How to get movie file from ReplayKit?
Asked on 2024-07-30
1 search
To get a movie file from ReplayKit, you can use the new recording API provided by ScreenCaptureKit. Here’s a step-by-step guide based on the information from the WWDC sessions:
-
Create an SC Recording Output Configuration:
- Set an output URL to specify where to save the file.
- Optionally, customize the file type and video codec for the saved file.
-
Create an SC Recording Output Instance:
- Use the recording configuration created in the previous step.
- Set the delegate for receiving events.
-
Add the Configured Recording Output to the Stream:
- Call
startCapture
to start the stream, which will also start recording. - To stop recording, call
stopCapture
. If you want to continue streaming after stopping the recording, callremoveRecordingOutput
instead.
- Call
-
Handle Recording Events:
- Use the SC Recording Output Delegate to be notified when the recording starts, if an error occurs, and when the recording has successfully finished.
Here is a code snippet to illustrate these steps:
// Step 1: Create an SC Recording Output Configuration
let recordingConfig = SCRecordingOutputConfiguration()
recordingConfig.outputURL = URL(fileURLWithPath: "path/to/save/file")
recordingConfig.fileType = .mov // Optional
recordingConfig.videoCodec = .h264 // Optional
// Step 2: Create an SC Recording Output Instance
let recordingOutput = SCRecordingOutput(configuration: recordingConfig)
recordingOutput.delegate = self // Set your delegate
// Step 3: Add the Configured Recording Output to the Stream
stream.addOutput(recordingOutput)
stream.startCapture()
// To stop recording
stream.stopCapture()
// To continue streaming after stopping the recording
stream.removeOutput(recordingOutput)
For more detailed information, you can refer to the session Capture HDR content with ScreenCaptureKit at the timestamp 08:41.
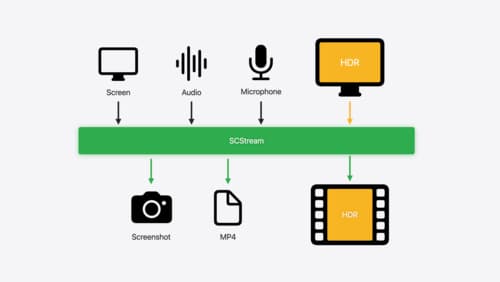
Capture HDR content with ScreenCaptureKit
Learn how to capture high dynamic colors using ScreenCaptureKit, and explore new features like HDR support, microphone capture, and straight-to-file recording.
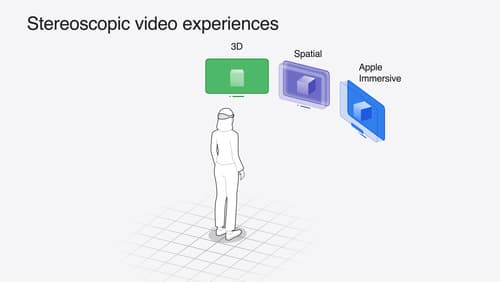
Build compelling spatial photo and video experiences
Learn how to adopt spatial photos and videos in your apps. Explore the different types of stereoscopic media and find out how to capture spatial videos in your iOS app on iPhone 15 Pro. Discover the various ways to detect and present spatial media, including the new QuickLook Preview Application API in visionOS. And take a deep dive into the metadata and stereo concepts that make a photo or video spatial.