How do I traverse a view's subviews in SwiftUI?
Generated on 8/3/2024
1 search
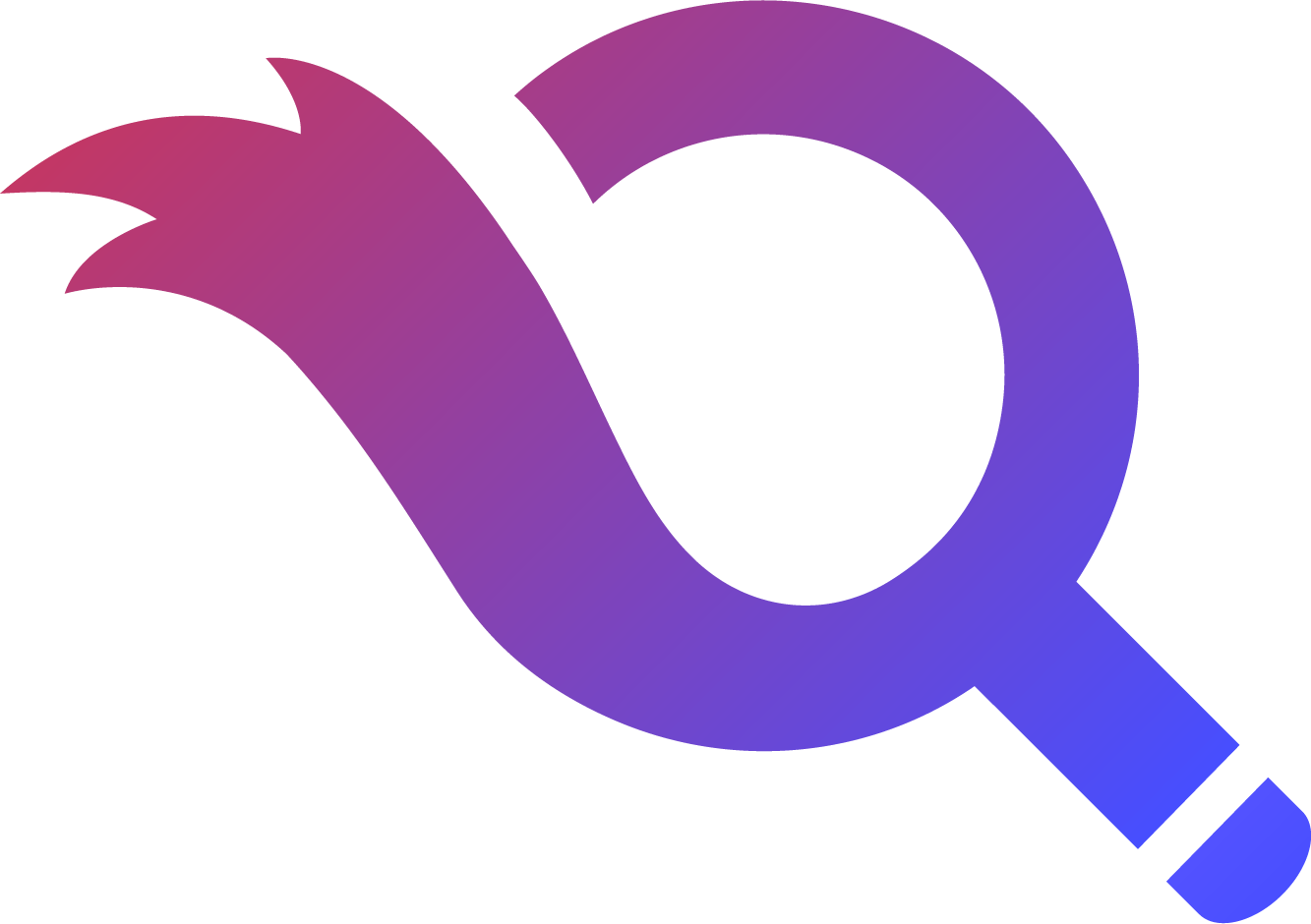
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To traverse a view's subviews in SwiftUI, you can use the new ForEachSubview
API. This API allows you to iterate over the resolved subviews of a given view. Here's a brief explanation of how it works:
-
Declared vs. Resolved Subviews: In SwiftUI, declared subviews are the views you define in your code, while resolved subviews are the actual views that get rendered on the screen. For example, a
ForEach
view is a declared subview that generates multiple resolved subviews based on the data it iterates over. -
Using
ForEachSubview
: TheForEachSubview
API accepts a single view as its input and passes each of its resolved subviews into a trailing view builder. This allows you to transform these subviews into different kinds of views.
Here's a code snippet demonstrating how to use ForEachSubview
:
struct ContentView: View {
var body: some View {
MyCustomContainer {
Text("Subview 1")
Text("Subview 2")
ForEach(0..<3) { index in
Text("Subview \(index + 3)")
}
}
}
}
struct MyCustomContainer<Content: View>: View {
let content: Content
init(@ViewBuilder content: () -> Content) {
self.content = content()
}
var body: some View {
ForEachSubview(of: content) { subview in
// Transform each subview here
subview
.padding()
.background(Color.blue)
}
}
}
In this example, MyCustomContainer
uses ForEachSubview
to iterate over its subviews and apply some transformations.
For more detailed information, you can refer to the session Demystify SwiftUI containers at the 05:03 timestamp.
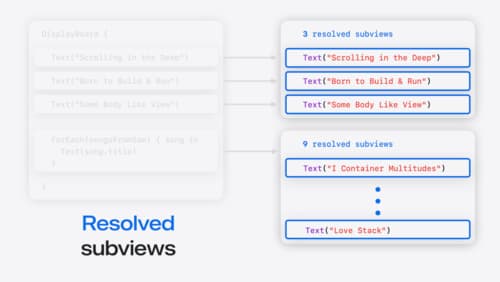
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
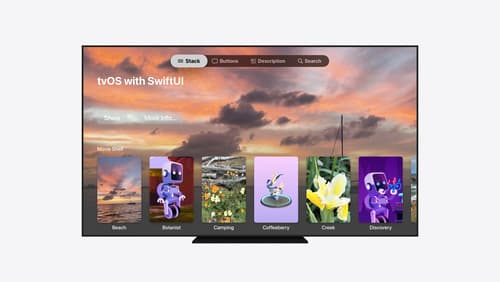
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
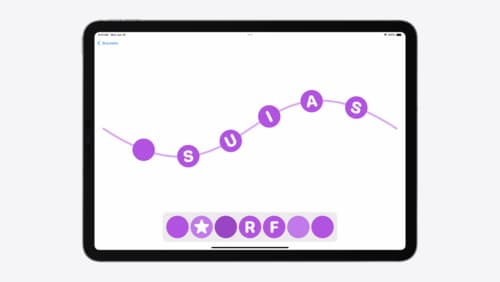
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
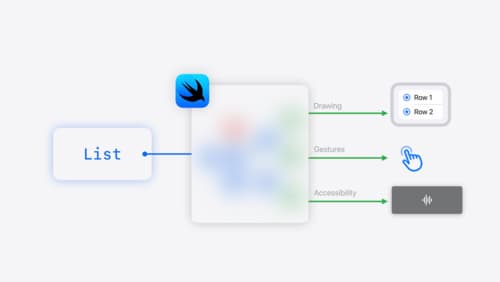
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.