how can I use UIApplication.shared.beginBackgroundTask from an isolated actor?
Asked on 08/03/2024
1 search
To use UIApplication.shared.beginBackgroundTask
from an isolated actor, you can leverage the @MainActor
annotation to ensure that the code runs on the main actor. This is important because UIApplication.shared
is isolated to the main actor, and accessing it from a different actor would require asynchronous context switching.
Here's a step-by-step approach based on the information from the WWDC session "Migrate your app to Swift 6":
-
Annotate the Function with
@MainActor
: This ensures that the function runs on the main actor, which is necessary for accessingUIApplication.shared
. -
Use
@MainActor
in Protocols: If the function is part of a protocol, you can annotate the protocol to guarantee that all its methods are called on the main actor. -
Use
assume isolated
: If you are certain that the function will always be called on the main actor, you can useassume isolated
to inform the compiler about this guarantee.
Here is an example implementation:
@MainActor
func startBackgroundTask() {
let taskID = UIApplication.shared.beginBackgroundTask {
// Cleanup code if the task expires
}
// Perform your background task here
UIApplication.shared.endBackgroundTask(taskID)
}
If you are working within a protocol, you can annotate the protocol and its methods:
@MainActor
protocol BackgroundTaskHandler {
func startBackgroundTask()
}
class MyBackgroundTaskHandler: BackgroundTaskHandler {
func startBackgroundTask() {
let taskID = UIApplication.shared.beginBackgroundTask {
// Cleanup code if the task expires
}
// Perform your background task here
UIApplication.shared.endBackgroundTask(taskID)
}
}
For more details on using @MainActor
and assume isolated
, you can refer to the session Migrate your app to Swift 6 (24:15).
Relevant Sessions
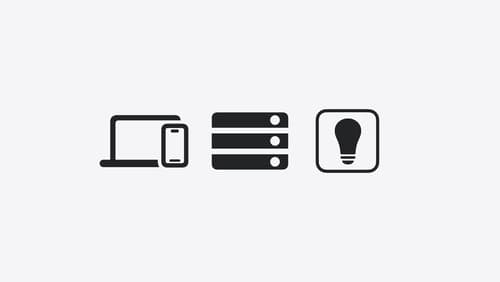
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
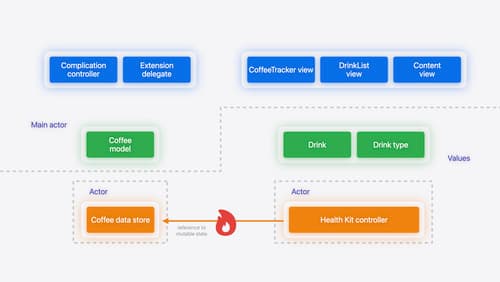
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.