - Item 2"]),
Item(title: "Level 1 - Item 2", subItems: ["Level 2 - Item 3", "Level 2 - Item 4"])
]
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Key Points from WWDC Sessions
-
Composition: SwiftUI supports creating lists in various ways, including using
ForEach
for dynamic data. This allows you to nest views and create complex hierarchies. For more details, you can refer to the Demystify SwiftUI containers session. -
Sections: You can use
Section
views to organize your list into different sections, each with optional headers and footers. This is particularly useful for creating multi-level lists. More information can be found in the Demystify SwiftUI containers session. -
Declarative Syntax: SwiftUI's declarative syntax makes it easy to define complex UI hierarchies. You can use
ViewBuilder
closures to declare the children of a container, making it straightforward to nest multiple levels of lists. This is discussed in the SwiftUI essentials session.
Relevant Sessions
By leveraging these techniques, you can create a multi-level nested list in SwiftUI that can handle an arbitrary number of levels.
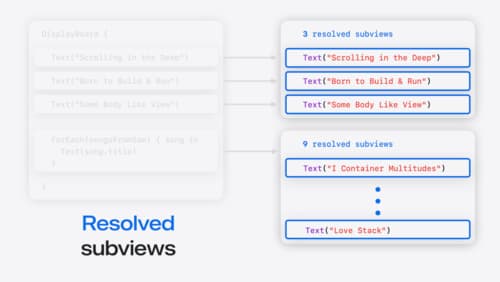
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
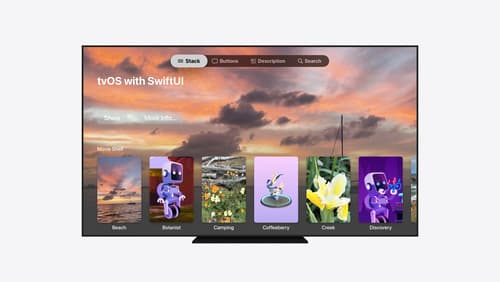
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
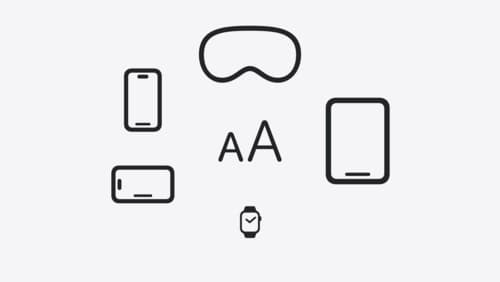
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.
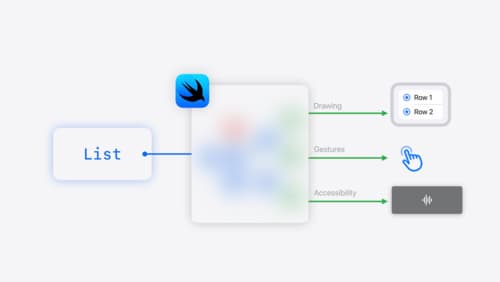
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.