How to use actor?
Generated on 8/3/2024
1 search
To use actors in Swift, you can follow these steps:
-
Define an Actor: Actors are similar to classes but are designed to handle concurrency by serializing access to their state. You can define an actor using the
actor
keyword.actor UserStore { var users: [String] = [] func addUser(_ user: String) { users.append(user) } func getUsers() -> [String] { return users } }
-
Accessing Actor Methods: Calls to actor methods from outside the actor are asynchronous. You need to use the
await
keyword to call these methods.let userStore = UserStore() Task { await userStore.addUser("Alice") let users = await userStore.getUsers() print(users) }
-
Concurrency Safety: Actors automatically protect their state by serializing accesses. Only a single task is allowed to execute at a time on an actor. This helps in avoiding data races and ensuring thread safety.
actor UserStore { private var users: [String] = [] func addUser(_ user: String) { users.append(user) } func getUsers() -> [String] { return users } }
-
Using
MainActor
: If you need to ensure that certain code runs on the main actor (main thread), you can use the@MainActor
attribute.@MainActor class ViewModel: ObservableObject { @Published var data: String = "" func updateData() { data = "Updated" } }
-
Assume Isolated: When you know for certain that a call will be on a particular actor, you can use the
assumeIsolated
method to tell the compiler this. This does not start a new task but tells Swift that the code is already running on the specified actor.@MainActor func updateUI() { // UI update code } func someFunction() { MainActor.assumeIsolated { updateUI() } }
For more detailed information, you can refer to the following sessions from WWDC:
These sessions cover the use of actors, concurrency, and migration to Swift 6, which includes enhanced data-race safety features.
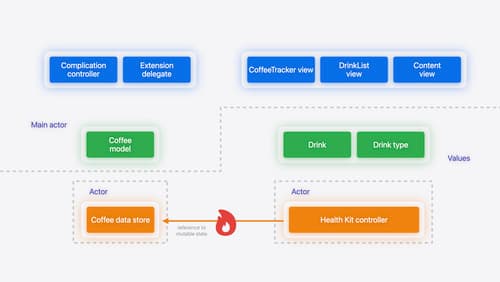
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
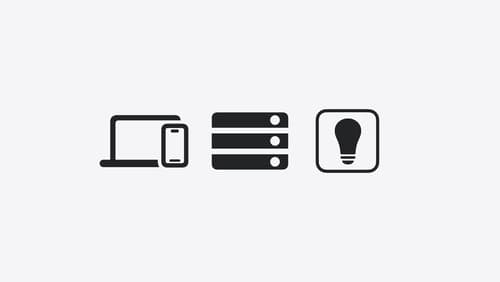
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.