How to write native photo picker
Generated on 8/3/2024
1 search
To write a native photo picker, you can use the PhotoKit framework provided by Apple. Here are the steps to implement a photo picker in your app:
-
Import the PhotoKit framework:
import PhotosUI
-
Create a PHPickerViewController:
var configuration = PHPickerConfiguration() configuration.selectionLimit = 1 // Set the limit to the number of photos you want to pick let picker = PHPickerViewController(configuration: configuration) picker.delegate = self
-
Present the picker:
present(picker, animated: true, completion: nil)
-
Implement the delegate method to handle the selected photos:
extension YourViewController: PHPickerViewControllerDelegate { func picker(_ picker: PHPickerViewController, didFinishPicking results: [PHPickerResult]) { picker.dismiss(animated: true, completion: nil) for result in results { result.itemProvider.loadObject(ofClass: UIImage.self) { (object, error) in if let image = object as? UIImage { DispatchQueue.main.async { // Use the selected image self.imageView.image = image } } } } } }
For more detailed information on working with PhotoKit, you can refer to the session Build a great Lock Screen camera capture experience from WWDC 2024. This session covers various aspects of capturing and handling photos and videos, including permissions and best practices.
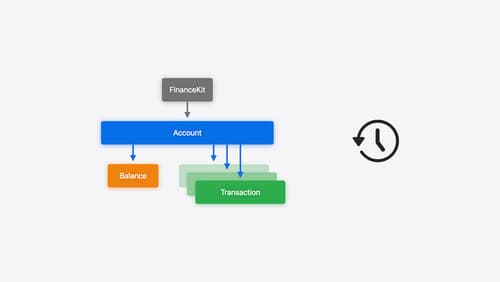
Meet FinanceKit
Learn how FinanceKit lets your financial management apps seamlessly and securely share on-device data from Apple Cash, Apple Card, and more, with user consent and control. Find out how to request one-time and ongoing access to accounts, transactions, and balances — and how to build great experiences for iOS and iPadOS.
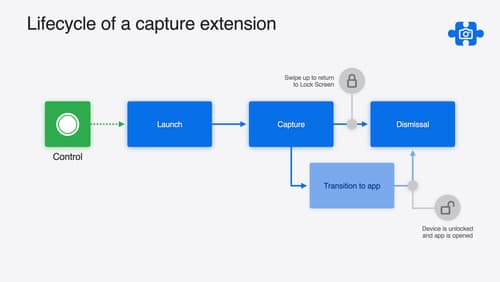
Build a great Lock Screen camera capture experience
Find out how the LockedCameraCapture API can help you bring your capture application’s most useful information directly to the Lock Screen. Examine the API’s features and functionality, learn how to get started creating a capture extension, and find out how that extension behaves when the device is locked.
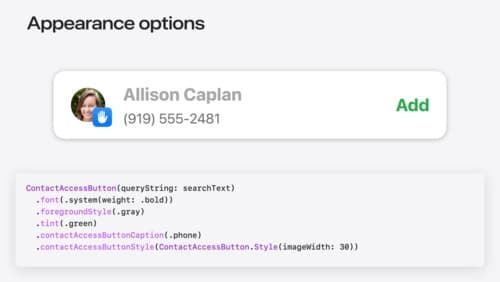
Meet the Contact Access Button
Learn about the new Contacts authorization modes and how to improve Contacts access in your app. Discover how to integrate the Contact Access Button into your app to share additional contacts on demand and provide an easier path to Contacts authorization. We’ll also cover Contacts security features and an alternative API to be used if the button isn’t appropriate for your app.
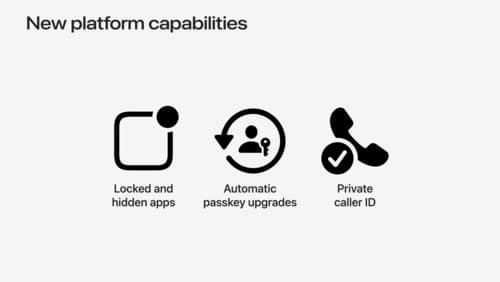
What’s new in privacy
At Apple, we believe privacy is a fundamental human right. Learn about new and improved permission flows and other features that manage data in a privacy-preserving way, so that you can focus on creating great app experiences.
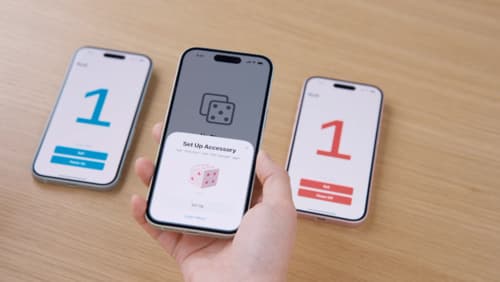
Meet AccessorySetupKit
Elevate your accessory setup experience with AccessorySetupKit. Display a beautiful pairing dialog with an image of your Bluetooth or Wi-Fi accessory — no trip to the Settings app required. Discover how to improve privacy by pairing only your app with an accessory. And learn how you can migrate existing accessories so they can be managed by AccessorySetupKit.