create a macos code example of an extremely large list with high performance
Generated on 8/2/2024
1 search
To create an extremely large list with high performance in macOS using Swift, you can leverage various techniques discussed in the WWDC sessions. Here is an example that demonstrates how to create a high-performance list using Swift:
import SwiftUI
struct ContentView: View {
let items = Array(0..<1000000) // 1 million items
var body: some View {
List(items, id: \.self) { item in
Text("Item \(item)")
}
.onAppear {
// Perform any additional setup or optimizations here
}
}
}
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Key Points for High Performance
-
Efficient Data Structures: Use efficient data structures to store your list items. In this example, an array is used, but for more complex data, consider using more appropriate structures.
-
Lazy Loading: SwiftUI's
List
is inherently optimized for large data sets by lazily loading items as they come into view. -
Avoiding Unnecessary Copies: Minimize the use of reference types and unnecessary copying of data. Swift's value types (like structs) are generally more performant for large data sets.
-
Profiling and Optimization: Regularly profile your application to identify performance bottlenecks. Use tools like Instruments' flame graph to spot issues at a glance. For example, you can use the new flame graph feature in Xcode 16 to identify and optimize slow parts of your code. What’s new in Xcode 16 (19:44).
-
Memory Management: Be mindful of memory allocation and deallocation. Use techniques like autorelease pools to manage temporary memory growth effectively. For more details, you can refer to the session on managing autorelease pool growth in Swift. Analyze heap memory (10:34).
-
Swift Optimizations: Take advantage of Swift's powerful optimizer and ensure whole module optimization is enabled. This can significantly reduce overhead by allowing more inlining and other optimizations. Explore Swift performance (03:21).
By following these guidelines and leveraging the tools and techniques discussed in the WWDC sessions, you can create a high-performance list in macOS using Swift.
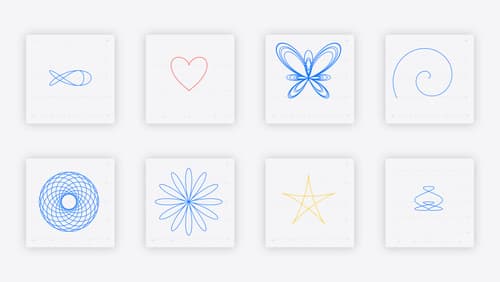
Swift Charts: Vectorized and function plots
The plot thickens! Learn how to render beautiful charts representing math functions and extensive datasets using function and vectorized plots in your app. Whether you’re looking to display functions common in aerodynamics, magnetism, and higher order field theory, or create large interactive heat maps, Swift Charts has you covered.
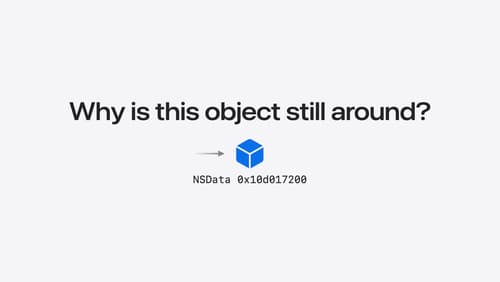
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
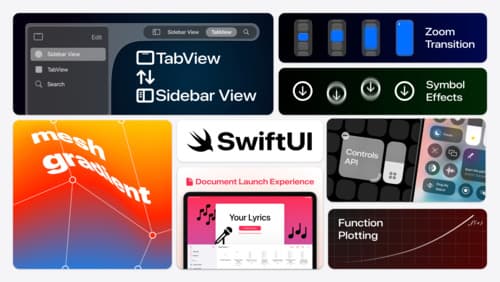
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
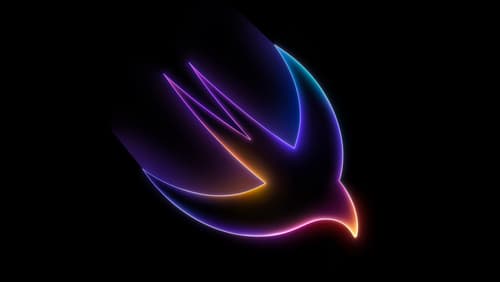
Platforms State of the Union
Discover the newest advancements on Apple platforms.
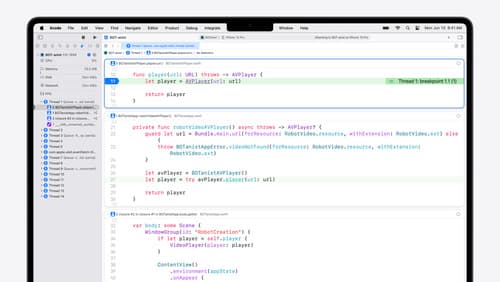
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
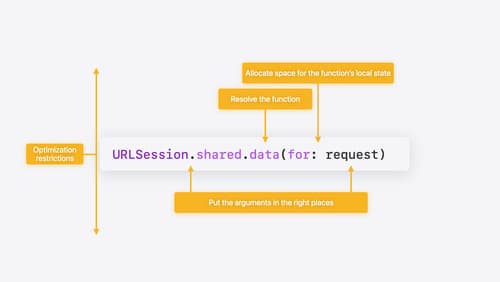
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
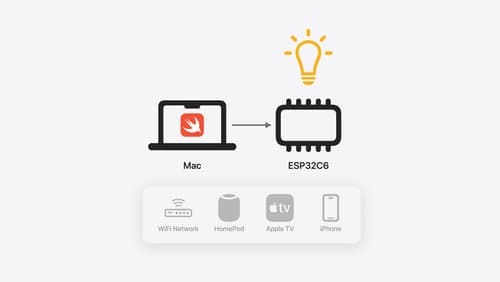
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.