how to display a dialog in swiftui
Generated on 8/5/2024
1 search
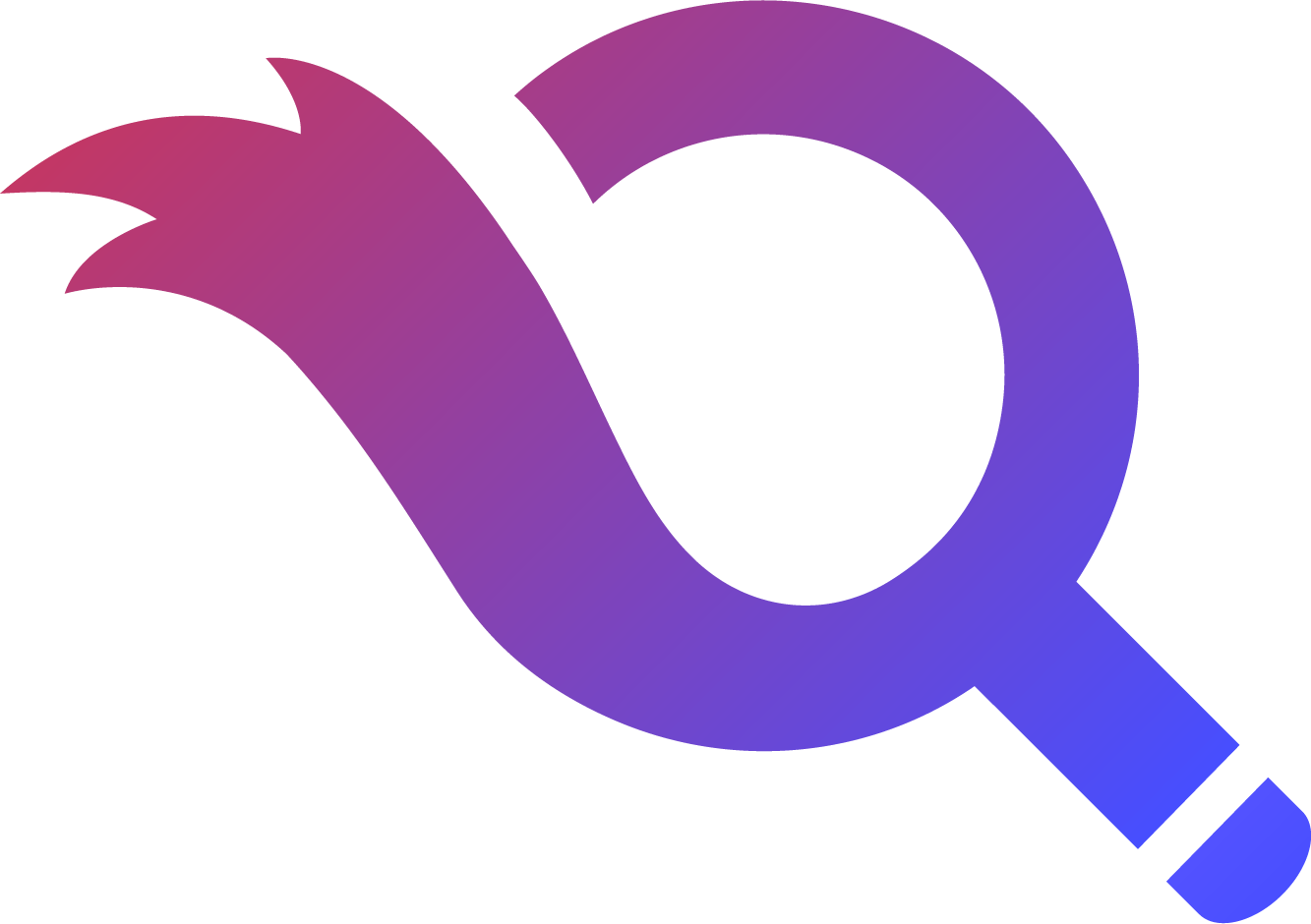
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To display a dialog in SwiftUI, you can use the alert
or sheet
modifiers, depending on the type of dialog you want to present. Here are some examples:
Using alert
The alert
modifier is used to present an alert dialog with a title, message, and buttons.
struct ContentView: View {
@State private var showAlert = false
var body: some View {
Button("Show Alert") {
showAlert = true
}
.alert("Alert Title", isPresented: $showAlert) {
Button("OK", role: .cancel) { }
} message: {
Text("This is an alert message.")
}
}
}
Using sheet
The sheet
modifier is used to present a modal view.
struct ContentView: View {
@State private var showSheet = false
var body: some View {
Button("Show Sheet") {
showSheet = true
}
.sheet(isPresented: $showSheet) {
SheetView()
}
}
}
struct SheetView: View {
var body: some View {
Text("This is a sheet.")
}
}
Using confirmationDialog
For more complex dialogs, you can use confirmationDialog
.
struct ContentView: View {
@State private var showDialog = false
var body: some View {
Button("Show Dialog") {
showDialog = true
}
.confirmationDialog("Dialog Title", isPresented: $showDialog) {
Button("Option 1") { }
Button("Option 2") { }
Button("Cancel", role: .cancel) { }
} message: {
Text("This is a confirmation dialog.")
}
}
}
For more advanced usage and examples, you can refer to the session What’s new in SwiftUI from WWDC 2024.
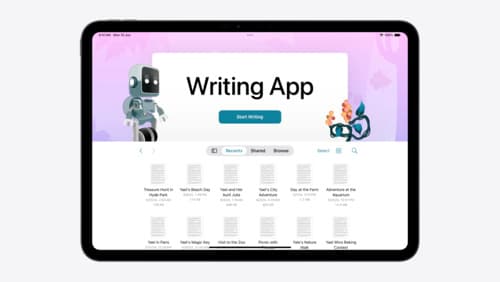
Evolve your document launch experience
Make your document-based app stand out, and bring its unique identity into focus with the new document launch experience. Learn how to leverage the new API to customize the first screen people see when they launch your app. Utilize the new system-provided design, and amend it with custom actions, delightful decorative views, and impressive animations.
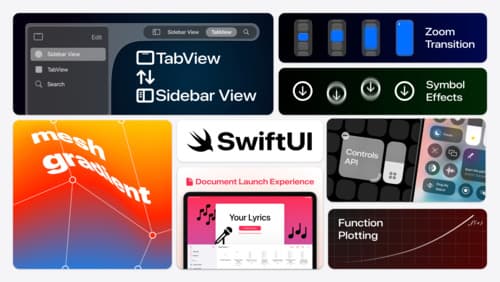
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
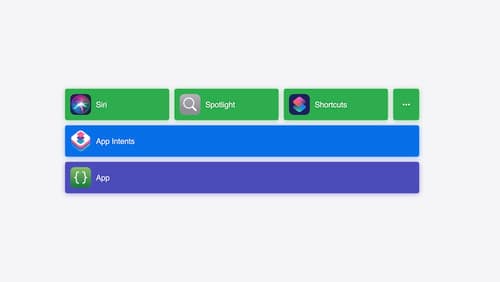
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.
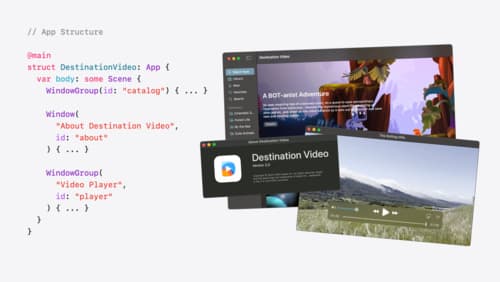
Tailor macOS windows with SwiftUI
Make your windows feel tailor-made for macOS. Fine-tune your app’s windows for focused purposes, ease of use, and to express functionality. Use SwiftUI to style window toolbars and backgrounds. Arrange your windows with precision, and make smart decisions about restoration and minimization.
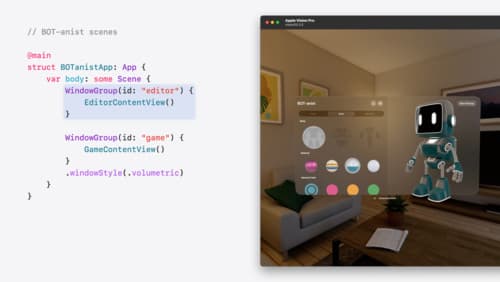
Work with windows in SwiftUI
Learn how to create great single and multi-window apps in visionOS, macOS, and iPadOS. Discover tools that let you programmatically open and close windows, adjust position and size, and even replace one window with another. We’ll also explore design principles for windows that help people use your app within their workflows.
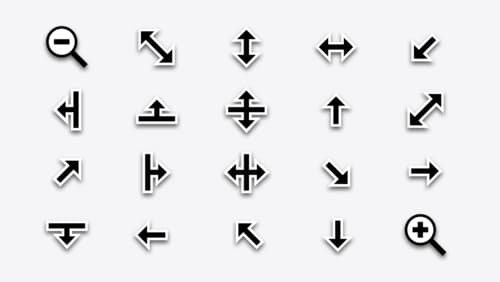
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
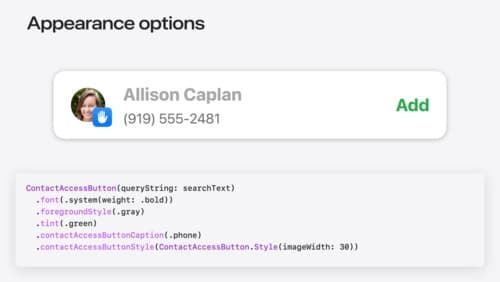
Meet the Contact Access Button
Learn about the new Contacts authorization modes and how to improve Contacts access in your app. Discover how to integrate the Contact Access Button into your app to share additional contacts on demand and provide an easier path to Contacts authorization. We’ll also cover Contacts security features and an alternative API to be used if the button isn’t appropriate for your app.
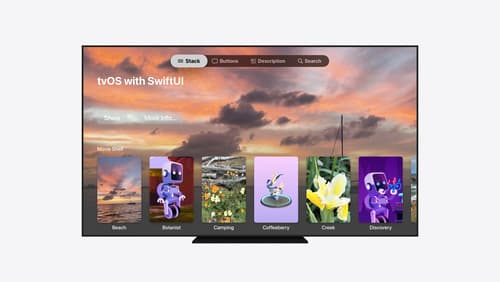
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.