what is the latest adding in URLSession and how to retry the network requests
Generated on 8/3/2024
1 search
The latest addition to URLSession
and how to retry network requests was not directly covered in the provided context. However, I can provide some general information based on recent updates and common practices.
Latest Additions in URLSession
Recent updates to URLSession
have focused on improving performance, security, and ease of use. Some of the notable features include:
- Async/Await Support: Swift's concurrency model has been integrated into
URLSession
, allowing for more readable and maintainable asynchronous code. - Background Tasks: Enhanced support for background URL sessions, which allows for tasks to continue running even when the app is not in the foreground.
- HTTP/2 and HTTP/3 Support: Improved support for modern HTTP protocols, which can provide better performance and reliability.
Retrying Network Requests
Retrying network requests can be handled in several ways, depending on the specific requirements and error conditions. Here are some common strategies:
- Exponential Backoff: Implementing a retry mechanism with exponential backoff to avoid overwhelming the server.
- Retry Policies: Defining custom retry policies based on the type of error (e.g., network timeout, server error).
- Using Combine or Async/Await: Leveraging Swift's concurrency features to handle retries in a more declarative manner.
Example with Async/Await
Here's a simple example of how you might implement a retry mechanism using Swift's async/await:
import Foundation
func fetchData(from url: URL) async throws -> Data {
let maxRetries = 3
var currentAttempt = 0
var lastError: Error?
while currentAttempt < maxRetries {
do {
let (data, _) = try await URLSession.shared.data(from: url)
return data
} catch {
lastError = error
currentAttempt += 1
// Implement a delay before retrying
try await Task.sleep(nanoseconds: UInt64(1_000_000_000 * currentAttempt)) // Exponential backoff
}
}
throw lastError ?? URLError(.unknown)
}
For more detailed information on Swift concurrency and error handling, you can refer to the session A Swift Tour: Explore Swift’s features and design which covers concurrency and error handling in Swift.
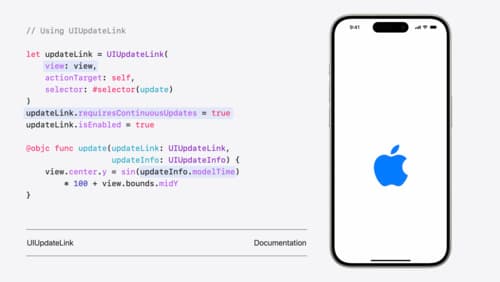
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.
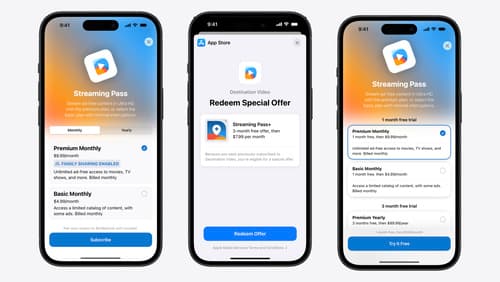
What’s new in StoreKit and In-App Purchase
Learn how to build and deliver even better purchase experiences using the App Store In-App Purchase system. We’ll demo new StoreKit views control styles and new APIs to improve your subscription customization, discuss new fields for transaction-level information, and explore new testability in Xcode. We’ll also review an important StoreKit deprecation.
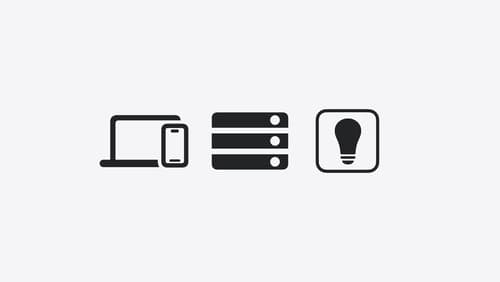
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
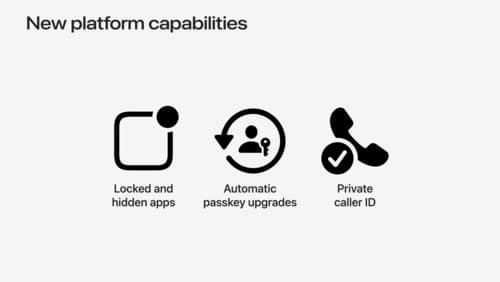
What’s new in privacy
At Apple, we believe privacy is a fundamental human right. Learn about new and improved permission flows and other features that manage data in a privacy-preserving way, so that you can focus on creating great app experiences.
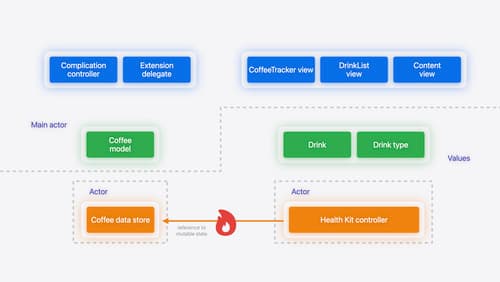
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.