How to make purchase using storekit 2
Generated on 8/6/2024
1 search
To make a purchase using StoreKit 2, you can utilize the Swift async/await
pattern to perform tasks such as fetching product metadata, purchasing, and retrieving your customer transaction history. Here is a brief overview of the steps involved:
-
Fetch Product Metadata: Use the
Product.products(for:)
method to fetch the product metadata for the items you want to offer in your app. -
Initiate a Purchase: Use the
Product.purchase()
method to initiate a purchase. This method will handle the purchase flow and return aTransaction
object upon completion. -
Handle Transactions: Use the
Transaction.updates
property to listen for transaction updates and handle them accordingly.
Here is a simple example in Swift:
import StoreKit
func fetchProducts() async throws -> [Product] {
let productIDs = ["com.example.app.product1", "com.example.app.product2"]
let products = try await Product.products(for: productIDs)
return products
}
func purchaseProduct(_ product: Product) async throws -> Transaction {
let result = try await product.purchase()
switch result {
case .success(let verification):
let transaction = try verification.payloadValue
// Handle successful purchase
return transaction
case .userCancelled, .pending:
// Handle user cancellation or pending state
throw PurchaseError.userCancelled
@unknown default:
// Handle unknown cases
throw PurchaseError.unknown
}
}
func listenForTransactions() {
Task {
for await result in Transaction.updates {
switch result {
case .verified(let transaction):
// Handle verified transaction
await transaction.finish()
case .unverified(_, _):
// Handle unverified transaction
}
}
}
}
For more detailed information, you can refer to the session What’s new in StoreKit and In-App Purchase from WWDC 2024. This session covers the new features in StoreKit 2 and provides a comprehensive guide on how to implement in-app purchases using the new APIs.
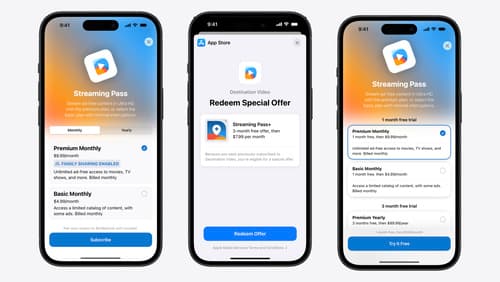
What’s new in StoreKit and In-App Purchase
Learn how to build and deliver even better purchase experiences using the App Store In-App Purchase system. We’ll demo new StoreKit views control styles and new APIs to improve your subscription customization, discuss new fields for transaction-level information, and explore new testability in Xcode. We’ll also review an important StoreKit deprecation.
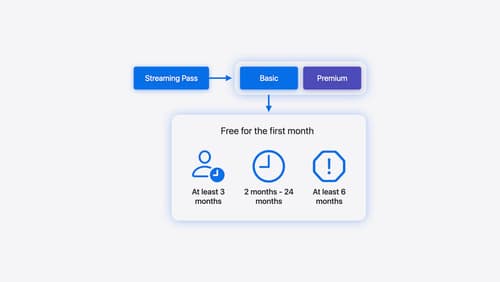
Implement App Store Offers
Learn how to engage customers with App Store Offers using App Store Connect, as well as the latest StoreKit features and APIs. Discover how you can set up win-back offers (a new way to re-engage previous subscribers) and generate offer codes for Mac apps. And find out how to test offers in sandbox and Xcode to make sure they work smoothly.