Is there an equivalent to uicalendarview for SwiftUI?
Generated on 8/2/2024
1 search
Yes, there is an equivalent to UICalendarView
for SwiftUI. You can use the interoperability features of SwiftUI to integrate UIKit views into your SwiftUI app. Specifically, you can create a UIViewRepresentable
to wrap UICalendarView
and use it within your SwiftUI view hierarchy.
For more details on how to achieve this, you can refer to the session SwiftUI essentials which covers the interoperability between SwiftUI and UIKit, including how to use UIKit views in SwiftUI.
Here is a brief example of how you might wrap UICalendarView
in SwiftUI:
import SwiftUI
import UIKit
struct CalendarView: UIViewRepresentable {
func makeUIView(context: Context) -> UICalendarView {
return UICalendarView()
}
func updateUIView(_ uiView: UICalendarView, context: Context) {
// Update the view if needed
}
}
struct ContentView: View {
var body: some View {
CalendarView()
.frame(height: 400)
}
}
This way, you can leverage the existing UIKit components while building your app's UI in SwiftUI.
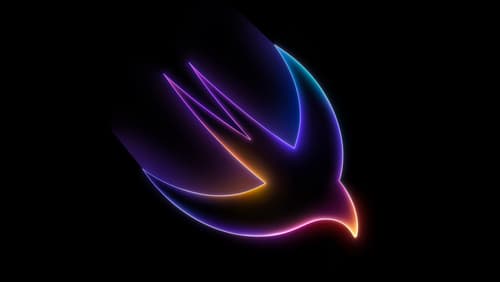
Platforms State of the Union
Discover the newest advancements on Apple platforms.
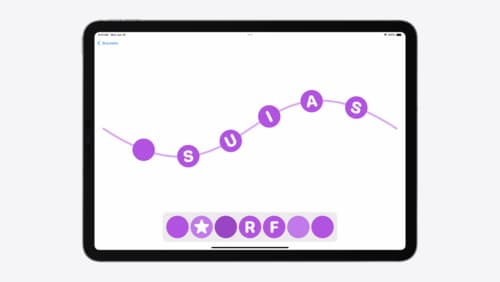
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
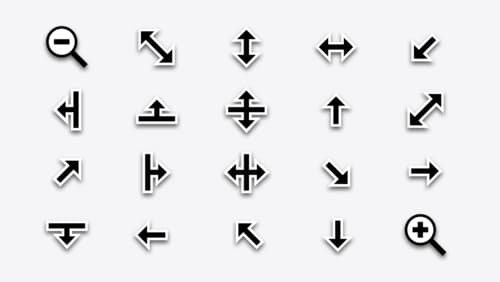
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
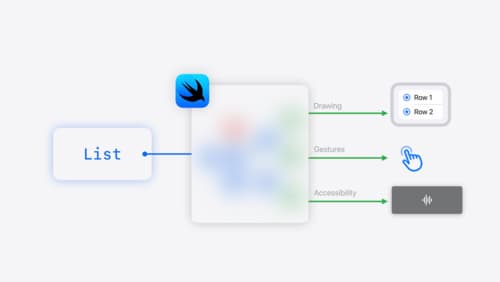
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.