How do I use sendable?
Asked on 08/04/2024
1 search
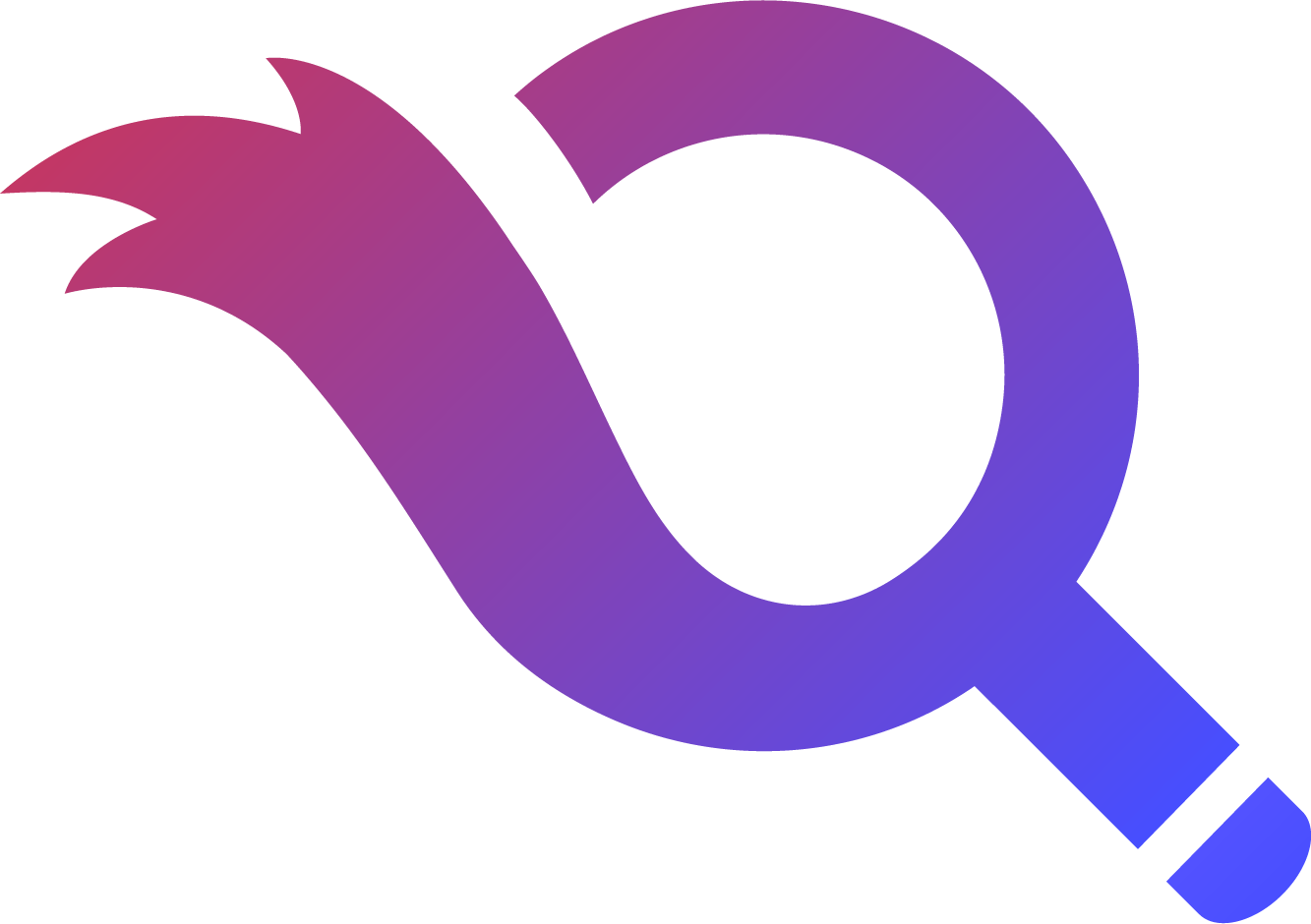
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To use Sendable
in Swift, you need to understand that it is a protocol that ensures a type can be safely transferred between different concurrency domains, such as actors or tasks. Here are the key points on how to use Sendable
:
-
Automatic Conformance for Internal Types: For internal types, Swift automatically considers them
Sendable
if they meet the criteria. This means they do not contain any mutable state that could lead to data races. -
Explicit Conformance for Public Types: For public types, you need to explicitly mark them as
Sendable
. This is because marking a type asSendable
is a guarantee to your clients that the type contains no mutable state that could lead to data races. You can do this by adding theSendable
protocol conformance to your type.public struct MyType: Sendable { // Your properties and methods here }
-
Handling Warnings: When you mark a type as
Sendable
, it can eliminate multiple warnings related to data race safety. This is particularly useful in large projects where multiple parts of the codebase might require the type to beSendable
. -
Dealing with Non-Sendable Types: If you encounter a type that cannot be marked as
Sendable
(e.g., an Objective-C type that stores mutable state), you need to make decisions about safety. One option is to reason about the type and ensure it is safe to use in a concurrent context, even if it is notSendable
.
For a practical example and more detailed explanation, you can refer to the session Migrate your app to Swift 6 (32:27).
Relevant Sessions
These sessions provide a comprehensive overview of how to work with Sendable
and ensure data race safety in your Swift applications.
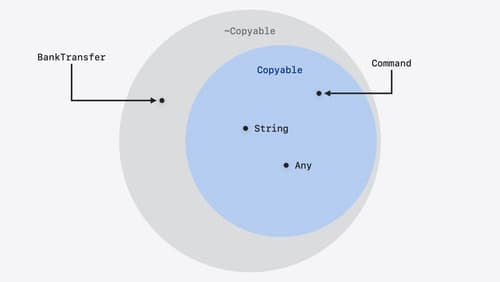
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
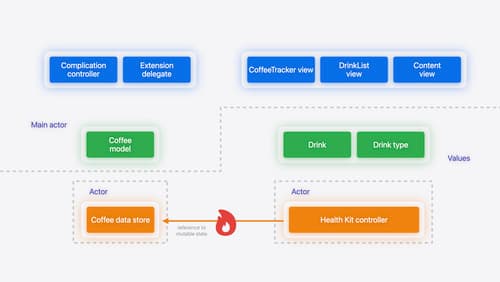
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
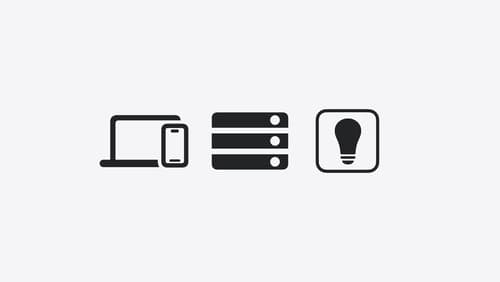
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
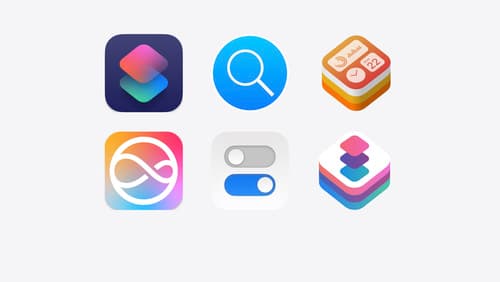
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.