What's a good way to handle asynchronous code
Generated on 8/5/2024
1 search
Handling asynchronous code in Swift can be effectively managed using the async
and await
keywords. Here are some key points and techniques discussed in various WWDC sessions:
-
Async/Await Syntax:
- Swift uses the
async
keyword to mark functions that may suspend, and theawait
keyword to indicate suspension points within those functions. This allows the CPU to yield to other tasks while waiting for an asynchronous operation to complete. - Example:
func fetchData() async throws -> Data { let data = try await someAsyncFunction() return data }
- For more details, you can refer to the session A Swift Tour: Explore Swift’s features and design.
- Swift uses the
-
Handling Completion Handlers:
- If you have code that uses completion handlers, Swift provides an
async
overload automatically. If anasync
overload is not available, you can usewithCheckedContinuation
orwithCheckedThrowingContinuation
to convert it to an expression that can be awaited. - Example:
func fetchData() async throws -> Data { return try await withCheckedThrowingContinuation { continuation in someFunctionWithCompletionHandler { result, error in if let error = error { continuation.resume(throwing: error) } else { continuation.resume(returning: result) } } } }
- For more information, see the session Go further with Swift Testing.
- If you have code that uses completion handlers, Swift provides an
-
Concurrency and Data-Race Safety:
- Swift 6 introduces complete data-race protection, ensuring that concurrent code is safe and reliable. This includes using actors to manage state and ensuring that access to shared mutable state is properly synchronized.
- Example:
actor UserStore { var users: [User] = [] func addUser(_ user: User) { users.append(user) } }
- For more details, refer to the session Migrate your app to Swift 6.
-
Testing Asynchronous Code:
- When writing tests for asynchronous code, you can use the same concurrency features as in your production code. The
await
keyword will suspend the test, allowing other test code to execute while waiting for the asynchronous operation to complete. - Example:
func testAsyncFunction() async throws { let result = try await someAsyncFunction() XCTAssertEqual(result, expectedValue) }
- For more information, see the session Go further with Swift Testing.
- When writing tests for asynchronous code, you can use the same concurrency features as in your production code. The
By leveraging these techniques, you can handle asynchronous code in Swift more effectively, ensuring better performance and reliability in your applications.
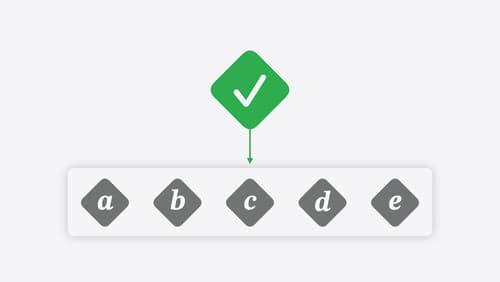
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
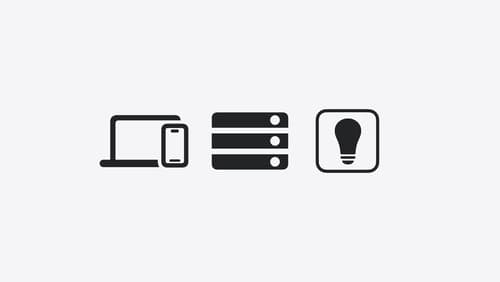
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
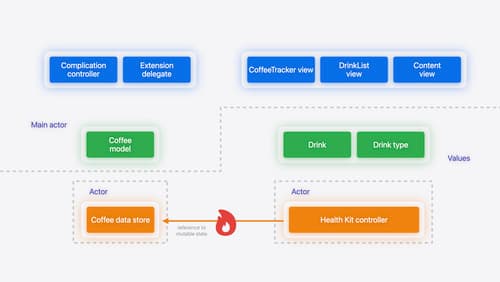
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
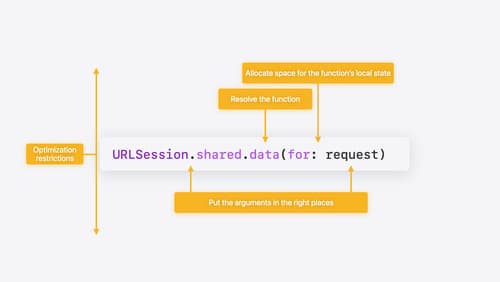
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.