how to use swiftui in uikit
Generated on 8/5/2024
1 search
To use SwiftUI in UIKit, you can leverage the interoperability features provided by SwiftUI. Here are the key points and steps to achieve this:
-
Embedding UIKit Views in SwiftUI:
- You can use
UIViewRepresentable
to wrap a UIKit view and use it within SwiftUI. This protocol allows you to create and update a UIKit view using imperative code. - Example:
import SwiftUI import UIKit struct MyUIKitView: UIViewRepresentable { func makeUIView(context: Context) -> UIView { let view = UIView() // Configure your UIKit view here return view } func updateUIView(_ uiView: UIView, context: Context) { // Update the view with new data } }
- You can use
-
Embedding SwiftUI Views in UIKit:
- You can use
UIHostingController
to embed a SwiftUI view in a UIKit view hierarchy. This is useful when you want to integrate SwiftUI views into an existing UIKit-based app. - Example:
import SwiftUI import UIKit class MyViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let swiftUIView = MySwiftUIView() let hostingController = UIHostingController(rootView: swiftUIView) addChild(hostingController) view.addSubview(hostingController.view) hostingController.didMove(toParent: self) // Set constraints or frame for hostingController.view } }
- You can use
-
Animations:
- You can set up animations on UIKit views and drive them with SwiftUI, including fully custom animations. This allows you to use the full suite of SwiftUI animation types to animate UIKit views.
- Example:
import SwiftUI import UIKit struct ContentView: View { @State private var animate = false var body: some View { VStack { MyUIKitViewWrapper() .frame(width: 100, height: 100) .background(animate ? Color.red : Color.blue) .animation(.easeInOut(duration: 1), value: animate) Button("Animate") { animate.toggle() } } } } struct MyUIKitViewWrapper: UIViewRepresentable { func makeUIView(context: Context) -> UIView { let view = UIView() // Configure your UIKit view here return view } func updateUIView(_ uiView: UIView, context: Context) { // Update the view with new data } }
For more detailed information, you can refer to the following sessions from WWDC 2024:
- SwiftUI essentials (20:25)
- Enhance your UI animations and transitions (08:34)
These sessions cover the fundamentals of integrating SwiftUI with UIKit and provide practical examples and tips.
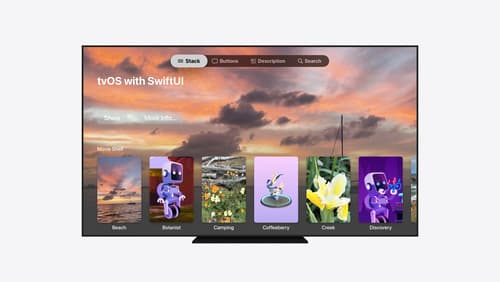
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
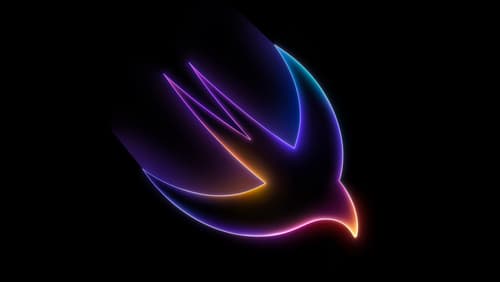
Platforms State of the Union
Discover the newest advancements on Apple platforms.
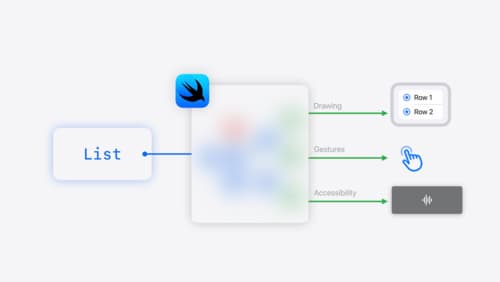
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
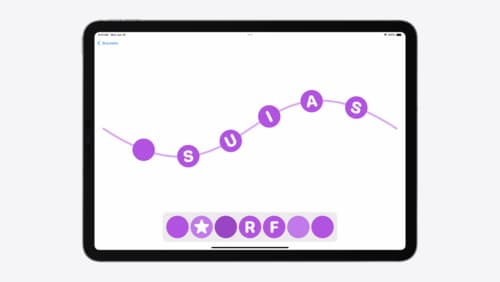
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.