How do I implement Shortcuts for my app?
Generated on 8/3/2024
1 search
To implement Shortcuts for your app, you can follow the guidance provided in the session titled Bring your app’s core features to users with App Intents. Here are the key steps:
-
Understand the App Intents Framework:
- The App Intents framework is the foundation for integrating your app with Shortcuts, Siri, widgets, and more. It allows you to define actions that users can trigger from various parts of the system.
-
Define Shortcuts Actions:
- A shortcuts action is an intent. All intents appear by default as actions in the Shortcuts app. An intent is a type that conforms to the
AppIntent
protocol. It has two required pieces: a localizable title and a perform method. - Example:
struct OpenPinnedTrailIntent: AppIntent { static var title: LocalizedStringResource = "Open Pinned Trail" func perform() async throws -> some IntentResult { // Code to open the pinned trail in your app return .result() } }
- A shortcuts action is an intent. All intents appear by default as actions in the Shortcuts app. An intent is a type that conforms to the
-
Create App Shortcuts:
- An app shortcut is a wrapper around an intent that highlights it as an important function of your app. This makes it available in Spotlight, Siri, and other system features.
- Example:
struct MyAppShortcutsProvider: AppShortcutsProvider { static var appShortcuts: [AppShortcut] { return [ AppShortcut(intent: OpenPinnedTrailIntent(), phrases: ["Open pinned trail in MyApp"]) ] } }
-
Test in Shortcuts App:
- Use the Shortcuts app to test your intents. Create a new shortcut, add your app's action, and verify it performs as expected.
- Example from the session:
let shortcut = Shortcut(intent: OpenPinnedTrailIntent())
-
Automatic Registration:
- The App Intents framework automatically detects and registers your intents, so they are available as soon as the app is installed. No additional registration code is needed.
For more detailed guidance, you can watch the session Bring your app’s core features to users with App Intents starting from the "Building the code" chapter.
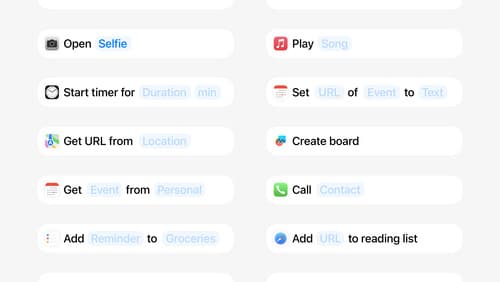
Design App Intents for system experiences
App Intents power system experiences in controls, Spotlight, Siri, and more. Find out how to identify the functionality that’s best for App Intents, and how to use parameters to make these intents flexible. Learn how to use App Intents to allow people to take action outside your app, and see examples of when to navigate into your app to show contextual information.
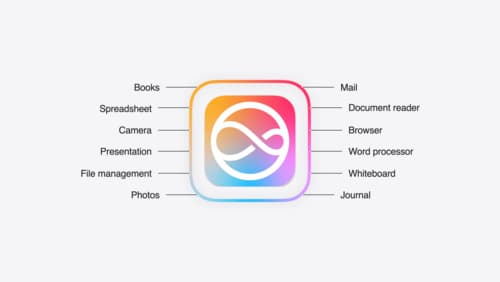
Bring your app to Siri
Learn how to use App Intents to expose your app’s functionality to Siri. Understand which intents are already available for your use, and how to create custom intents to integrate actions from your app into the system. We’ll also cover what metadata to provide, making your entities searchable via Spotlight, annotating onscreen references, and much more.
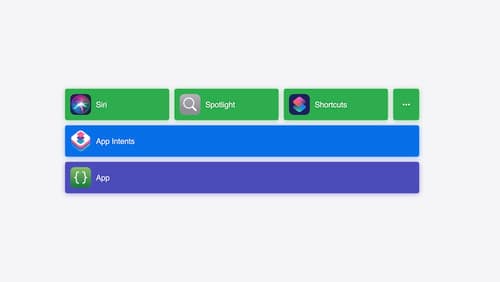
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.