How to embed few independent table views into one scroll view?
Generated on 8/6/2024
1 search
To embed a few independent table views into one scroll view using SwiftUI, you can leverage the flexibility of SwiftUI's container views and composition capabilities. Here are the steps you can follow:
-
Create Individual Table Views: Define each of your table views as separate SwiftUI views. You can use
List
orForEach
to create these table views. -
Embed in a Scroll View: Use a
ScrollView
to contain all your individual table views. This allows you to scroll through the combined content. -
Use VStack or HStack: Depending on your layout preference (vertical or horizontal), you can use a
VStack
orHStack
to stack your table views inside theScrollView
.
Here is a basic example in SwiftUI:
import SwiftUI
struct ContentView: View {
var body: some View {
ScrollView {
VStack {
TableView1()
TableView2()
TableView3()
}
}
}
}
struct TableView1: View {
var body: some View {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
}
}
}
struct TableView2: View {
var body: some View {
List {
Text("Item A")
Text("Item B")
Text("Item C")
}
}
}
struct TableView3: View {
var body: some View {
List {
Text("Item X")
Text("Item Y")
Text("Item Z")
}
}
}
Relevant Sessions
- Demystify SwiftUI containers discusses how to use
ForEach
and other container views to manage and compose complex layouts. - SwiftUI essentials covers the fundamentals of views and how to create custom layouts.
By following these steps and referring to the relevant sessions, you should be able to effectively embed multiple independent table views into a single scroll view in your SwiftUI application.
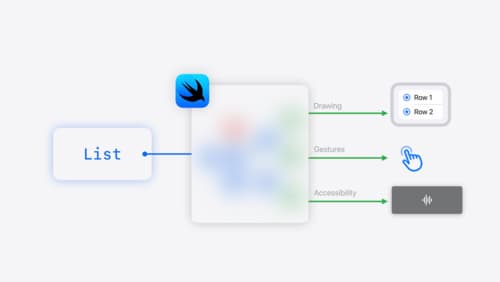
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
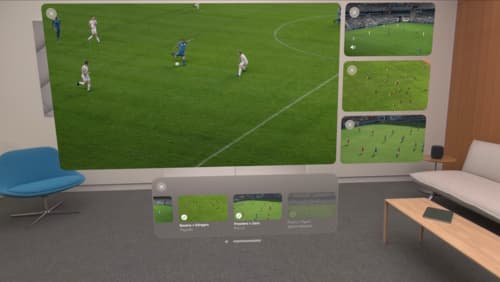
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.
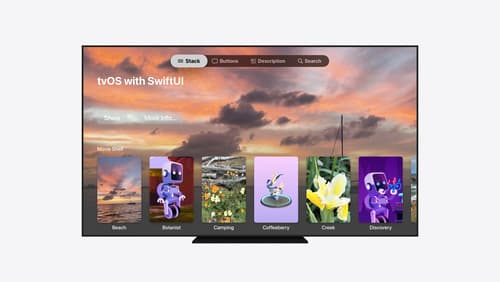
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
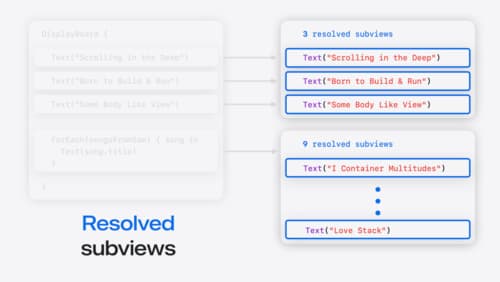
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.