how can i use UIStackView to display multi views in UIScrollView
Asked on 08/05/2024
1 search
To use UIStackView
to display multiple views within a UIScrollView
, you can follow these steps:
- Create a
UIScrollView
: This will be the container that allows scrolling. - Add a
UIStackView
to theUIScrollView
: The stack view will manage the layout of its subviews. - Configure the
UIStackView
: Set its axis, distribution, alignment, and spacing properties as needed. - Add your views to the
UIStackView
: These can be any views you want to display.
Here's a basic example in Swift:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Create a UIScrollView
let scrollView = UIScrollView()
scrollView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(scrollView)
// Create a UIStackView
let stackView = UIStackView()
stackView.axis = .vertical
stackView.distribution = .fill
stackView.alignment = .fill
stackView.spacing = 10
stackView.translatesAutoresizingMaskIntoConstraints = false
scrollView.addSubview(stackView)
// Add constraints to scrollView
NSLayoutConstraint.activate([
scrollView.leadingAnchor.constraint(equalTo: view.leadingAnchor),
scrollView.trailingAnchor.constraint(equalTo: view.trailingAnchor),
scrollView.topAnchor.constraint(equalTo: view.topAnchor),
scrollView.bottomAnchor.constraint(equalTo: view.bottomAnchor)
])
// Add constraints to stackView
NSLayoutConstraint.activate([
stackView.leadingAnchor.constraint(equalTo: scrollView.leadingAnchor),
stackView.trailingAnchor.constraint(equalTo: scrollView.trailingAnchor),
stackView.topAnchor.constraint(equalTo: scrollView.topAnchor),
stackView.bottomAnchor.constraint(equalTo: scrollView.bottomAnchor),
stackView.widthAnchor.constraint(equalTo: scrollView.widthAnchor)
])
// Add views to the stackView
for i in 1...10 {
let label = UILabel()
label.text = "Label \(i)"
label.backgroundColor = .lightGray
label.textAlignment = .center
stackView.addArrangedSubview(label)
}
}
}
Relevant Sessions
- Get started with Dynamic Type (06:21): This session discusses using
UIStackView
for dynamic layouts in UIKit, which is relevant to your question.
For more detailed information, you can refer to the session Get started with Dynamic Type.
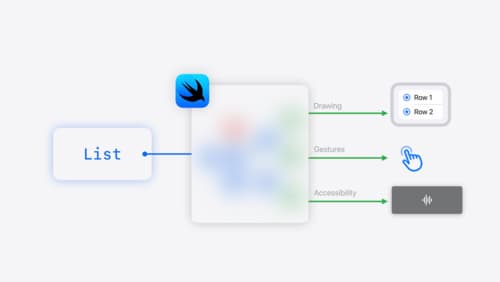
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
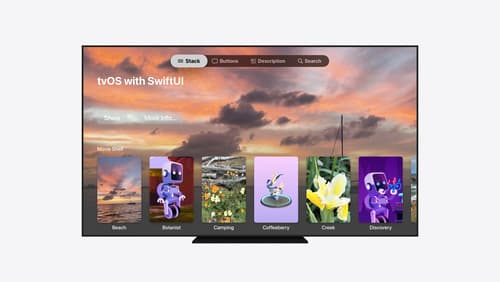
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
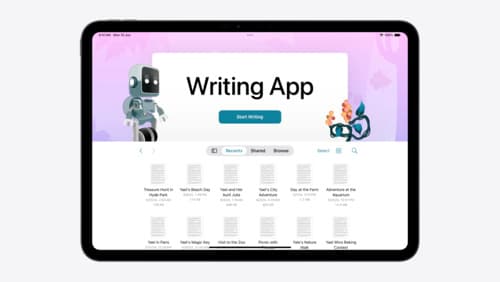
Evolve your document launch experience
Make your document-based app stand out, and bring its unique identity into focus with the new document launch experience. Learn how to leverage the new API to customize the first screen people see when they launch your app. Utilize the new system-provided design, and amend it with custom actions, delightful decorative views, and impressive animations.