async stream
Generated on 8/5/2024
1 search
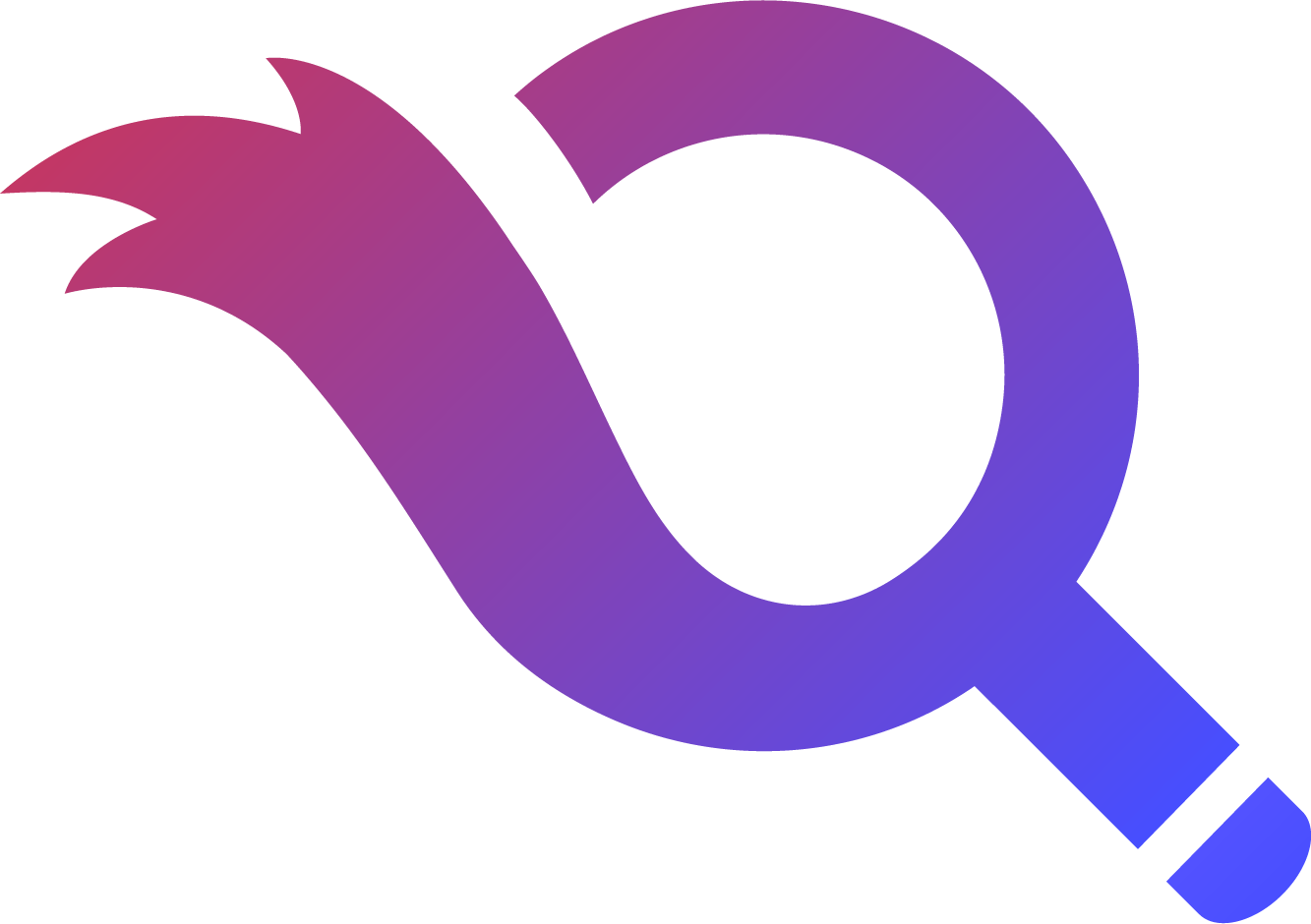
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
Async Stream in Swift
In Swift, asynchronous functions and streams are implemented using the async
and await
keywords. Here are some key points about how async functions work in Swift:
-
Async Functions and Suspension Points:
- Async functions are split into multiple partial functions at runtime, each spanning the gaps between potential suspension points (e.g.,
await
calls). - When an async function is called, it may suspend at an
await
point, yielding the CPU to other tasks. This is crucial for efficient concurrency.
- Async functions are split into multiple partial functions at runtime, each spanning the gaps between potential suspension points (e.g.,
-
Memory Allocation for Async Functions:
- Async functions do not allocate memory on the C stack. Instead, they use a separate stack, allocating memory in slabs.
- When an async function needs memory, it requests it from the current slab. If the slab is full, a new slab is allocated using
malloc
.
-
Partial Functions:
- Each async function is divided into partial functions. For example, if there is one
await
in the function, it results in two partial functions. - The first partial function runs until the
await
point, and the second one resumes after theawait
.
- Each async function is divided into partial functions. For example, if there is one
-
Concurrency and Task Suspension:
- Swift uses the
async
keyword to mark functions that may suspend, and theawait
keyword to indicate suspension points. - This allows the CPU to be used efficiently by other tasks while waiting for asynchronous operations to complete.
- Swift uses the
For a detailed explanation of async functions and their implementation, you can refer to the session Explore Swift performance.
Relevant Sessions
- Explore Swift performance
- A Swift Tour: Explore Swift’s features and design
- Explore the Swift on Server ecosystem
- Go further with Swift Testing
These sessions cover various aspects of async functions, concurrency, and their applications in Swift.
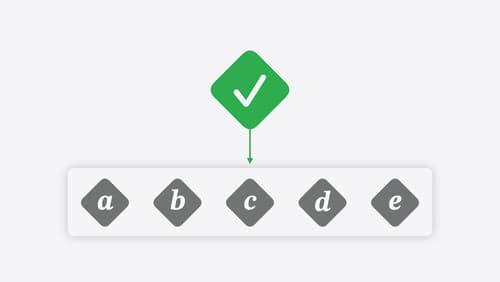
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
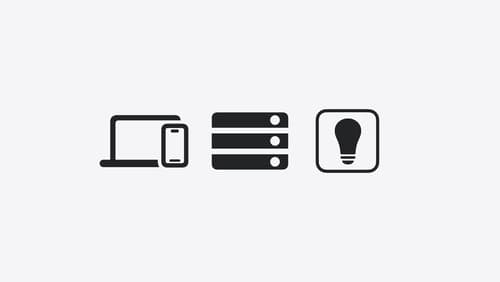
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
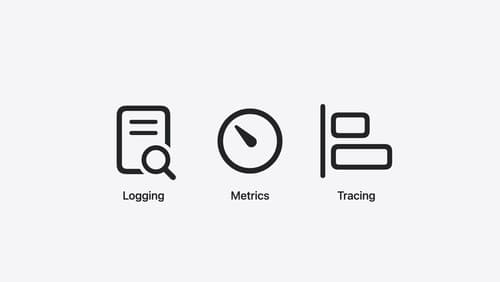
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.
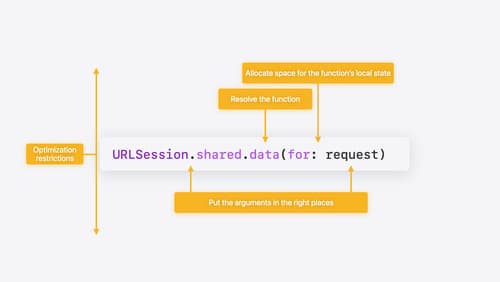
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.