Implementing a Collection
Generated on 8/5/2024
1 search
To implement a collection in Swift, you can leverage the power of generics and protocols. Here's a brief overview based on the content from the WWDC sessions:
-
Using Generics and Protocols:
- Swift's collection types, such as arrays, dictionaries, and sets, can be extended using generics. For example, you can add a method to remove duplicates from any collection that contains elements conforming to the
Hashable
protocol. This is demonstrated in the session A Swift Tour: Explore Swift’s features and design.
- Swift's collection types, such as arrays, dictionaries, and sets, can be extended using generics. For example, you can add a method to remove duplicates from any collection that contains elements conforming to the
-
Collection Types:
- Swift provides various collection types, including arrays, dictionaries, and sets. Each of these types conforms to the
Collection
protocol, which allows for common operations like iteration and indexing. This is covered in the session A Swift Tour: Explore Swift’s features and design.
- Swift provides various collection types, including arrays, dictionaries, and sets. Each of these types conforms to the
-
Performance Considerations:
- When working with collections, it's important to understand the performance implications of different operations. For example, the way Swift handles memory allocation and value copying can impact the performance of your collections. This is discussed in the session Explore Swift performance.
-
Custom Containers in SwiftUI:
- If you're working with SwiftUI, you can create custom containers using new APIs like container values. These allow for flexible composition and customization of your views. This is detailed in the session Demystify SwiftUI containers.
Relevant Sessions
- A Swift Tour: Explore Swift’s features and design
- Explore Swift performance
- Demystify SwiftUI containers
If you need more specific details or examples, feel free to ask!
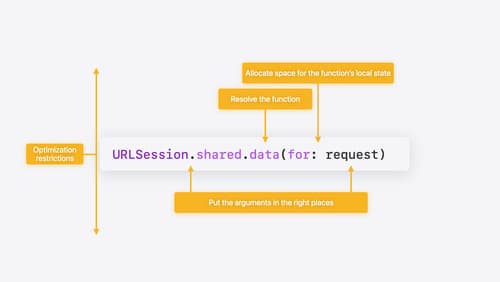
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
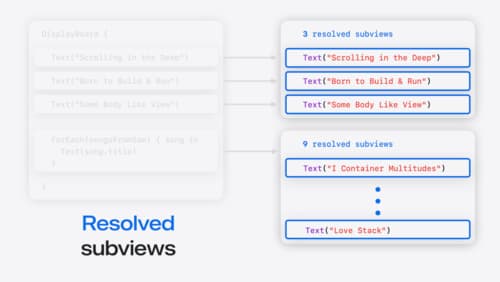
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
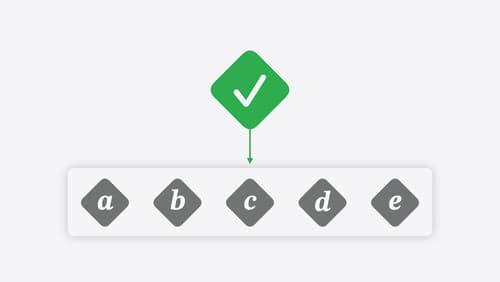
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
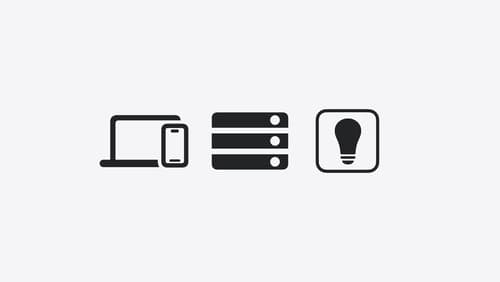
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.