how to use conditional compiling in SPM modules
Asked on 2024-08-05
1 search
To use conditional compiling in Swift Package Manager (SPM) modules, you can leverage build settings and feature macros to control which parts of your code are compiled under different conditions. This is particularly useful when you need to support multiple configurations or environments.
Here are the steps to achieve this:
-
Define Conditional Compilation Flags: You can define custom flags in your
Package.swift
file or in Xcode build settings. These flags can be used to conditionally include or exclude code. -
Use
#if
Directives in Code: In your Swift code, use#if
,#elseif
, and#else
directives to conditionally compile code based on the flags you defined.
Example
Step 1: Define Flags in Package.swift
// swift-tools-version:5.7
import PackageDescription
let package = Package(
name: "MyPackage",
targets: [
.target(
name: "MyTarget",
swiftSettings: [
.define("FEATURE_A"),
.define("FEATURE_B", .when(configuration: .release))
]
)
]
)
Step 2: Use #if
Directives in Your Code
public struct MyFeature {
public init() {}
public func performAction() {
#if FEATURE_A
print("Feature A is enabled")
#elseif FEATURE_B
print("Feature B is enabled")
#else
print("No feature is enabled")
#endif
}
}
Additional Resources
For more detailed information on how to manage and optimize your builds, you can refer to the session Demystify explicitly built modules from WWDC 2024. This session covers how to handle different module variants and optimize build settings to reduce unnecessary work.
Relevant Sessions
- Demystify explicitly built modules
- What’s new in Xcode 16
- A Swift Tour: Explore Swift’s features and design
These sessions provide insights into module management, build optimizations, and the latest updates in Xcode that can help you with conditional compiling in SPM modules.
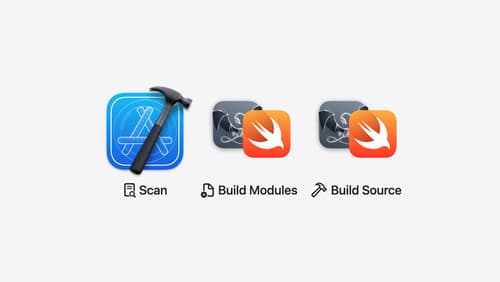
Demystify explicitly built modules
Explore how builds are changing in Xcode 16 with explicitly built modules. Discover how modules are used to build your code, how explicitly built modules improve transparency in compilation tasks, and how you can optimize your build by sharing modules across targets.
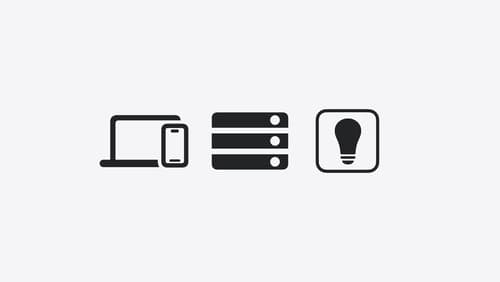
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
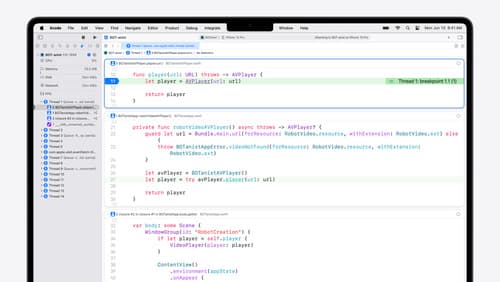
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.