how do I call a web api and show the results in a table view
Generated on 8/6/2024
1 search
To call a web API and show the results in a table view, you can follow these steps:
- Fetch Data from the Web API: Use URLSession to make an asynchronous request to the web API.
- Parse the JSON Response: Decode the JSON response into Swift model objects.
- Update the UI: Use SwiftUI to display the data in a table view.
Here’s a basic example to illustrate these steps:
Step 1: Fetch Data from the Web API
import Foundation
struct APIResponse: Codable {
let results: [Result]
}
struct Result: Codable, Identifiable {
let id: Int
let name: String
}
func fetchData(completion: @escaping ([Result]) -> Void) {
guard let url = URL(string: "https://api.example.com/data") else { return }
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
do {
let decodedResponse = try JSONDecoder().decode(APIResponse.self, from: data)
DispatchQueue.main.async {
completion(decodedResponse.results)
}
} catch {
print("Error decoding data: \(error)")
}
}
}.resume()
}
Step 2: Parse the JSON Response
The fetchData
function above decodes the JSON response into an array of Result
objects.
Step 3: Update the UI
Use SwiftUI to display the data in a table view.
import SwiftUI
struct ContentView: View {
@State private var results = [Result]()
var body: some View {
NavigationView {
List(results) { result in
Text(result.name)
}
.navigationTitle("Results")
.onAppear {
fetchData { fetchedResults in
self.results = fetchedResults
}
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Example from WWDC Sessions
For more detailed examples, you can refer to the following sessions from WWDC:
- Migrate your TVML app to SwiftUI: This session discusses how to use SwiftUI to build interfaces, including how to handle search and display results in a grid layout.
- Bring context to today’s weather: This session provides an example of fetching data from a web API using WeatherKit and displaying it.
These sessions provide practical examples and best practices for fetching data from web APIs and displaying it in your app using SwiftUI.
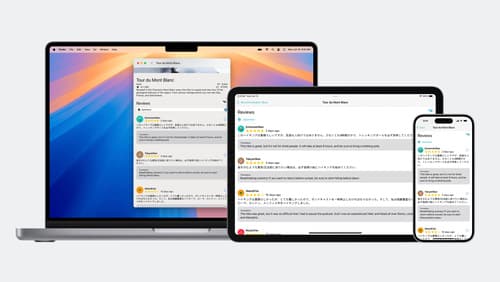
Meet the Translation API
Discover how you can translate text across different languages in your app using the new Translation framework. We’ll show you how to quickly display translations in the system UI, and how to translate larger batches of text for your app’s UI.
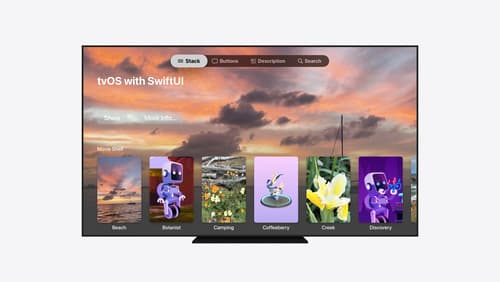
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
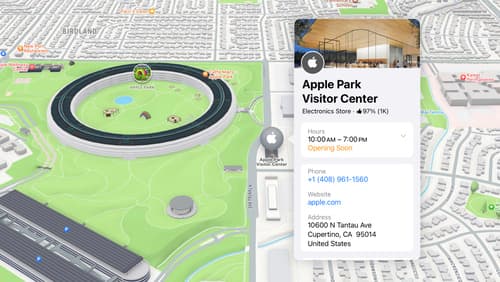
Unlock the power of places with MapKit
Discover powerful new ways to integrate maps into your apps and websites with MapKit and MapKit JS. Learn how to save and reference unique places using Place ID. Check out improvements to search that make it more efficient to find relevant places. Get introduced to the new Place Card API that lets you display rich information about places so customers can explore destinations right in your app. And, we’ll show you quick ways to embed maps in your website with our simplified token provisioning and Web Embed API.
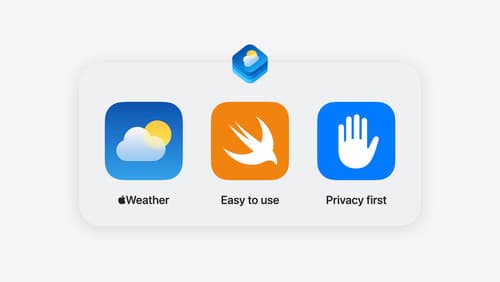
Bring context to today’s weather
Harness the power of WeatherKit to get detailed weather forecast data such as precipitation amounts by type, cloud cover by altitude, or maximum wind speed. Find out how you can summarize weather by different parts of the day and highlight significant upcoming changes to temperature or precipitation. Understand how you can compare current weather to the past through our Historical Comparisons dataset and dive into historical weather statistics for any location in the world. We’ll also explore how you can do all of this faster with our Swift and REST APIs.