create a view model that will store data in swift data
Generated on 8/3/2024
1 search
To create a view model that will store data using SwiftData, you can follow the steps outlined in the WWDC sessions. Here’s a basic example to get you started:
-
Define Your Model: Use the
@Model
macro to define your data model. -
Create a Model Container: Use the
ModelContainer
to manage your data. -
Create a View Model: Define a view model that interacts with the
ModelContainer
.
Here’s a simple implementation:
Step 1: Define Your Model
import SwiftData
@Model
class Trip {
@Attribute(.unique) var id: UUID
var destination: String
var date: Date
init(destination: String, date: Date) {
self.id = UUID()
self.destination = destination
self.date = date
}
}
Step 2: Create a Model Container
import SwiftData
struct TripsApp: App {
var body: some Scene {
WindowGroup {
ContentView()
.modelContainer(for: Trip.self)
}
}
}
Step 3: Create a View Model
import SwiftUI
import SwiftData
class TripsViewModel: ObservableObject {
@Published var trips: [Trip] = []
private var modelContext: ModelContext
init(modelContext: ModelContext) {
self.modelContext = modelContext
fetchTrips()
}
func fetchTrips() {
let request = Trip.fetchRequest()
if let fetchedTrips = try? modelContext.fetch(request) {
trips = fetchedTrips
}
}
func addTrip(destination: String, date: Date) {
let newTrip = Trip(destination: destination, date: date)
modelContext.insert(newTrip)
try? modelContext.save()
fetchTrips()
}
}
Step 4: Use the View Model in a SwiftUI View
import SwiftUI
struct ContentView: View {
@StateObject private var viewModel: TripsViewModel
init() {
let modelContext = ModelContext(container: ModelContainer(for: Trip.self))
_viewModel = StateObject(wrappedValue: TripsViewModel(modelContext: modelContext))
}
var body: some View {
VStack {
List(viewModel.trips) { trip in
Text(trip.destination)
}
Button(action: {
viewModel.addTrip(destination: "New York", date: Date())
}) {
Text("Add Trip")
}
}
}
}
Relevant Sessions
These sessions provide a comprehensive overview of how to use SwiftData for data persistence and custom data stores.
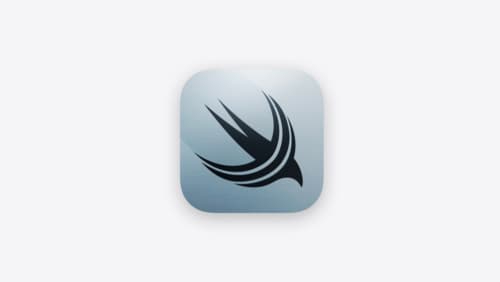
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.
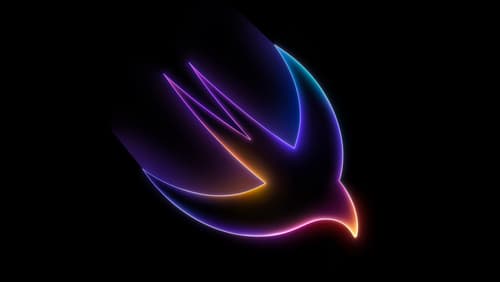
Platforms State of the Union
Discover the newest advancements on Apple platforms.
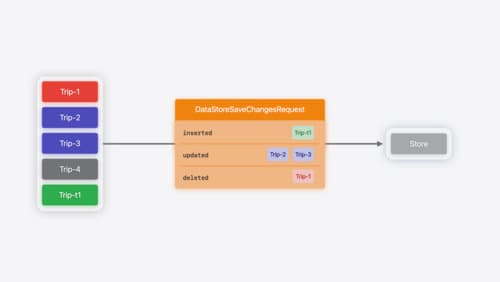
Create a custom data store with SwiftData
Combine the power of SwiftData’s expressive, declarative modeling API with your own persistence backend. Learn how to build a custom data store and explore how to progressively add persistence features in your app. To get the most out of this session, watch “Meet SwiftData” and “Model your schema with SwiftData” from WWDC23.