If I have Search intent which basically a deeplink to my search screen. How to update it to integrate naturally with Apple Intelligence scehem
Generated on 8/2/2024
1 search
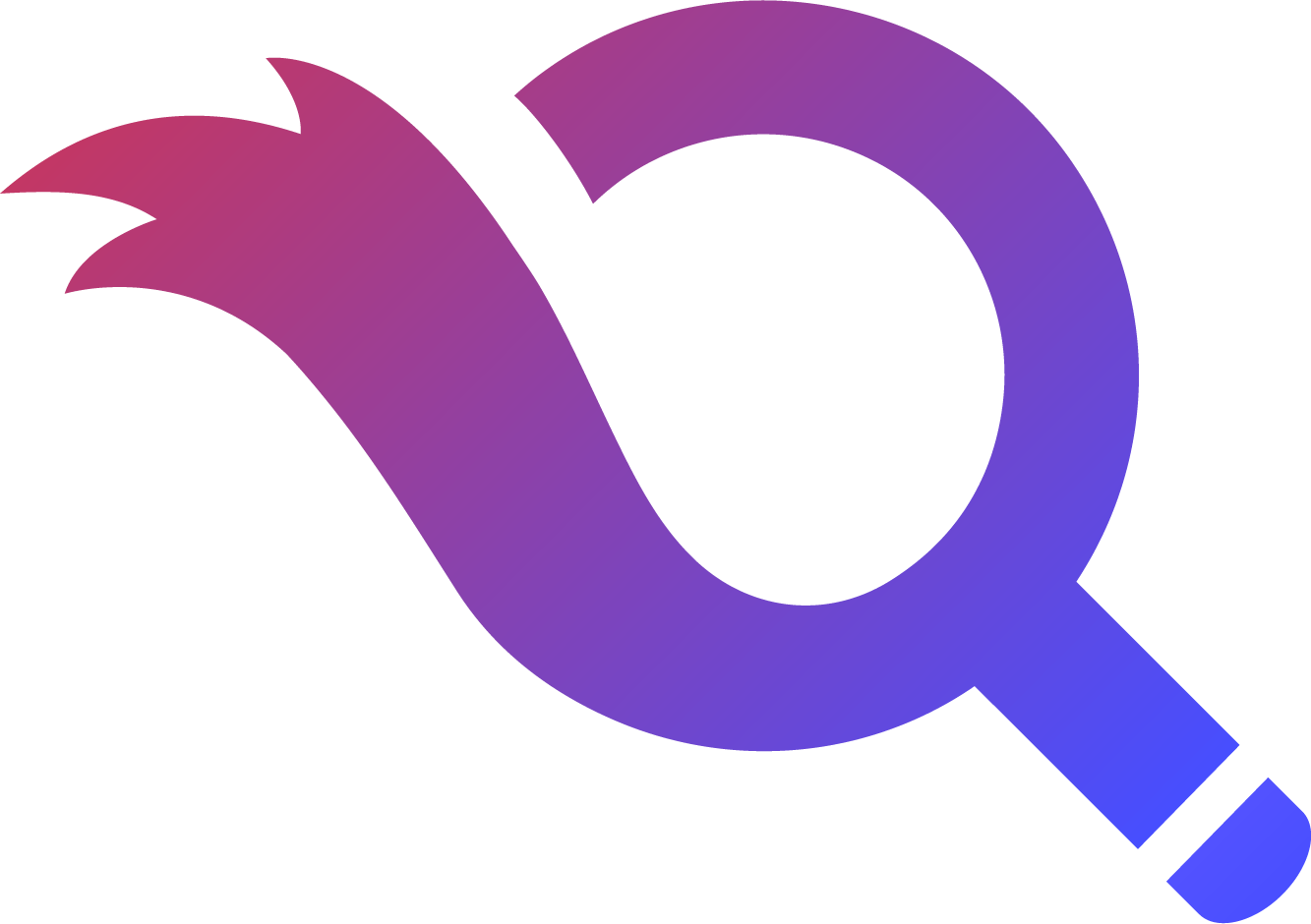
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To update your search intent to integrate naturally with Apple Intelligence, you can follow these steps:
-
Define Your App Intent: Ensure you have an app intent that represents your search functionality. This intent should conform to the new assistant schema for search. For example, if you have a method in your app that routes users to a search results page, you can expose this functionality to Siri.
@AppIntent struct SearchPhotosIntent: AppIntent { static var title: LocalizedStringResource = "Search Photos" @Parameter(title: "Search Criteria") var criteria: String func perform() async throws -> some IntentResult { // Your code to perform the search } }
-
Add Assistant Schema: Add the new assistant schema for search to your app intent declaration. This allows Siri to route users directly to your search results UI.
@AppIntent struct SearchPhotosIntent: AppIntent { static var title: LocalizedStringResource = "Search Photos" @Parameter(title: "Search Criteria") var criteria: String @AssistantSchema static var schema: AssistantSchema { AssistantSchema( domain: .system, intentType: .search, parameters: [ .init(name: "criteria", type: .string) ] ) } func perform() async throws -> some IntentResult { // Your code to perform the search } }
-
Test with Shortcuts: Use the Shortcuts app to create a new action and test your search intent. This helps ensure that the intent works as expected before integrating it with Siri.
// Example of creating a shortcut action let shortcut = Shortcut(name: "Search Photos", intent: SearchPhotosIntent(criteria: "San Fran"))
-
Leverage Apple Intelligence: With the new capabilities of Apple Intelligence, Siri can perform semantic searches. This means Siri can understand the context of your search criteria and provide more relevant results.
// Example of semantic search let searchResults = try await Siri.performSemanticSearch(for: "pets")
For more detailed information, you can refer to the session Bring your app to Siri.
Relevant Sessions
These sessions provide comprehensive guidance on integrating your app with Siri and leveraging the new capabilities of Apple Intelligence.
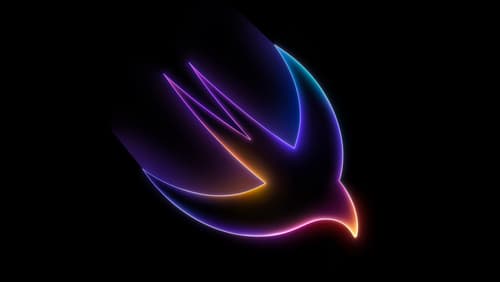
Platforms State of the Union
Discover the newest advancements on Apple platforms.
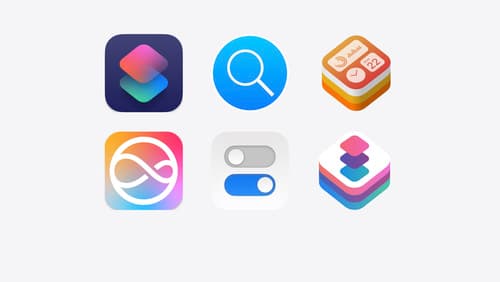
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.
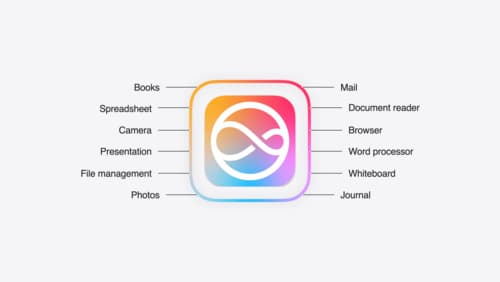
Bring your app to Siri
Learn how to use App Intents to expose your app’s functionality to Siri. Understand which intents are already available for your use, and how to create custom intents to integrate actions from your app into the system. We’ll also cover what metadata to provide, making your entities searchable via Spotlight, annotating onscreen references, and much more.