Tell me basics of concurrency
Generated on 8/3/2024
1 search
Concurrency in Swift is a powerful feature that allows you to perform multiple tasks simultaneously, improving the efficiency and responsiveness of your applications. Here are some basics of concurrency in Swift:
-
Data Race Safety: One of the primary goals of Swift concurrency is to ensure data race safety. Data races occur when multiple threads access shared data simultaneously, and at least one thread modifies the data. This can lead to unpredictable behavior and crashes. Swift 6 achieves data race safety by default, turning potential data race issues into compile-time errors, which significantly improves the security and stability of your app (What’s new in Swift).
-
Concurrency Architecture: In Swift, concurrency can be managed using actors and the
async/await
pattern. Actors are used to protect mutable state and ensure that only one task can access the state at a time. Theasync/await
pattern allows you to write asynchronous code that is easy to read and maintain. For example, UI views and models can run on the main actor, while background operations are performed on dedicated actors (Migrate your app to Swift 6). -
Structured Concurrency: Swift's structured concurrency model helps organize concurrent code in a clear and manageable way. Tasks are used to represent units of work that can be executed concurrently. The
await
keyword is used to pause the execution of a task until the awaited task completes. This model helps avoid the hidden complexity of concurrency and makes the code easier to understand and maintain (A Swift Tour: Explore Swift’s features and design). -
Adopting Concurrency Features: When migrating to Swift 6, you can gradually adopt concurrency features. For instance, you can enable strict concurrency checking in your project's build settings to identify and fix potential concurrency issues. This can be done on a per-target basis, allowing you to incrementally update your codebase (Migrate your app to Swift 6).
-
Handling Legacy Code: When working with legacy code or third-party libraries that are not yet updated for Swift concurrency, you may encounter challenges. Swift provides mechanisms to handle these situations, such as using the
@preconcurrency
attribute to indicate that certain code is not concurrency-safe (Migrate your app to Swift 6).
For a more detailed exploration of concurrency in Swift, you can refer to the session A Swift Tour: Explore Swift’s features and design which covers concurrency starting at 18:33.
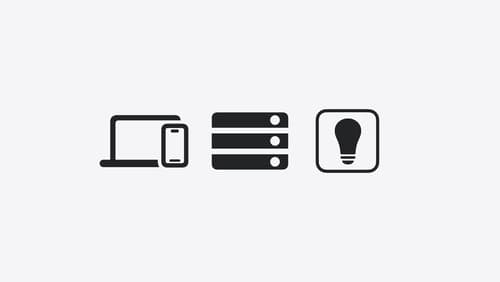
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
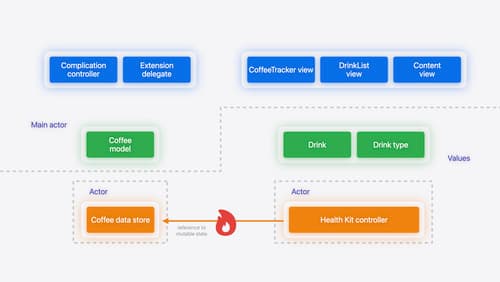
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
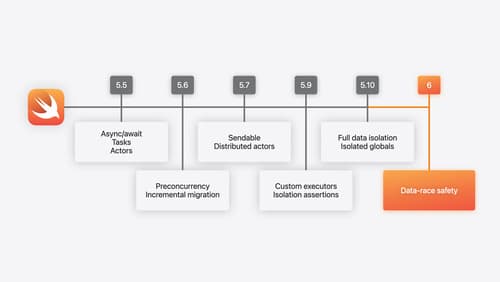
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.