how do I use background fetch?
Generated on 8/5/2024
1 search
To use background fetch in your app, you can take advantage of the performFetchWithCompletionHandler
method provided by the UIApplicationDelegate
protocol. This method allows your app to fetch data in the background and update its content even when the app is not actively running.
Here are the steps to implement background fetch:
-
Enable Background Fetch:
- Go to your app's target settings in Xcode.
- Select the "Capabilities" tab.
- Turn on "Background Modes" and check "Background fetch".
-
Implement
performFetchWithCompletionHandler
:- In your app delegate, implement the
application(_:performFetchWithCompletionHandler:)
method. - This method will be called by the system at appropriate times to allow your app to fetch new data.
- In your app delegate, implement the
func application(_ application: UIApplication, performFetchWithCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
// Fetch your data here
fetchData { newData in
if newData {
completionHandler(.newData)
} else {
completionHandler(.noData)
}
}
}
- Schedule Background Fetch:
- You can use
setMinimumBackgroundFetchInterval
to set how frequently your app should fetch data.
- You can use
application.setMinimumBackgroundFetchInterval(UIApplication.backgroundFetchIntervalMinimum)
- Fetch Data:
- Implement your data fetching logic in a separate method and call it from
performFetchWithCompletionHandler
.
- Implement your data fetching logic in a separate method and call it from
func fetchData(completion: @escaping (Bool) -> Void) {
// Your data fetching logic here
// Call completion(true) if new data is fetched, otherwise call completion(false)
}
For more detailed information on background fetch and other related topics, you might find the session Extend your app’s controls across the system useful, especially the chapter on "Update toggle states" which starts at 399 seconds. This session covers various aspects of updating app states and controls, which can be relevant when dealing with background fetch scenarios.
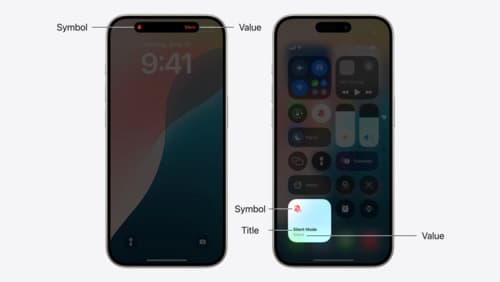
Extend your app’s controls across the system
Bring your app’s controls to Control Center, the Lock Screen, and beyond. Learn how you can use WidgetKit to extend your app’s controls to the system experience. We’ll cover how you can to build a control, tailor its appearance, and make it configurable.
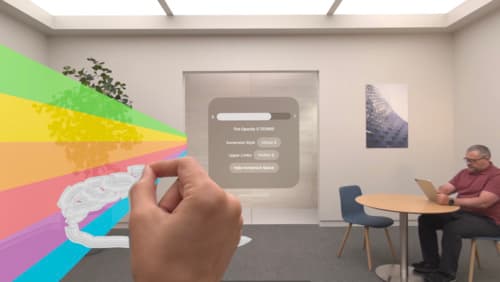
Render Metal with passthrough in visionOS
Get ready to extend your Metal experiences for visionOS. Learn best practices for integrating your rendered content with people’s physical environments with passthrough. Find out how to position rendered content to match the physical world, reduce latency with trackable anchor prediction, and more.
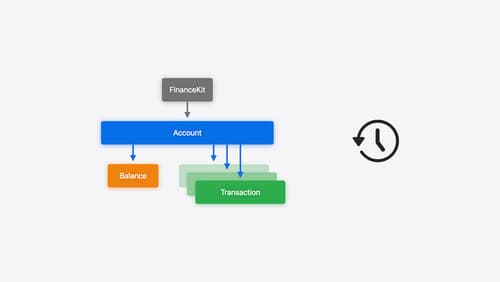
Meet FinanceKit
Learn how FinanceKit lets your financial management apps seamlessly and securely share on-device data from Apple Cash, Apple Card, and more, with user consent and control. Find out how to request one-time and ongoing access to accounts, transactions, and balances — and how to build great experiences for iOS and iPadOS.
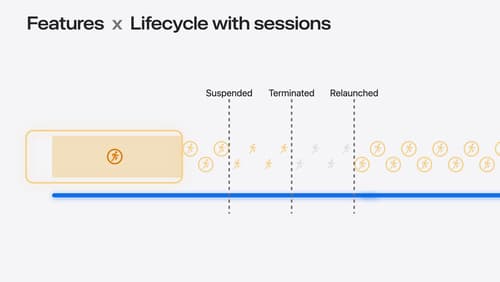
What’s new in location authorization
Location authorization is turning 2.0. Learn about new recommendations and techniques to get the authorization you need, and a new system of diagnostics that can let you know when an authorization goal can’t be met.
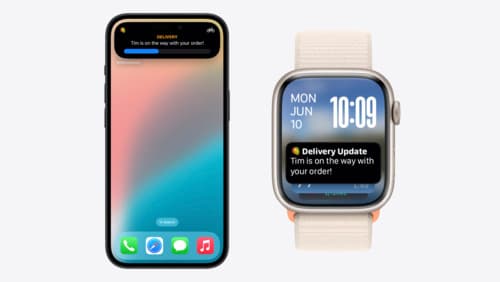
Bring your Live Activity to Apple Watch
Bring Live Activities into the Smart Stack on Apple Watch with iOS 18 and watchOS 11. We’ll cover how Live Activities are presented on Apple Watch, as well as how you can enhance their presentation for the Smart Stack. We’ll also explore additional considerations to ensure Live Activities on Apple Watch always present up-to-date information.
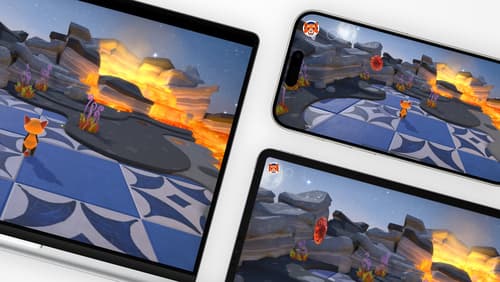
Design advanced games for Apple platforms
Learn how to adapt your high-end game so it feels at home on Mac, iPad, and iPhone. We’ll go over how to make your game look stunning on different displays, tailor your input and controls to be intuitive on each device, and take advantage of Apple technologies that deliver great player experiences.
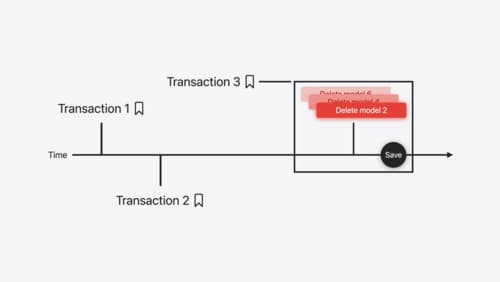
Track model changes with SwiftData history
Reveal the history of your model’s changes with SwiftData! Use the history API to understand when data store changes occurred, and learn how to use this information to build features like remote server sync and out-of-process change handing in your app. We’ll also cover how you can build support for the history API into a custom data store.
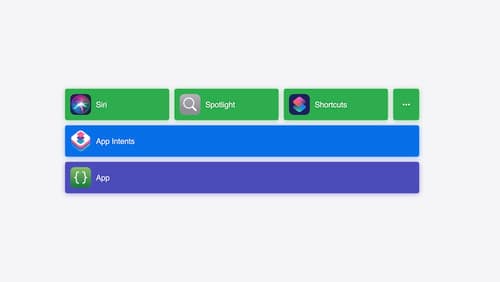
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.