How can I parse Package.swift using swift-syntax?
Asked on 08/05/2024
1 search
To parse Package.swift
using swift-syntax
, you can follow these general steps:
-
Set Up Your Project: Ensure you have a Swift project where you can add dependencies.
-
Add
swift-syntax
Dependency: Includeswift-syntax
in yourPackage.swift
file. Here’s an example of how to add it:// swift-tools-version:5.5 import PackageDescription let package = Package( name: "YourProjectName", dependencies: [ .package(url: "https://github.com/apple/swift-syntax.git", from: "0.50500.0"), ], targets: [ .target( name: "YourProjectName", dependencies: [ .product(name: "SwiftSyntax", package: "swift-syntax"), ]), ] )
-
Parse
Package.swift
: Useswift-syntax
to parse thePackage.swift
file. Here’s a basic example of how you might do this:import SwiftSyntax let sourceFile = try SyntaxParser.parse(URL(fileURLWithPath: "path/to/Package.swift")) // Traverse the syntax tree class Visitor: SyntaxVisitor { override func visit(_ node: FunctionCallExprSyntax) -> SyntaxVisitorContinueKind { print("Found function call: \(node)") return .visitChildren } } let visitor = Visitor() sourceFile.walk(visitor)
For more detailed information on how to use swift-syntax
, you can refer to the official documentation or explore examples provided in the Swift community.
If you want to learn more about Swift packages and their organization, you might find the session A Swift Tour: Explore Swift’s features and design helpful, especially the chapter on "Code organization" starting at 09:48.
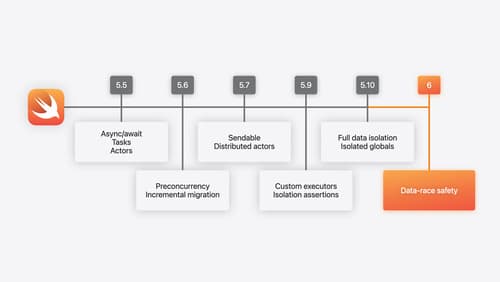
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
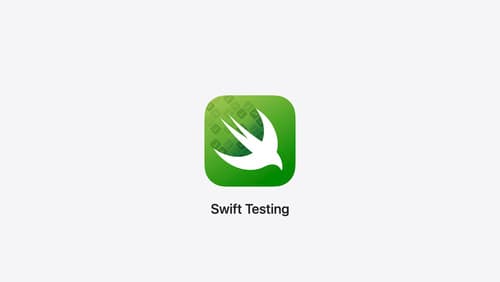
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
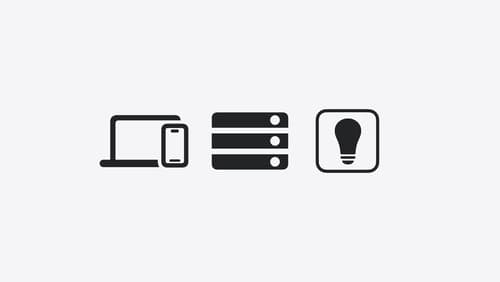
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.