what is the best way to implement a REST-full server API
Generated on 8/3/2024
1 search
To implement a RESTful server API using Swift, you can leverage the Swift on Server ecosystem, which provides robust tools and libraries for building server applications. Here are the key steps and best practices:
-
Choose a Framework: Use a popular server-side Swift framework like Vapor. Vapor is a powerful web framework that simplifies the process of building server applications.
-
Define Your API: Use OpenAPI to document your API. The SwiftOpenAPI generator can help you generate server and client code from your OpenAPI specifications. This ensures your API is well-documented and consistent.
-
Set Up Your Project: Create a new Swift package and set up your project to use Vapor and the OpenAPI generator. You can use editors like Xcode, VS Code, or any other editor that supports the Language Server Protocol.
-
Implement Endpoints:
- GET Method: Implement a method to list all events. This method should return a JSON array of events.
- POST Method: Implement a method to create a new event. This method should accept a JSON body and return appropriate status codes (e.g., 201 for success, 400 for errors).
-
Database Integration: Use a database driver to persist data. For example, you can use PostgresNeo for PostgreSQL, which provides an asynchronous interface and a built-in connection pool.
-
Concurrency: Utilize Swift's concurrency features to handle highly concurrent workloads efficiently. Swift's tasks and async/await syntax make it easier to write scalable and responsive server applications.
-
Testing and Debugging: Use built-in terminals in your editor to run and debug your service. You can use tools like
curl
to test your endpoints. -
Explore More Libraries: The Swift on Server ecosystem offers various libraries for networking, observability, message streaming, and more. Explore these libraries to enhance your server application.
For a detailed walkthrough, you can refer to the session Explore the Swift on Server ecosystem.
Relevant Sessions
- Explore the Swift on Server ecosystem
- Explore App Store server APIs for In-App Purchase
- A Swift Tour: Explore Swift’s features and design
These sessions provide comprehensive insights into building server applications with Swift, including practical examples and best practices.
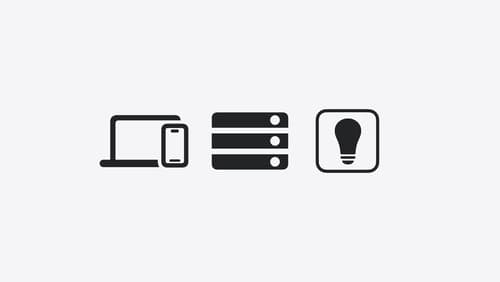
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
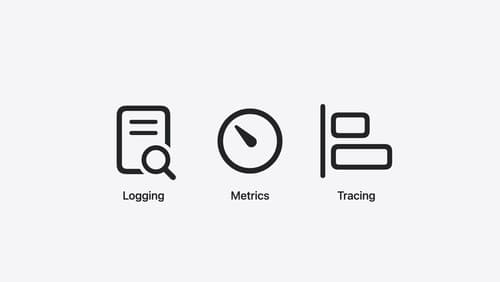
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.
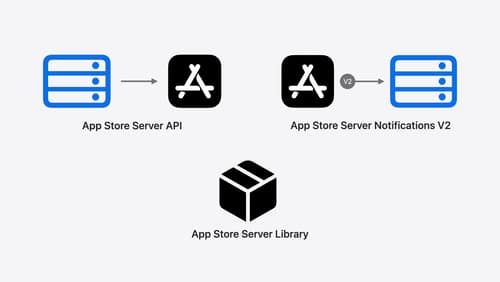
Explore App Store server APIs for In-App Purchase
Learn how to leverage your server to build great In-App Purchase experiences with the latest updates to the App Store Server API, App Store Server Notifications, and the open source App Store Server Library. After a recap of current APIs, we’ll introduce updated endpoint functionality, new transaction fields, and a new notification type. We’ll also discuss best practices for the purchase lifecycle, delivering content, and targeting offers, so you can become a server power user.