How can I check if a task is using the main thread or not?
Generated on 8/3/2024
1 search
To check if a task is using the main thread or not, you can use several methods and tools provided by Xcode and Swift. Here are a few approaches:
-
Thread Performance Checker in Xcode:
- Xcode 16 introduces a thread performance checker that can surface runtime issues related to thread usage. It can pinpoint the exact line of code causing the issue, even if it doesn't reproduce locally. You can set a breakpoint and use the unified backtrace view to trace where the call is coming from and check if it's on the main thread. For more details, you can refer to the session What’s new in Xcode 16.
-
Main Actor Annotations in Swift:
- In Swift 6, many delegates and protocols, especially those related to UI frameworks like SwiftUI, are annotated to operate only on the main actor. You can use the
@MainActor
annotation to ensure that a function or property is accessed on the main thread. If you need to check if a function is running on the main actor, you can useMainActor.assumeIsolated
to assert that the code is running on the main actor. This will trap if the function is not called from the main actor, helping you catch concurrency issues early. For more information, see the session Migrate your app to Swift 6.
- In Swift 6, many delegates and protocols, especially those related to UI frameworks like SwiftUI, are annotated to operate only on the main actor. You can use the
-
Concurrency Guarantees in Callbacks:
- When dealing with delegate callbacks, it's important to understand the concurrency guarantees provided by the framework. Some callbacks guarantee they will always be on the main thread, while others do not. You can annotate delegate protocols or callbacks to specify that they should always be on the main actor, making these guarantees explicit. For more details, refer to the session Migrate your app to Swift 6.
By using these tools and techniques, you can effectively check and ensure that your tasks are running on the main thread when necessary.
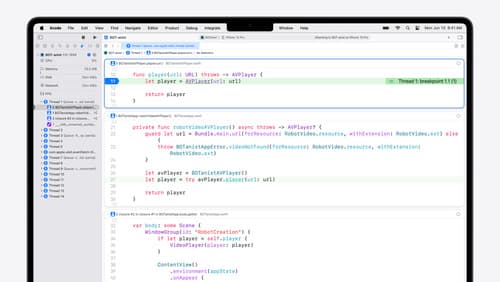
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
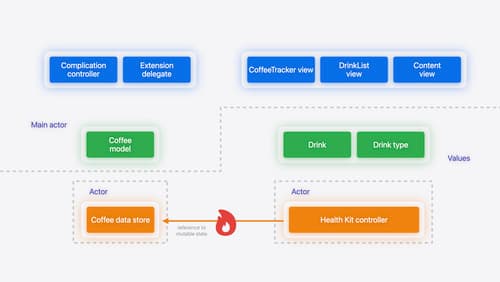
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.