How can I call async code (written with Swift concurrency and async/await) from synchronous code
Generated on 8/6/2024
1 search
To call async code (written with Swift concurrency and async/await) from synchronous code, you can use the Task
API to bridge between synchronous and asynchronous contexts. Here’s a basic example of how you can achieve this:
func synchronousFunction() {
let semaphore = DispatchSemaphore(value: 0)
Task {
await asyncFunction()
semaphore.signal()
}
semaphore.wait()
}
func asyncFunction() async {
// Your async code here
}
In this example, synchronousFunction
is a synchronous function that calls the asynchronous asyncFunction
. It uses a DispatchSemaphore
to wait for the asynchronous task to complete before continuing.
For more details on Swift concurrency and async/await, you can refer to the session A Swift Tour: Explore Swift’s features and design at WWDC 2024, which covers the basics of writing concurrent code in Swift, including tasks, async/await, and actors.
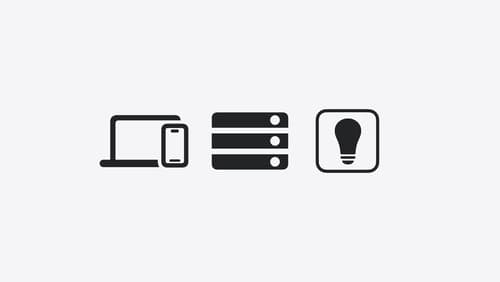
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
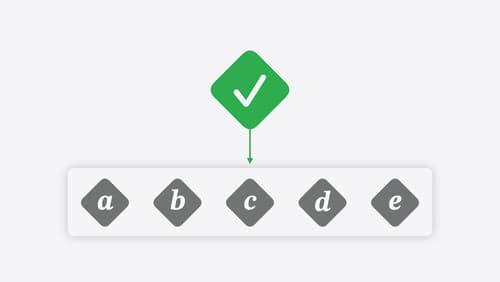
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
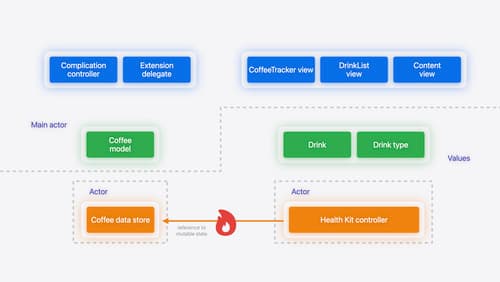
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.