how to write shader
Generated on 8/6/2024
1 search
To write shaders for custom visual effects in SwiftUI, you need to use the Metal shading language. Here are the steps to get started:
-
Instantiate a Shader in SwiftUI:
- You can instantiate a shader in SwiftUI by calling a function with its name on the shader library. You can also pass additional parameters to your shader function, such as colors, numbers, or images.
- Example:
let myShader = ShaderLibrary.myShaderFunction(color: .red, number: 5)
-
Apply the Shader to a View:
- Use the
layerEffect
view modifier to apply the shader to a view. SwiftUI will call your shader function for every pixel of your view. - Example:
myView.layerEffect(myShader)
- Use the
-
Write the Shader in Metal:
- Shaders are written in the Metal shading language, not Swift. The shader function name in the Metal file should match the invocation in the shader library.
- Example Metal shader function:
fragment half4 myShaderFunction(VertexOut in [[stage_in]], constant float4 &color [[buffer(0)]]) { // Shader code here return half4(color); }
-
Understand the Arguments:
- The
position
argument refers to the pixel's location, and thelayer
argument represents your view's content. You can sample the layer to obtain its contents. - Example:
fragment half4 myShaderFunction(VertexOut in [[stage_in]], constant float4 &color [[buffer(0)]]) { float2 pos = in.position.xy; // Sample the layer content half4 layerColor = layer.sample(pos); return layerColor * half4(color); }
- The
For more detailed information, you can refer to the session Create custom visual effects with SwiftUI (20:10).
Relevant Sessions
These sessions provide comprehensive insights into writing and optimizing shaders for both SwiftUI and advanced game development on Apple platforms.
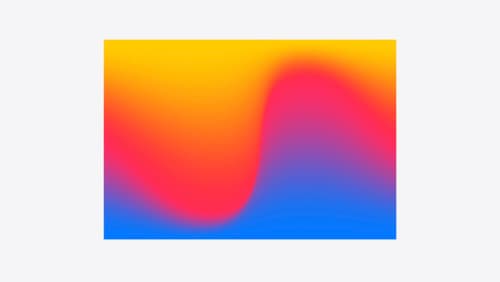
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
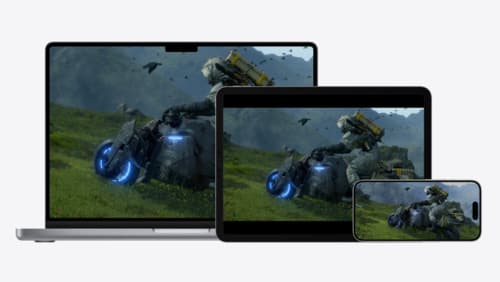
Port advanced games to Apple platforms
Discover how simple it can be to reach players on Apple platforms worldwide. We’ll show you how to evaluate your Windows executable on Apple silicon, start your game port with code samples, convert your shader code to Metal, and bring your game to Mac, iPhone, and iPad. Explore enhanced Metal tools that understand HLSL shaders to validate, debug, and profile your ported shaders on Metal.