asyncstream
Generated on 8/5/2024
3 searches
AsyncStream in Swift
AsyncStream is a powerful feature in Swift that allows for handling asynchronous sequences of values. It is particularly useful when dealing with streams of data that are produced over time, such as network responses, user input events, or any other kind of asynchronous data source.
Key Concepts
-
Async Functions:
- Async functions in Swift are designed to handle operations that can suspend execution to wait for a result, such as network requests or file I/O.
- These functions use the
async
keyword and are called with theawait
keyword to indicate potential suspension points.
-
Memory Management:
- Async functions manage their local state on a separate stack from the C stack.
- They are split into multiple partial functions at runtime to handle suspension points efficiently.
-
Concurrency:
- Swift's concurrency model uses
async
andawait
to manage asynchronous operations. - Actors are used to encapsulate shared mutable state and ensure safe concurrent access.
- Swift's concurrency model uses
Relevant Sessions
-
- This session discusses how async functions are implemented, including their memory management and how they are split into partial functions to handle suspension points.
-
A Swift Tour: Explore Swift’s features and design:
- This session covers the basics of writing concurrent code in Swift, including tasks,
async
/await
, and actors.
- This session covers the basics of writing concurrent code in Swift, including tasks,
-
- This session introduces improvements in Swift 6, including complete data race safety and new low-level synchronization primitives.
Example Usage
Here is a simple example of using AsyncStream
in Swift:
import Foundation
func fetchData() async -> AsyncStream<String> {
AsyncStream { continuation in
// Simulate asynchronous data fetching
DispatchQueue.global().asyncAfter(deadline: .now() + 1) {
continuation.yield("Data 1")
}
DispatchQueue.global().asyncAfter(deadline: .now() + 2) {
continuation.yield("Data 2")
}
DispatchQueue.global().asyncAfter(deadline: .now() + 3) {
continuation.finish()
}
}
}
Task {
for await data in fetchData() {
print(data)
}
}
In this example, fetchData
returns an AsyncStream
that yields data asynchronously. The Task
block consumes this stream, printing each piece of data as it arrives.
Conclusion
AsyncStream and the related concurrency features in Swift provide a robust framework for handling asynchronous operations efficiently and safely. For more detailed information, you can refer to the sessions mentioned above from WWDC 2024.
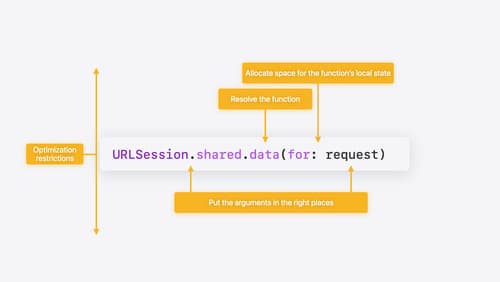
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
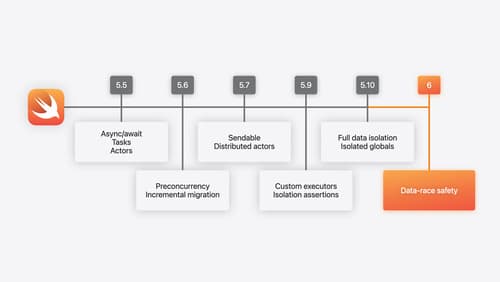
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
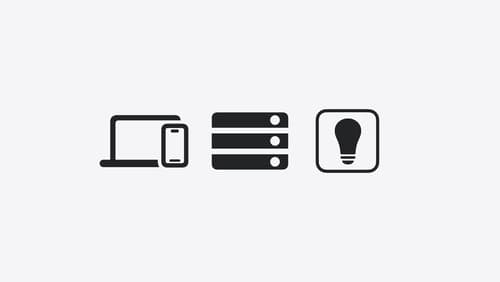
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.