Create a MeshGradient I can use as a view background that’s animated and looks like swirling Northern Lights
Asked on 08/03/2024
1 search
To create a MeshGradient that looks like swirling Northern Lights and can be used as a view background in SwiftUI, you can follow the steps outlined in the "Create custom visual effects with SwiftUI" session from WWDC 2024. Here’s a basic example to get you started:
import SwiftUI
struct NorthernLightsView: View {
@State private var controlPoints: [SIMD2<Float>] = [
SIMD2<Float>(0.1, 0.1),
SIMD2<Float>(0.5, 0.1),
SIMD2<Float>(0.9, 0.1),
SIMD2<Float>(0.1, 0.5),
SIMD2<Float>(0.5, 0.5),
SIMD2<Float>(0.9, 0.5),
SIMD2<Float>(0.1, 0.9),
SIMD2<Float>(0.5, 0.9),
SIMD2<Float>(0.9, 0.9)
]
@State private var colors: [Color] = [
.blue, .green, .purple,
.cyan, .mint, .pink,
.indigo, .teal, .orange
]
var body: some View {
MeshGradient(
colors: colors,
controlPoints: controlPoints
)
.animation(
Animation.linear(duration: 5)
.repeatForever(autoreverses: true)
)
.onAppear {
animateControlPoints()
}
.ignoresSafeArea()
}
private func animateControlPoints() {
withAnimation {
controlPoints = controlPoints.map { _ in
SIMD2<Float>(Float.random(in: 0...1), Float.random(in: 0...1))
}
}
}
}
struct ContentView: View {
var body: some View {
NorthernLightsView()
}
}
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Explanation:
- MeshGradient Setup: The
MeshGradient
view is created using a 3x3 grid of control points and corresponding colors. - Animation: The control points are animated to move randomly within the grid, creating a dynamic swirling effect.
- OnAppear: The
animateControlPoints
function is called when the view appears, starting the animation.
For more details on creating custom visual effects with SwiftUI, you can refer to the Create custom visual effects with SwiftUI (07:10) session from WWDC 2024.
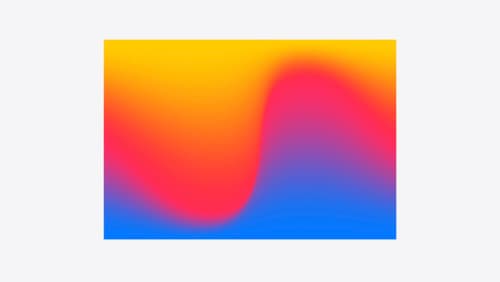
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
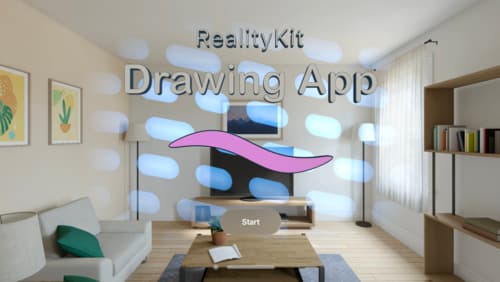
Build a spatial drawing app with RealityKit
Harness the power of RealityKit through the process of building a spatial drawing app. As you create an eye-catching spatial experience that integrates RealityKit with ARKit and SwiftUI, you’ll explore how resources work in RealityKit and how to use features like low-level mesh and texture APIs to achieve fast updates of the users’ brush strokes.