how to use swift models
Asked on 08/03/2024
1 search
To use Swift models with SwiftData, you can follow these steps:
-
Import the Framework: First, ensure you import the SwiftData framework into your project.
-
Define Your Model: Use the
@Model
macro to define your data models. This macro helps in persisting your app's information with a lightweight API that feels natural in Swift.import SwiftData @Model class Trip { var destination: String var date: Date var notes: String? init(destination: String, date: Date, notes: String? = nil) { self.destination = destination self.date = date self.notes = notes } }
-
Customize the Schema: You can further refine your model using attributes to specify behaviors on properties and relationships to describe how models relate to one another. For example, you can use the
@Attribute
macro to define unique constraints or indexing.@Model class Trip { @Attribute(.unique) var destination: String var date: Date var notes: String? init(destination: String, date: Date, notes: String? = nil) { self.destination = destination self.date = date self.notes = notes } }
-
Model Container: Use the
modelContainer
modifier on yourWindowGroup
to tell the entire view hierarchy about your model.@main struct TripsApp: App { var body: some Scene { WindowGroup { ContentView() .modelContainer(for: Trip.self) } } }
-
Querying Data: You can fetch and query your models from the model container. This allows your views to populate with data from the model container.
struct ContentView: View { @Query var trips: [Trip] var body: some View { List(trips) { trip in Text(trip.destination) } } }
For more detailed information, you can refer to the session "What’s new in SwiftData" which covers the adoption of SwiftData, customizing the schema, and other new features like indexing and unique constraints.
Relevant Sessions
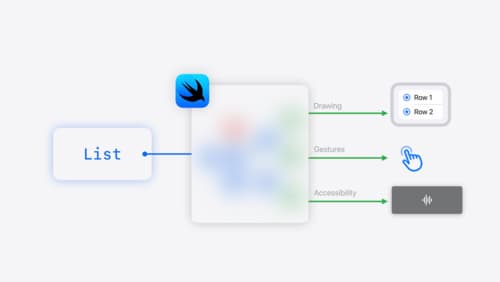
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
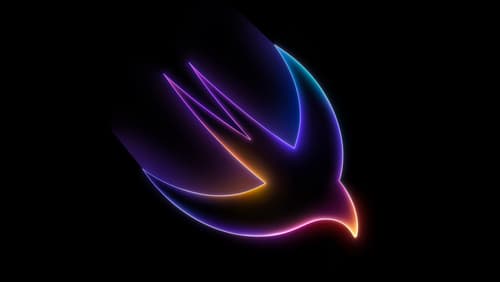
Platforms State of the Union
Discover the newest advancements on Apple platforms.
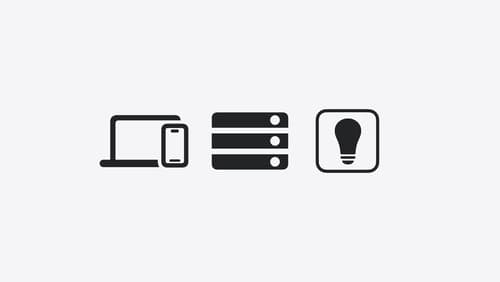
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.