How do I record spatial videos with iOS 18?
Generated on 8/5/2024
1 search
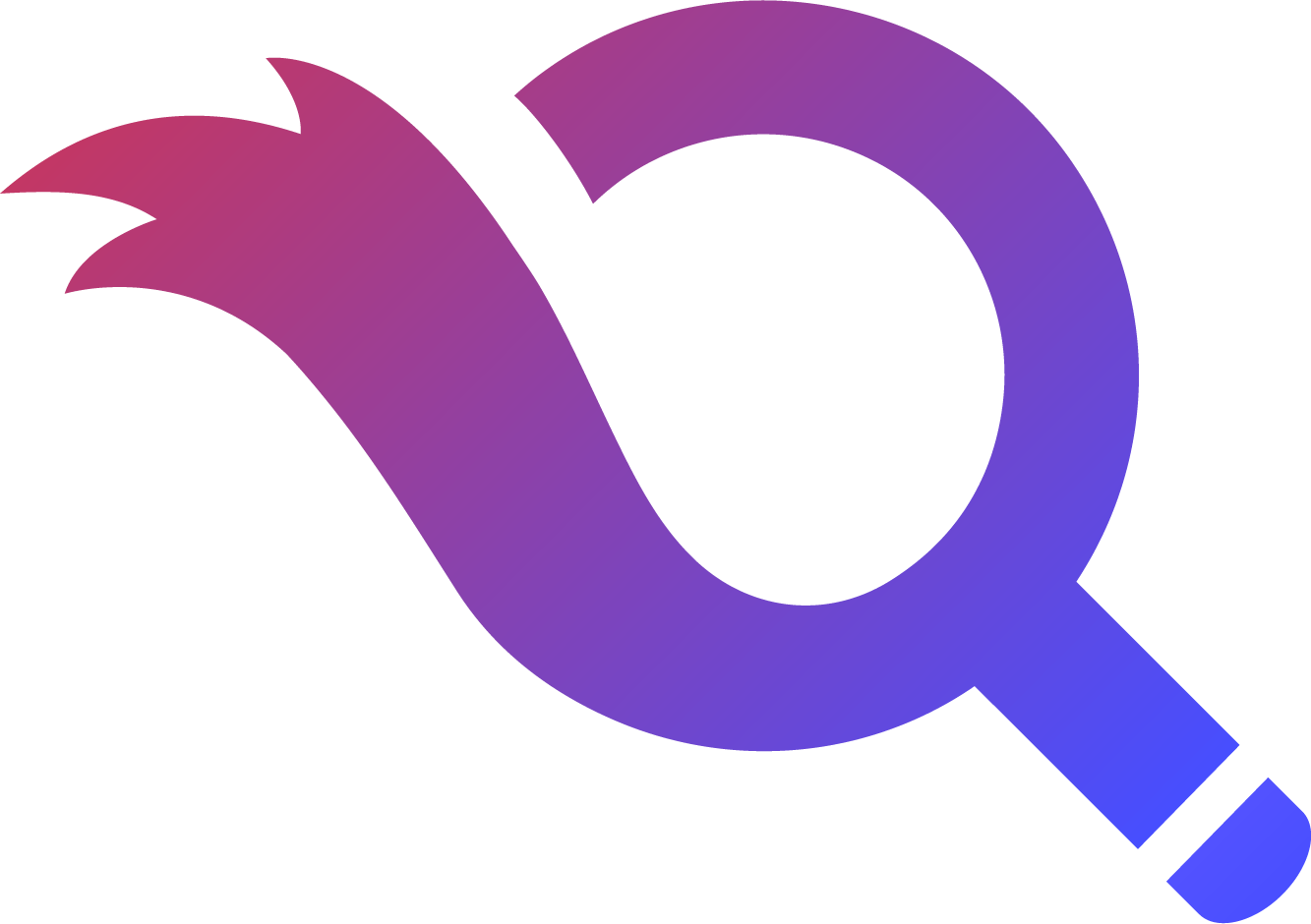
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To record spatial videos with iOS 18, you can follow these steps using the AV Foundation framework:
- Change the AV Capture Device: Use the built-in dual wide camera, as spatial video requires both the wide and ultra-wide cameras to be streaming simultaneously.
- Pick a Supported Video Format: Iterate through the formats in the video device and check if
isSpatialVideoCaptureSupported
is true. When you find a format that supports spatial video, make it the active format on the video device. Ensure to lock and unlock the video device appropriately and add error handling to return false if no suitable format is found. - Enable Spatial Video Recording: Check that spatial video capture is supported and set
isSpatialVideoCaptureEnabled
to true. Note that this feature only works on iPhone 15 Pro.
Here is a brief code outline to achieve this:
let captureSession = AVCaptureSession()
guard let videoDevice = AVCaptureDevice.default(.builtInDualWideCamera, for: .video, position: .back) else {
return
}
do {
try videoDevice.lockForConfiguration()
defer { videoDevice.unlockForConfiguration() }
for format in videoDevice.formats {
if format.isSpatialVideoCaptureSupported {
videoDevice.activeFormat = format
break
}
}
if !videoDevice.isSpatialVideoCaptureSupported {
return
}
videoDevice.isSpatialVideoCaptureEnabled = true
} catch {
print("Error configuring video device: \(error)")
return
}
let videoInput = try AVCaptureDeviceInput(device: videoDevice)
captureSession.addInput(videoInput)
let movieOutput = AVCaptureMovieFileOutput()
captureSession.addOutput(movieOutput)
captureSession.startRunning()
For more details, you can refer to the session Build compelling spatial photo and video experiences.