get user location using async
Generated on 8/4/2024
1 search
To get the user's location using async in Swift, you can leverage the new CoreLocation APIs introduced at WWDC. Specifically, you can use the CLLocationUpdate
and CLMonitor
objects, which present an async sequence interface for getting latitude and longitude updates or state change events.
Here's a simplified example of how you might implement this:
import CoreLocation
func getUserLocation() async throws -> CLLocation {
let locationManager = CLLocationManager()
locationManager.requestWhenInUseAuthorization()
for try await location in locationManager.locationUpdates {
return location
}
throw NSError(domain: "LocationError", code: 1, userInfo: [NSLocalizedDescriptionKey: "Failed to get location"])
}
In this example:
- You create an instance of
CLLocationManager
. - Request location authorization using
requestWhenInUseAuthorization()
. - Use an async sequence to await location updates.
For more details on the new CoreLocation APIs and how to handle location authorization, you can refer to the session What’s new in location authorization (01:47).
Relevant Sessions
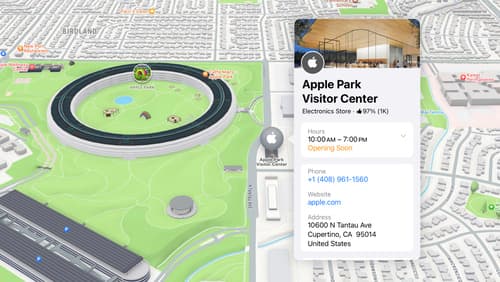
Unlock the power of places with MapKit
Discover powerful new ways to integrate maps into your apps and websites with MapKit and MapKit JS. Learn how to save and reference unique places using Place ID. Check out improvements to search that make it more efficient to find relevant places. Get introduced to the new Place Card API that lets you display rich information about places so customers can explore destinations right in your app. And, we’ll show you quick ways to embed maps in your website with our simplified token provisioning and Web Embed API.
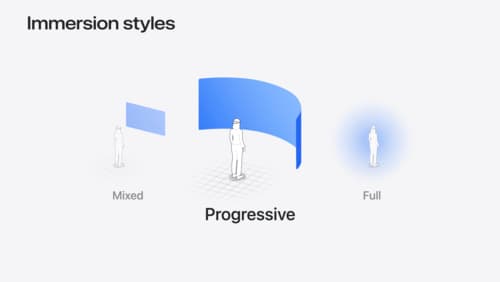
Dive deep into volumes and immersive spaces
Discover powerful new ways to customize volumes and immersive spaces in visionOS. Learn to fine-tune how volumes resize and respond to people moving around them. Make volumes and immersive spaces interact through the power of coordinate conversions. Find out how to make your app react when people adjust immersion with the Digital Crown, and use a surrounding effect to dynamically customize the passthrough tint in your immersive space experience.
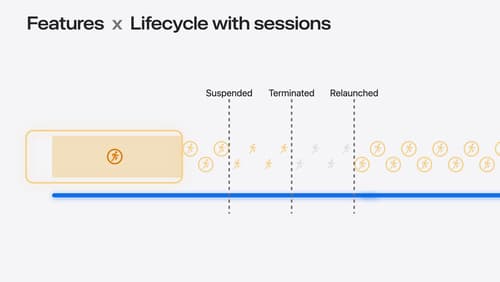
What’s new in location authorization
Location authorization is turning 2.0. Learn about new recommendations and techniques to get the authorization you need, and a new system of diagnostics that can let you know when an authorization goal can’t be met.