recording video with multiple cameras
Asked on 08/06/2024
1 search
To record video with multiple cameras, you can utilize the AV Foundation framework, which provides a robust set of APIs for capturing and processing video. Here's a high-level overview of how you can achieve this, particularly focusing on spatial video recording as discussed in the session "Build compelling spatial photo and video experiences" at WWDC 2024.
Steps to Record Video with Multiple Cameras
-
Create an AV Capture Session:
- Initialize an
AVCaptureSession
to manage the data flow between the input (camera) and output (movie file).
- Initialize an
-
Set Up the Capture Device:
- Use the built-in dual wide camera, which includes both the wide and ultra-wide cameras. This is essential for spatial video recording.
- Example code snippet:
let captureSession = AVCaptureSession() guard let captureDevice = AVCaptureDevice.default(.builtInDualWideCamera, for: .video, position: .back) else { fatalError("No dual wide camera available") }
-
Add Input to the Session:
- Create an
AVCaptureDeviceInput
with the capture device and add it to the session. - Example code snippet:
do { let input = try AVCaptureDeviceInput(device: captureDevice) captureSession.addInput(input) } catch { print("Error adding input: \(error)") }
- Create an
-
Add Output to the Session:
- Create an
AVCaptureMovieFileOutput
to manage the output and write it to disk. - Example code snippet:
let movieOutput = AVCaptureMovieFileOutput() captureSession.addOutput(movieOutput)
- Create an
-
Enable Spatial Video Recording:
- Check if spatial video capture is supported and enable it.
- Example code snippet:
if captureDevice.isSpatialVideoCaptureSupported { captureDevice.isSpatialVideoCaptureEnabled = true } else { print("Spatial video capture not supported on this device") }
-
Start the Capture Session:
- Commit the configuration and start running the session.
- Example code snippet:
captureSession.commitConfiguration() captureSession.startRunning()
Additional Enhancements
-
Video Stabilization:
- Set the preferred video stabilization mode to
cinematicExtendedEnhanced
for smoother videos. - Example code snippet:
if let connection = movieOutput.connection(with: .video) { connection.preferredVideoStabilizationMode = .cinematicExtendedEnhanced }
- Set the preferred video stabilization mode to
-
Video Preview:
- Use
AVCaptureVideoPreviewLayer
to get a video preview feed. - Example code snippet:
let previewLayer = AVCaptureVideoPreviewLayer(session: captureSession) previewLayer.videoGravity = .resizeAspectFill
- Use
For more detailed information and code examples, you can refer to the session "Build compelling spatial photo and video experiences" at WWDC 2024.
Relevant Sessions
These sessions provide comprehensive insights into recording and handling video with multiple cameras, including spatial video and multiview playback.
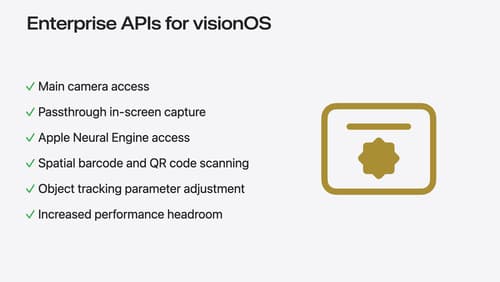
Introducing enterprise APIs for visionOS
Find out how you can use new enterprise APIs for visionOS to create spatial experiences that enhance employee and customer productivity on Apple Vision Pro.
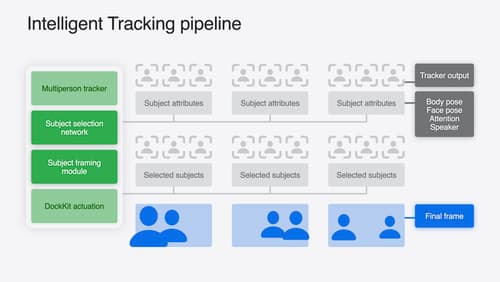
What’s new in DockKit
Discover how intelligent tracking in DockKit allows for smoother transitions between subjects. We will cover what intelligent tracking is, how it uses an ML model to select and track subjects, and how you can use it in your app.
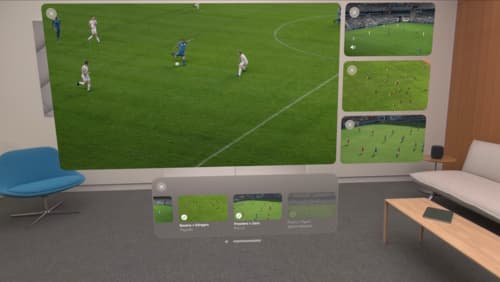
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.