Quick sort algorithm in Swift
Generated on 8/4/2024
1 search
To implement the Quick Sort algorithm in Swift, you can leverage Swift's powerful features such as generics and functional programming. Below is an example of how you might write a Quick Sort function in Swift:
func quickSort<T: Comparable>(_ array: [T]) -> [T] {
guard array.count > 1 else { return array }
let pivot = array[array.count / 2]
let less = array.filter { $0 < pivot }
let equal = array.filter { $0 == pivot }
let greater = array.filter { $0 > pivot }
return quickSort(less) + equal + quickSort(greater)
}
// Example usage:
let unsortedArray = [3, 6, 8, 10, 1, 2, 1]
let sortedArray = quickSort(unsortedArray)
print(sortedArray) // Output: [1, 1, 2, 3, 6, 8, 10]
This implementation uses Swift's filter
function to partition the array into elements less than, equal to, and greater than the pivot. It then recursively sorts the partitions and concatenates them to produce the sorted array.
For more information on Swift's collection algorithms and functional programming features, you might find the session A Swift Tour: Explore Swift’s features and design particularly useful. This session covers Swift's collection types and algorithms, which can help you understand how to use Swift's standard library to write efficient and elegant code.
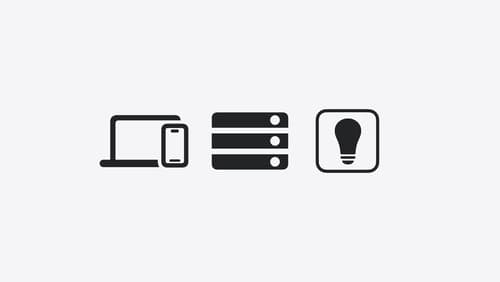
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
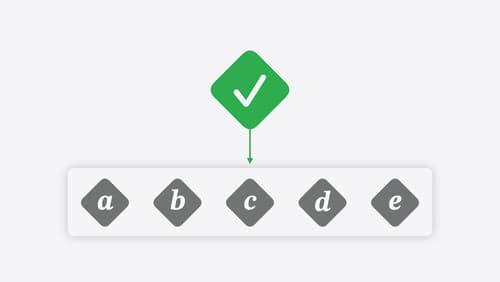
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
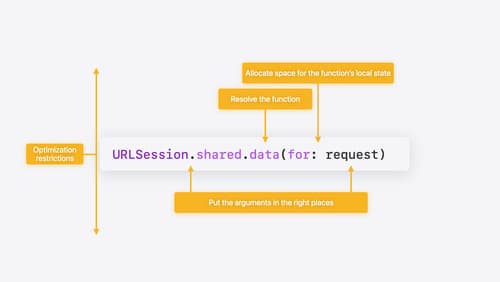
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
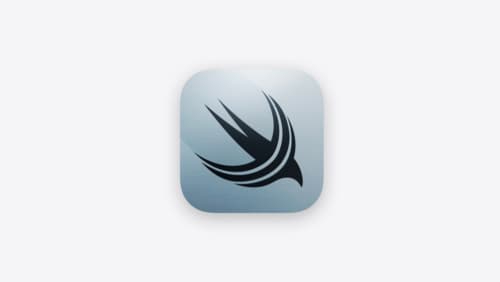
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.