how does async work
Generated on 8/2/2024
1 search
Async functions in Swift are designed to efficiently handle tasks that may need to wait for other operations to complete, such as reading from a disk or messaging another service. Here’s a detailed explanation of how async functions work:
-
Suspension and Resumption:
- When a task is waiting for an asynchronous operation to complete, it suspends to yield the CPU to other tasks that have work to do. This is modeled in code using the
async
andawait
keywords. A function that may suspend is marked with theasync
keyword, and theawait
keyword is used to indicate that a suspension can occur on that line. (A Swift Tour: Explore Swift’s features and design)
- When a task is waiting for an asynchronous operation to complete, it suspends to yield the CPU to other tasks that have work to do. This is modeled in code using the
-
Memory Allocation:
- Async functions keep their local state on a separate stack from the C stack and are split into multiple functions at runtime. When an async function wants to allocate memory, it asks the task for memory. The stack tries to satisfy that from the current slab, and if it can, the task will mark that part of the slab as used and give it to the function. If the allocation doesn't fit, a new slab is allocated with
malloc
. Deallocation hands the memory back to the task, where it becomes marked as unused. (Explore Swift performance)
- Async functions keep their local state on a separate stack from the C stack and are split into multiple functions at runtime. When an async function wants to allocate memory, it asks the task for memory. The stack tries to satisfy that from the current slab, and if it can, the task will mark that part of the slab as used and give it to the function. If the allocation doesn't fit, a new slab is allocated with
-
Partial Functions:
- Async functions are split into partial functions that span the gaps between potential suspension points. For example, if there is one
await
in the function, it results in two partial functions. The first partial function starts with the entry to the original function and runs until the suspension point. The second partial function picks up after theawait
. This ensures that only one partial function is on the C stack at a time. (Explore Swift performance)
- Async functions are split into partial functions that span the gaps between potential suspension points. For example, if there is one
-
Concurrency and Actors:
- Swift provides a feature called actors, which are similar to classes but automatically protect their state by serializing accesses. Calls to actor methods from outside the context of the actor are asynchronous. This helps in managing concurrent accesses safely. (A Swift Tour: Explore Swift’s features and design)
-
Testing Asynchronous Code:
- When writing concurrent test code, you can use the same concurrency features in Swift as in production code. The
await
keyword works the same way in tests, suspending the test and allowing other test code to keep the CPU busy while work is pending. (Go further with Swift Testing)
- When writing concurrent test code, you can use the same concurrency features in Swift as in production code. The
For a more detailed exploration of async functions, you can refer to the session Explore Swift performance.
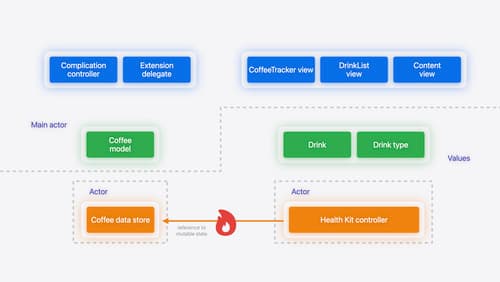
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
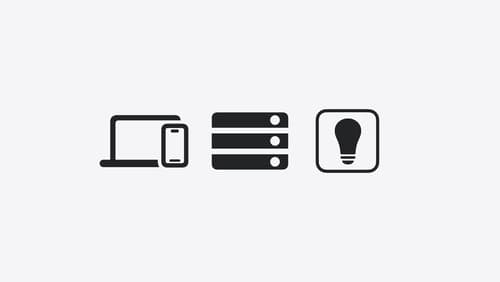
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
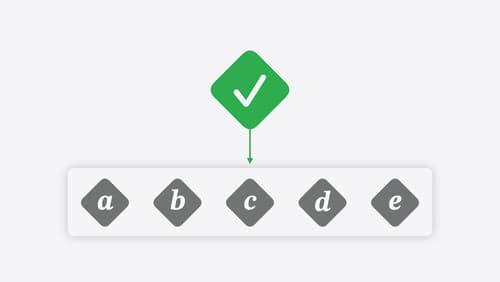
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
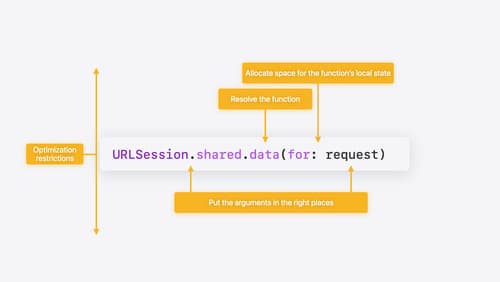
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.