How do I use Swift actors?
Generated on 8/4/2024
1 search
To use Swift actors, you can follow these steps:
-
Define an Actor: Actors in Swift are similar to classes but are designed to handle concurrency by serializing access to their state. You can define an actor using the
actor
keyword.actor UserStore { var users: [String] = [] func addUser(_ user: String) { users.append(user) } func getUsers() -> [String] { return users } }
-
Accessing Actor Methods: When you call methods on an actor from outside its context, these calls are asynchronous. You need to use the
await
keyword.let userStore = UserStore() Task { await userStore.addUser("Alice") let users = await userStore.getUsers() print(users) }
-
Concurrency Safety: Actors automatically protect their state by ensuring that only one task can access their state at a time. This helps prevent data races.
actor UserStore { var users: [String] = [] func addUser(_ user: String) { users.append(user) } func getUsers() -> [String] { return users } } let userStore = UserStore() Task { await userStore.addUser("Alice") let users = await userStore.getUsers() print(users) }
-
Using Actors in HTTP Handlers: When using actors in an HTTP request handler, you need to ensure that accesses to the actor are asynchronous.
func handleRequest(username: String) async -> [String] { await userStore.addUser(username) return await userStore.getUsers() }
For more detailed information, you can refer to the session A Swift Tour: Explore Swift’s features and design which covers the basics of writing concurrent code in Swift, including tasks, async/await, and actors.
Relevant Sessions
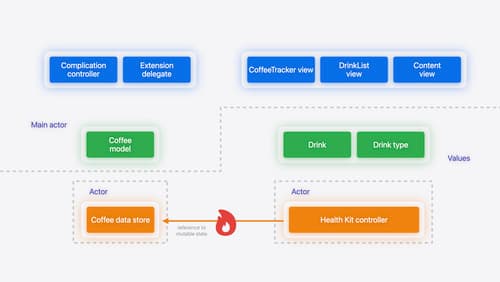
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
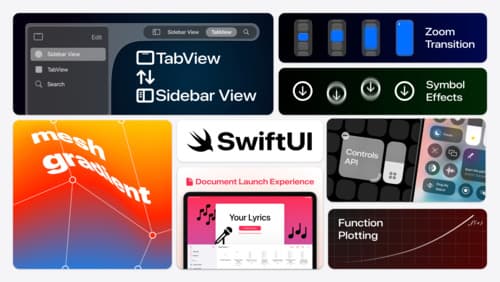
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
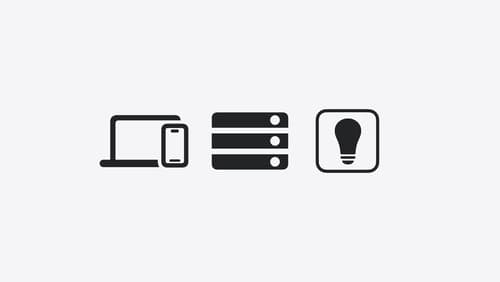
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.