How can I run to concurrency tasks in parallel?
Generated on 8/4/2024
1 search
To run concurrency tasks in parallel in Swift, you can use the concurrency features provided by Swift, such as async
and await
. Here are some key points from the WWDC sessions that can help you:
-
Tasks in Swift: The fundamental unit of concurrency in Swift is a task, which represents an independent concurrent execution context. Tasks are lightweight, so you can create many of them. You can wait for a task to complete to get its result, or you can cancel one if its work becomes unnecessary. Tasks can execute concurrently, making them great for handling multiple operations simultaneously (A Swift Tour: Explore Swift’s features and design).
-
Async/Await Syntax: To model task suspension in code, Swift uses the
async
andawait
syntax. A function that may suspend is marked with theasync
keyword. When an async function is called, theawait
keyword is used to indicate that a suspension can occur on that line. This allows the CPU to be yielded to other tasks that have work to do while waiting for an asynchronous operation to complete (A Swift Tour: Explore Swift’s features and design). -
Parallel Testing: In the context of testing, Swift testing runs test functions in parallel by default, regardless of whether they are synchronous or asynchronous. This is different from XCtest, which only supports parallelization using multiple processes, each running one test at a time. This parallel execution helps reduce execution time and surface hidden dependencies between tests (Go further with Swift Testing).
-
Concurrency in Vision Framework: When working with the Vision framework, you can limit the number of concurrent tasks to manage resources effectively. For example, you might limit the number of tasks to five, adding a new task as soon as one finishes to ensure no more than five requests are processed at a time (Discover Swift enhancements in the Vision framework).
For more detailed information, you can refer to the following sessions:
- A Swift Tour: Explore Swift’s features and design
- Go further with Swift Testing
- Discover Swift enhancements in the Vision framework
These sessions cover the basics of concurrency in Swift, how to use async/await, and practical examples of running tasks in parallel.
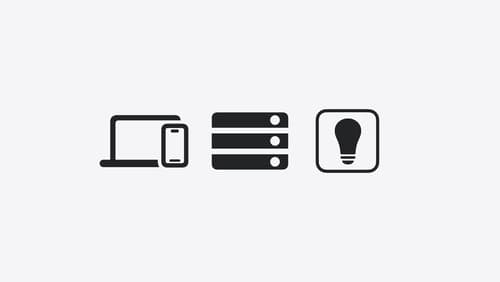
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
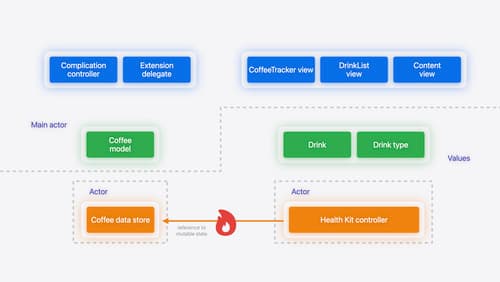
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
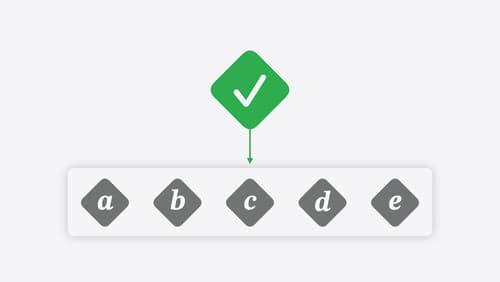
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
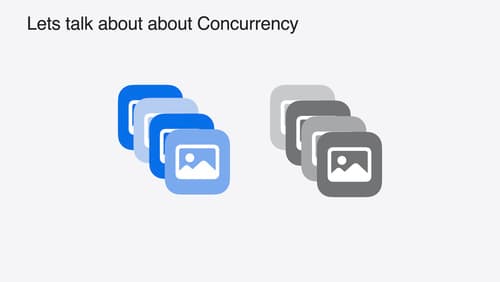
Discover Swift enhancements in the Vision framework
The Vision Framework API has been redesigned to leverage modern Swift features like concurrency, making it easier and faster to integrate a wide array of Vision algorithms into your app. We’ll tour the updated API and share sample code, along with best practices, to help you get the benefits of this framework with less coding effort. We’ll also demonstrate two new features: image aesthetics and holistic body pose.