how do I resize a sprite in spitekit
Generated on 8/2/2024
1 search
To resize a sprite in SpriteKit, you can adjust the size
property of the SKSpriteNode
. Here is a simple example in Swift:
import SpriteKit
class GameScene: SKScene {
override func didMove(to view: SKView) {
let sprite = SKSpriteNode(imageNamed: "exampleSprite")
sprite.position = CGPoint(x: self.frame.midX, y: self.frame.midY)
// Set the initial size of the sprite
sprite.size = CGSize(width: 100, height: 100)
self.addChild(sprite)
// Resize the sprite after 2 seconds
let resizeAction = SKAction.resize(toWidth: 200, height: 200, duration: 2.0)
sprite.run(resizeAction)
}
}
In this example:
- We create an
SKSpriteNode
with an image named "exampleSprite". - We set its initial size to 100x100 points.
- We then create an
SKAction
to resize the sprite to 200x200 points over 2 seconds and run this action on the sprite.
If you need more detailed information on resizing elements in other contexts, such as SwiftUI or UIKit, you might find the following sessions from WWDC useful:
- Get started with Dynamic Type - This session covers how to handle images and symbols resizing in SwiftUI and UIKit.
- Work with windows in SwiftUI - This session discusses resizing windows and their content in SwiftUI.
- Dive deep into volumes and immersive spaces - This session explains how to handle resizing of volumes in immersive spaces.
Feel free to check these sessions for more comprehensive insights!
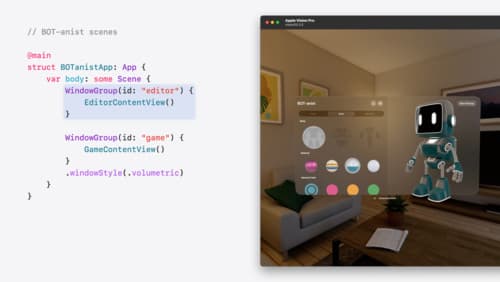
Work with windows in SwiftUI
Learn how to create great single and multi-window apps in visionOS, macOS, and iPadOS. Discover tools that let you programmatically open and close windows, adjust position and size, and even replace one window with another. We’ll also explore design principles for windows that help people use your app within their workflows.
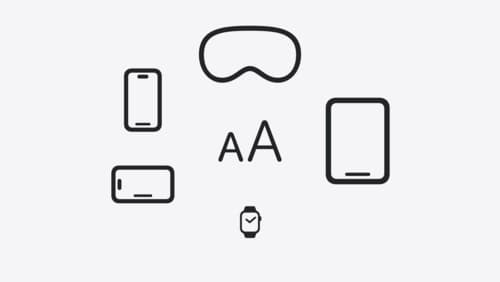
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.
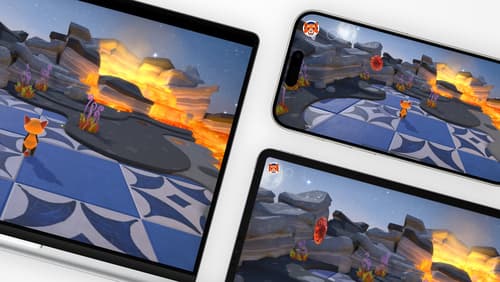
Design advanced games for Apple platforms
Learn how to adapt your high-end game so it feels at home on Mac, iPad, and iPhone. We’ll go over how to make your game look stunning on different displays, tailor your input and controls to be intuitive on each device, and take advantage of Apple technologies that deliver great player experiences.
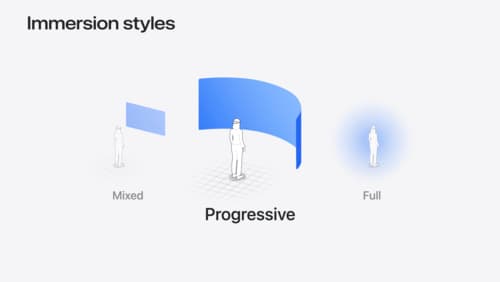
Dive deep into volumes and immersive spaces
Discover powerful new ways to customize volumes and immersive spaces in visionOS. Learn to fine-tune how volumes resize and respond to people moving around them. Make volumes and immersive spaces interact through the power of coordinate conversions. Find out how to make your app react when people adjust immersion with the Digital Crown, and use a surrounding effect to dynamically customize the passthrough tint in your immersive space experience.