Show a page refresh in swiftui
Generated on 8/4/2024
1 search
To implement a page refresh in SwiftUI, you can use the refreshable
modifier, which was introduced in iOS 15. This modifier allows you to add pull-to-refresh functionality to your views, such as List
or ScrollView
.
Here's a basic example of how to use refreshable
in a SwiftUI view:
import SwiftUI
struct ContentView: View {
@State private var items = ["Item 1", "Item 2", "Item 3"]
var body: some View {
NavigationView {
List(items, id: \.self) { item in
Text(item)
}
.refreshable {
await fetchData()
}
.navigationTitle("Pull to Refresh")
}
}
func fetchData() async {
// Simulate a network call
try? await Task.sleep(nanoseconds: 2 * 1_000_000_000)
items.append("New Item")
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In this example:
- The
List
displays a list of items. - The
refreshable
modifier is added to theList
, which calls thefetchData
function when the user pulls down to refresh. - The
fetchData
function simulates a network call by sleeping for 2 seconds and then adds a new item to the list.
For more detailed information on state-driven views and animations in SwiftUI, you can refer to the SwiftUI essentials session from WWDC 2024.
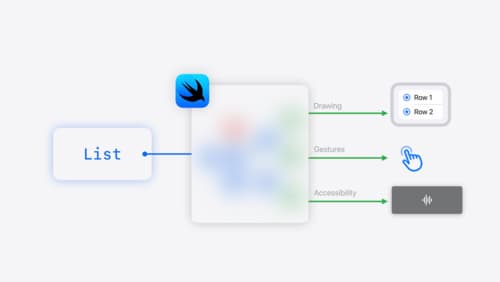
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
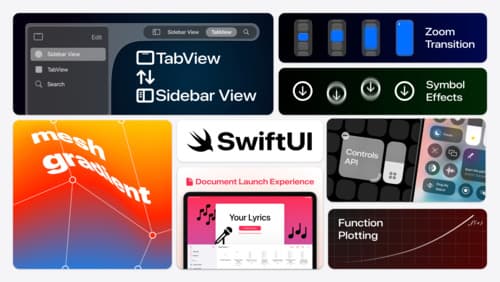
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
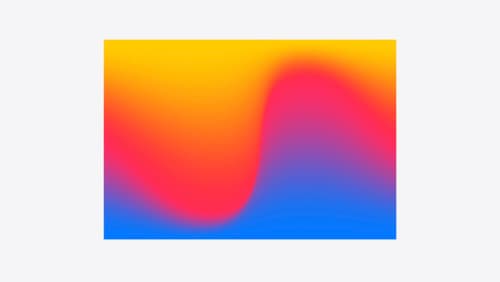
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
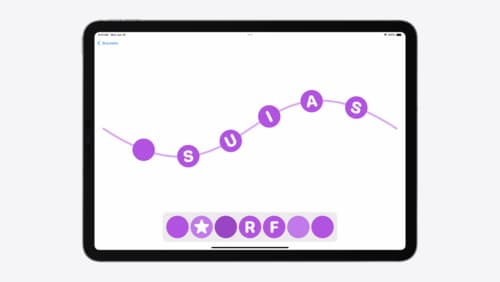
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.