How can I use Combine for network requests?
Asked on 08/02/2024
1 search
To use Combine for network requests, you can leverage its powerful declarative API to handle asynchronous data streams. Here’s a basic example of how you can use Combine to perform a network request:
- Create a URLSession data task publisher: This will be used to make the network request.
- Handle the response: Use Combine operators to process the response, handle errors, and update the UI or data model.
Here’s a simple example in Swift:
import Combine
import Foundation
// Define a struct to decode the JSON response
struct Post: Decodable {
let id: Int
let title: String
let body: String
}
// Create a URL for the request
let url = URL(string: "https://jsonplaceholder.typicode.com/posts/1")!
// Create a URLSession data task publisher
let publisher = URLSession.shared.dataTaskPublisher(for: url)
.map { $0.data }
.decode(type: Post.self, decoder: JSONDecoder())
.receive(on: DispatchQueue.main)
.sink(receiveCompletion: { completion in
switch completion {
case .finished:
print("Request completed successfully.")
case .failure(let error):
print("Request failed with error: \(error)")
}
}, receiveValue: { post in
print("Received post: \(post)")
})
// Store the publisher if needed to keep the subscription alive
var cancellables = Set<AnyCancellable>()
publisher.store(in: &cancellables)
In this example:
dataTaskPublisher(for:)
creates a publisher that wraps a URL session data task.map { $0.data }
extracts the data from the URL response.decode(type:decoder:)
decodes the JSON data into aPost
struct.receive(on:)
ensures that the subsequent operations are performed on the main thread, which is important for updating the UI.sink(receiveCompletion:receiveValue:)
subscribes to the publisher and handles the completion and received value.
For more advanced usage and optimizations, you can explore sessions like A Swift Tour: Explore Swift’s features and design and Discover Swift enhancements in the Vision framework which cover concurrency and async operations in Swift.
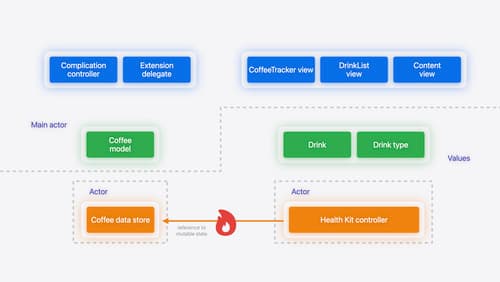
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
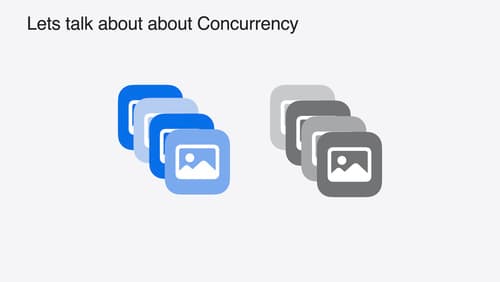
Discover Swift enhancements in the Vision framework
The Vision Framework API has been redesigned to leverage modern Swift features like concurrency, making it easier and faster to integrate a wide array of Vision algorithms into your app. We’ll tour the updated API and share sample code, along with best practices, to help you get the benefits of this framework with less coding effort. We’ll also demonstrate two new features: image aesthetics and holistic body pose.
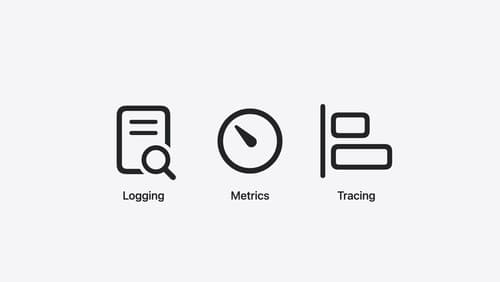
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.