how is memory allocated for closures
Generated on 8/4/2024
1 search
Memory allocation for closures in Swift can be understood by looking at how closures capture and manage their context. Here are the key points:
-
Types of Memory:
- Global Memory: Allocated and initialized when the program is loaded.
- Stack Memory: Used for local variables and call frames, very cheap but limited to certain patterns.
- Heap Memory: Flexible, used for class instances and features without strong static lifetime restrictions, but more expensive to allocate and deallocate.
-
Closure Contexts:
- Non-Escaping Closures: These closures are used within the duration of the call and can capture variables by reference without changing their lifetime. The context can be allocated on the stack.
- Escaping Closures: These closures can outlive the function they were created in, so their context must be heap-allocated and managed with retains and releases. The context behaves like an instance of an anonymous Swift class.
-
Capturing Variables:
- When a closure captures a variable, it does so by reference. If the closure is non-escaping, it captures a pointer to the variable's allocation. If the closure is escaping, the context object must be heap-allocated to ensure the variable's lifetime is extended appropriately.
-
Memory Graph Debugger:
- The memory graph debugger in Xcode can help identify closure contexts and potential reference cycles. Closure contexts are labeled as such in the heap, and strong captures can create reference cycles that need to be managed using weak or unowned references.
For more detailed information, you can refer to the following sessions and chapters:
- Explore Swift performance (Closures)
- Analyze heap memory (Resolving leaks of Swift closure contexts)
These sessions provide a comprehensive overview of how memory is allocated and managed for closures in Swift.
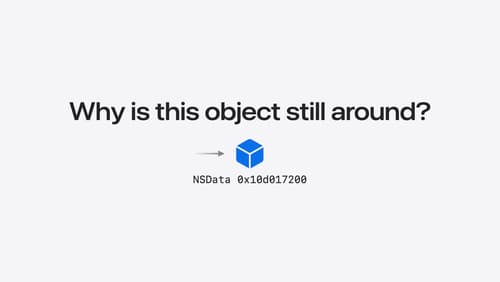
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
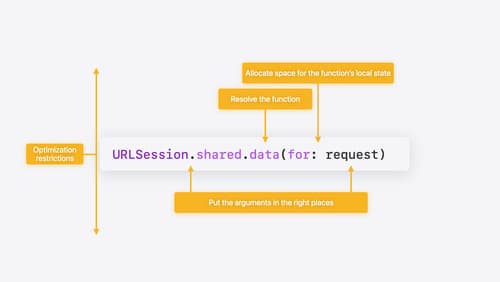
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.