make a scrollview snap to page
Generated on 8/2/2024
1 search
To make a ScrollView
snap to a page in SwiftUI, you can use the paging
behavior provided by SwiftUI. This can be achieved by setting the paging
modifier on your ScrollView
. Here's an example of how you can do this:
import SwiftUI
struct PagingScrollView: View {
var body: some View {
ScrollView(.horizontal, showsIndicators: false) {
HStack(spacing: 0) {
ForEach(0..<10) { index in
Text("Page \(index)")
.frame(width: UIScreen.main.bounds.width, height: UIScreen.main.bounds.height)
.background(index % 2 == 0 ? Color.blue : Color.green)
}
}
}
.paging()
}
}
struct PagingScrollView_Previews: PreviewProvider {
static var previews: some View {
PagingScrollView()
}
}
In this example, the ScrollView
is set to scroll horizontally, and the paging
modifier is applied to make it snap to each page as you scroll.
For more advanced scroll effects, you can refer to the session Create custom visual effects with SwiftUI which covers various scroll effects and transitions you can apply to your ScrollView
.
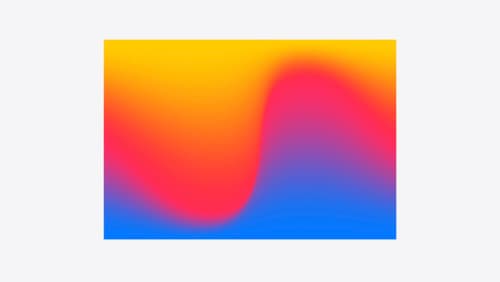
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
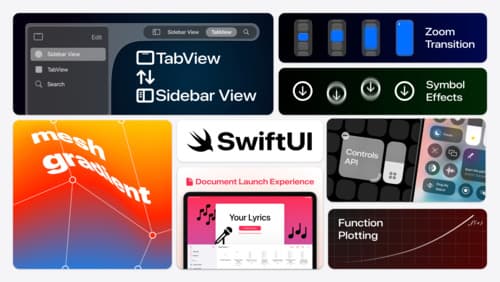
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
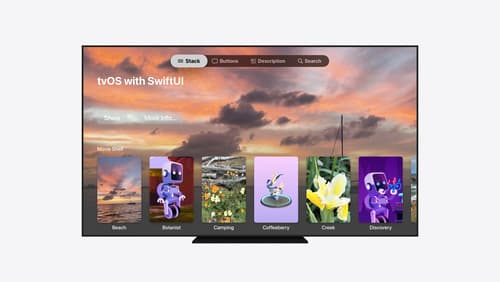
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.