how do I search using CoreData
Generated on 8/5/2024
1 search
To search using CoreData, you can leverage the new features introduced in iOS 18, which include the ability to build complex predicates using Foundation's new expression macro. Here’s a brief overview of how you can implement search functionality:
-
Add a Search Bar: Start by adding a search bar to your app's user interface. This will allow users to input their search queries.
-
Build a Predicate: Use the search text from the search bar to build a predicate. A predicate is a logical condition that can be used to filter data. For example, you can create a predicate to check if a trip's name contains the search text.
-
Compound Predicate: If the search text might apply to more than one property (e.g., trip's name and destination), you can build a compound predicate to check multiple properties.
-
Expression Macro: New in iOS 18, the expression macro allows you to build complex predicates easily. Expressions can represent complex evaluations using a model's properties and can be composed within predicates to tailor the results of queries even further.
Here is a simple example of how you might implement this in code:
import CoreData
// Assuming you have a search bar and a managed object context
let searchText = "search query"
let context: NSManagedObjectContext = ...
// Create a predicate to filter trips by name or destination
let namePredicate = NSPredicate(format: "name CONTAINS[cd] %@", searchText)
let destinationPredicate = NSPredicate(format: "destination CONTAINS[cd] %@", searchText)
let compoundPredicate = NSCompoundPredicate(orPredicateWithSubpredicates: [namePredicate, destinationPredicate])
// Fetch request with the compound predicate
let fetchRequest: NSFetchRequest<Trip> = Trip.fetchRequest()
fetchRequest.predicate = compoundPredicate
do {
let results = try context.fetch(fetchRequest)
// Handle the results
} catch {
// Handle the error
}
For more detailed information, you can refer to the session What’s new in SwiftData (09:47).
Additionally, if you are interested in integrating search functionality with Spotlight, you can look into the session Support semantic search with Core Spotlight (00:51) which covers how to build a full search experience in your app using Core Spotlight.
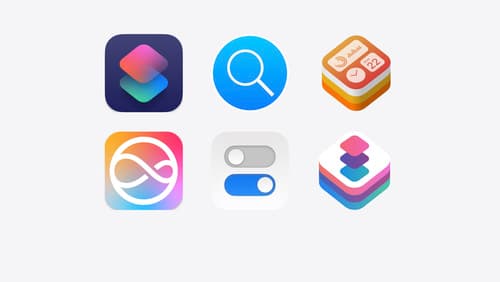
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.
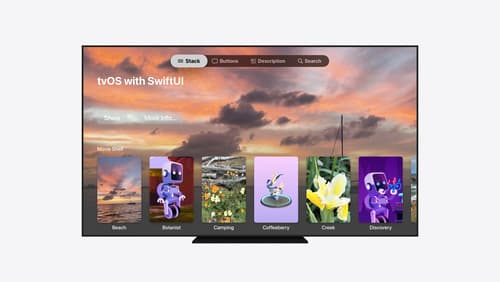
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
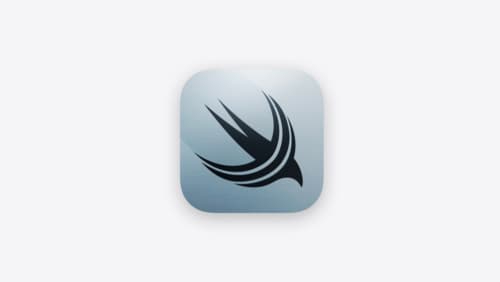
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.
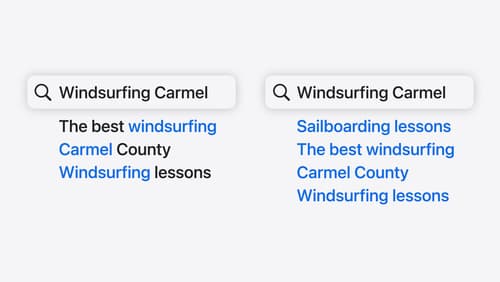
Support semantic search with Core Spotlight
Learn how to provide semantic search results in your app using Core Spotlight. Understand how to make your app’s content available in the user’s private, on-device index so people can search for items using natural language. We’ll also share how to optimize your app’s performance by scheduling indexing activities. To get the most out of this session, we recommend first checking out Core Spotlight documentation on the Apple Developer website.
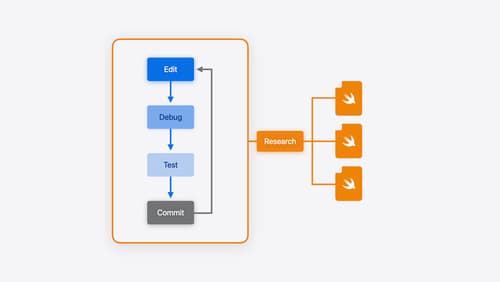
Xcode essentials
Edit, debug, commit, repeat. Explore the suite of tools in Xcode that help you iterate quickly when developing apps. Discover tips and tricks to help optimize and boost your development workflow.