create me a list view with images in swiftui
Generated on 8/5/2024
1 search
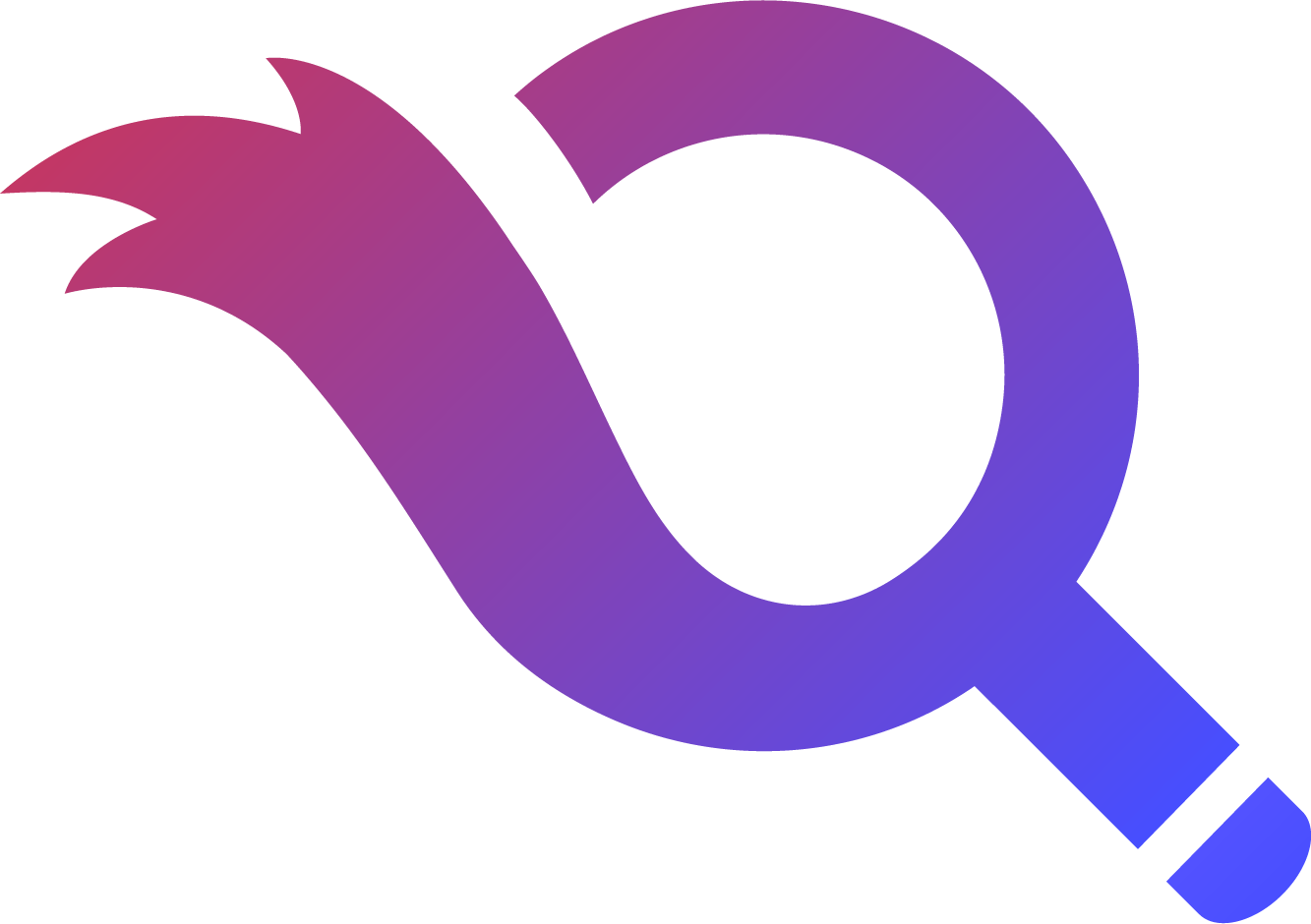
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To create a list view with images in SwiftUI, you can follow the example provided in the "SwiftUI essentials" session. Here's a basic implementation:
import SwiftUI
struct Pet: Identifiable {
let id = UUID()
let name: String
let imageName: String
}
struct ContentView: View {
let pets = [
Pet(name: "Whiskers", imageName: "whiskers"),
Pet(name: "Rufus", imageName: "rufus"),
Pet(name: "Bubbles", imageName: "bubbles")
]
var body: some View {
List(pets) { pet in
HStack {
Image(pet.imageName)
.resizable()
.frame(width: 50, height: 50)
.clipShape(Circle())
Text(pet.name)
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Explanation:
- Model: Define a
Pet
struct that conforms to theIdentifiable
protocol. - Data: Create an array of
Pet
instances. - View: Use a
List
to display each pet. Inside theList
, use anHStack
to arrange the image and text horizontally. - Image: Use the
Image
view to display the pet's image, making it resizable and clipping it to a circle.
This example leverages the declarative nature of SwiftUI to create a list view with images and text. For more advanced customizations, you can use view modifiers and other SwiftUI features.
For more details, you can refer to the SwiftUI essentials session.
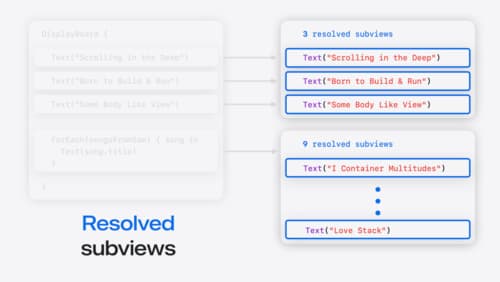
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
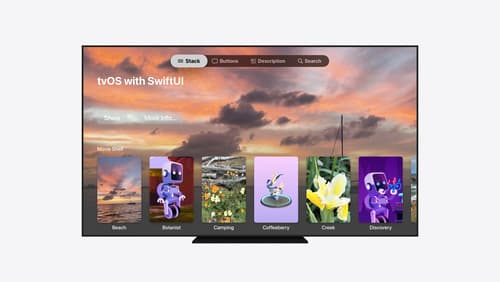
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
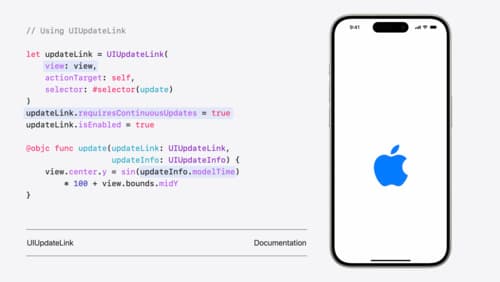
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.
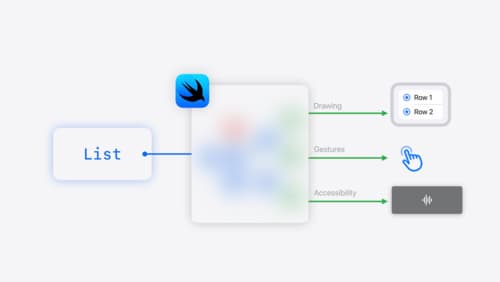
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.