how can I do a color conversion with vimage in the accelerate framework?
Generated on 8/5/2024
1 search
To perform color conversion with vImage in the Accelerate framework, you can follow these steps:
-
Import the Accelerate framework:
import Accelerate
-
Set up the source and destination buffers:
var sourceBuffer = vImage_Buffer() var destinationBuffer = vImage_Buffer()
-
Initialize the source buffer with your image data:
// Assuming you have a UIImage named `sourceImage` let cgImage = sourceImage.cgImage! let format = vImage_CGImageFormat(cgImage: cgImage) vImageBuffer_InitWithCGImage(&sourceBuffer, &format, nil, cgImage, vImage_Flags(kvImageNoFlags))
-
Initialize the destination buffer:
vImageBuffer_Init(&destinationBuffer, sourceBuffer.height, sourceBuffer.width, format.bitsPerPixel, vImage_Flags(kvImageNoFlags))
-
Perform the color conversion:
let error = vImageConvert_RGB888toARGB8888(&sourceBuffer, nil, 0, &destinationBuffer, vImage_Flags(kvImageNoFlags)) if error != kvImageNoError { print("Error in color conversion: \(error)") }
-
Create a CGImage from the destination buffer:
let resultCGImage = vImageCreateCGImageFromBuffer(&destinationBuffer, &format, nil, nil, vImage_Flags(kvImageNoFlags), nil)?.takeRetainedValue()
-
Convert the CGImage to a UIImage:
let resultImage = UIImage(cgImage: resultCGImage!)
This example demonstrates converting an RGB image to an ARGB image. You can adjust the conversion function and parameters based on your specific needs.
For more detailed information on color conversion and other image processing tasks using vImage, you can refer to the Accelerate framework documentation.
Relevant Sessions
- Keep colors consistent across captures (WWDC 2024) - This session discusses color consistency and provides code examples related to color processing.
- Use HDR for dynamic image experiences in your app (WWDC 2024) - This session covers HDR image processing, which may include color conversion techniques.
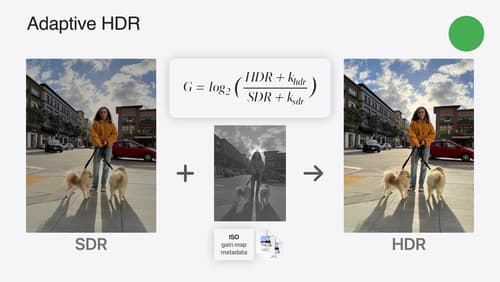
Use HDR for dynamic image experiences in your app
Discover how to read and write HDR images and process HDR content in your app. Explore the new supported HDR image formats and advanced methods for displaying HDR images. Find out how HDR content can coexist with your user interface — and what to watch out for when adding HDR image support to your app.
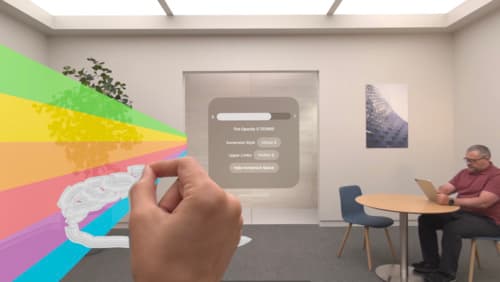
Render Metal with passthrough in visionOS
Get ready to extend your Metal experiences for visionOS. Learn best practices for integrating your rendered content with people’s physical environments with passthrough. Find out how to position rendered content to match the physical world, reduce latency with trackable anchor prediction, and more.
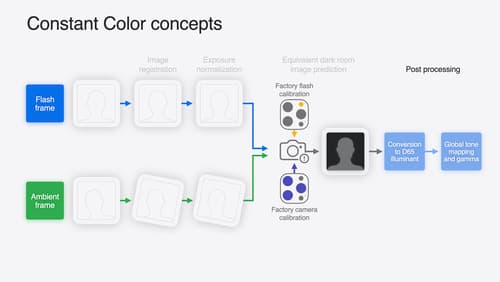
Keep colors consistent across captures
Meet the Constant Color API and find out how it can help people use your app to determine precise colors. You’ll learn how to adopt the API, explore its scientific and marketing potential, and discover best practices for making the most of the technology.